How to Overload a Constructor in Java
- Default Constructor in Java
- Parameterized Constructor in Java
- Code Without Constructor Overloading
- Constructor Overloading in Java
- Constructor Overloading Best Practices
- Overload a Constructor
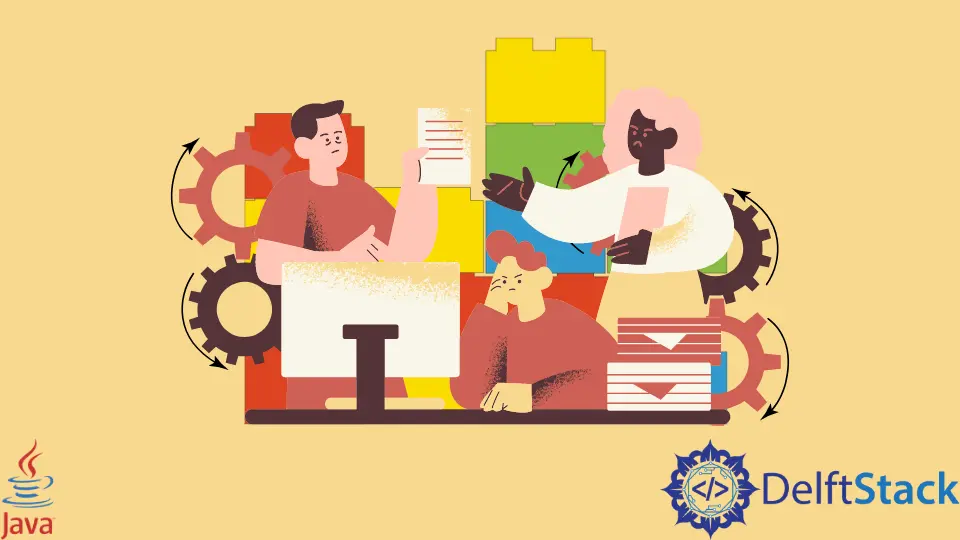
This tutorial introduces how to overload constructors in Java. We’ve also listed some example codes you can follow to understand this topic better.
A constructor is a method called to allocate memory for a class object and initialize the class attributes for that object. If no constructor has been created for a class, then Java provides a default constructor.
For example, no constructor has been defined in the class below. Still, we can create an object of that class while the attributes are initialized to their default values(null, in this case).
Default Constructor in Java
class Student {
String name;
Double gpa;
}
public class Main {
public static void main(String[] args) {
Student s = new Student();
System.out.println(s.name + "\t" + s.gpa);
}
}
Output:
null null
The constructor overloading concept is similar to method overloading, which means that we have more than one constructor for a single class. Constructor overloading is done to initialize the member variables of the class in different ways.
We can create as many overloaded constructors as we want. The only condition is that the overloaded constructors should differ in the number and the type of parameters that they take.
For example, consider the Student
class shown below with two attributes: student name
and GPA
. A constructor is defined for the class, which takes a string name and a double GPA
as parameters and initializes the corresponding attributes for the new object.
Parameterized Constructor in Java
class Student {
String name;
Double gpa;
Student(String s, Double g) {
name = s;
gpa = g;
}
}
public class Main {
public static void main(String[] args) {
Student s = new Student("Justin", 9.75);
System.out.println(s.name + "\t" + s.gpa);
}
}
Output:
Justin 9.75
Code Without Constructor Overloading
Consider a scenario where only the student name is passed to the constructor when creating a new object; the GPA
should be automatically set to null
in this case. If we do not overload the constructor and just pass the name, we get the following compilation error.
class Student {
String name;
Double gpa;
Student(String s, Double g) {
name = s;
gpa = g;
}
}
public class Main {
public static void main(String[] args) {
Student s = new Student("Justin");
System.out.println(s.name + "\t" + s.gpa);
}
}
Output:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The constructor Student(String) is undefined
at ConstructorOverloading.main(ConstructorOverloading.java:18)
Constructor Overloading in Java
We can create an overloaded constructor with just the student name as the parameter to tackle the situation above. We will set the GPA
to null
when this constructor is called.
class Student {
String name;
Double gpa;
Student(String s, Double g) {
name = s;
this.gpa = g;
}
Student(String s) {
name = s;
gpa = null; // Setting GPA to null
}
}
public class Main {
public static void main(String[] args) {
Student s1 = new Student("Justin");
Student s2 = new Student("Jessica", 9.23);
System.out.println(s1.name + "\t" + s1.gpa);
System.out.println(s2.name + "\t" + s2.gpa);
}
}
Output:
Justin null
Jessica 9.23
Consider another situation where a Student
object is created, but neither the name nor the GPA
has been mentioned. We can make another overloaded constructor that takes no arguments(default constructor) and sets the name
and GPA
attributes to null
.
Remember that Java provides a default constructor only when no other constructor is created for a class. But for our class, constructors already exist, and so we need to create a default constructor.
class Student {
String name;
Double gpa;
Student(String s, Double g) {
name = s;
gpa = g;
}
Student(String s) {
name = s;
gpa = null;
}
Student() {
name = null;
gpa = null;
}
}
public class Main {
public static void main(String[] args) {
Student s1 = new Student();
Student s2 = new Student("Justin");
Student s3 = new Student("Jessica", 9.23);
System.out.println(s1.name + "\t" + s1.gpa);
System.out.println(s2.name + "\t" + s2.gpa);
System.out.println(s3.name + "\t" + s3.gpa);
}
}
Output:
null null
Justin null
Jessica 9.23
Constructor Overloading Best Practices
There are no set guidelines to follow when overloading constructors. However, there are some recommended best practices that we can follow to avoid confusion and reduce the scope of error.
- One should use the
this
keyword to reference the member variables of the class. It avoids confusion and makes our code more readable. - We can also pass more intuitive parameter names to the constructor. For example, if a constructor initializes the name field, then the constructor signature could be
Student(String name)
instead ofStudent(String s)
. Using thethis
keyword, we can differentiate between the parameter, calledname
, and the class attribute, also calledname
.
Let’s carry on with the example of the Student
class and use the this
keyword inside the constructors.
class Student {
String name;
Double gpa;
Student(String name, Double gpa) // passing more intuitive parameter names
{
this.name = name; // using this keyword to avoid confusion
this.gpa = gpa;
}
Student(String name) {
this.name = name;
this.gpa = null;
Student()
this.name = null;
this.gpa = null;
}
}
- It is also a good practice for a class to only have a
single primary constructor
, and all the remaining constructors should call this primary constructor to initialize the attributes. - We can call a constructor from another constructor by using the
this()
function. The number and types of attributes that we pass tothis()
will decide which constructor will be called. - It will reduce code redundancy, and we only need to write the logic for a single constructor.
- In the
Student
class, we need three overloaded constructors. The primary constructor will be the parametrized one that takes bothname
andGPA
as parameters. The other two overloaded constructors will simply call the primary constructor and pass the values of the parameters asnull
.
class Student {
String name;
Double gpa;
Student(String name, Double gpa) // Primary Constructor that sets the attribute values
{
this.name = name;
this.gpa = gpa;
}
Student(String name) {
this(name, null); // Calling the primary constructor with GPA as null
}
Student() {
this(null, null); // Calling the primary constructor with both parameters as null
}
}
Overload a Constructor
Overloading constructors is done to initialize the attributes of a class in different ways. Overloaded constructors should differ in the number of parameters, or the data type of the parameters passed to them.
The signature of each constructor should be different from the others. We should use the this
keyword to reference the class attributes and other constructors as it makes our code less redundant and easier to understand.