The Default Constructor in Java
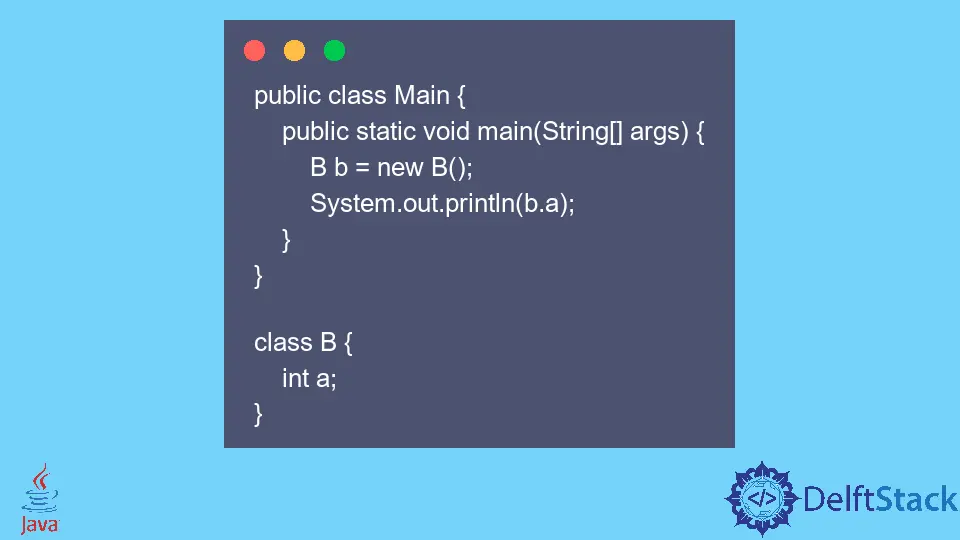
In the Java language, the term constructor
is similar to the methods we run in programming. There are a few properties of a constructor that you should keep in mind during its creation.
- The name of the constructor must always be the same as that of the class name.
- There must not be any return type of the constructor.
- There can be parameterized constructor and
no-argument
constructor (default constructor) in a class. - The constructor always gets invoked when an object gets instantiated.
- The constructor should not proceed with keywords as
abstract
,static
,final
, andsynchronized
.
the Default Constructor in Java
The Java default constructor is often called a no-args
constructor. Below, you can check out the code block to understand the default constructor in Java.
public class Main {
public static void main(String[] args) {
B b = new B();
System.out.println(b.a);
}
}
class B {
int a;
}
In the code block above, you’ll observe that there are two classes; the first holds the main
method, and the other one is written to depict the constructor’s functionality.
The main
function is the entry point of the program, and the execution starts here. So the driver class statement, new B()
, calls the public no-argument
constructor of the B
class. Since there was no explicit definition of the given constructor, the compiler creates a default constructor on its own and invokes that in the statement above.
The constructor initializes the variables of the class. When no constructor gets defined, the compiler initializes the value of the variable to their defaults. When the variable a
of the B
class gets referenced in the println
function, the value printed is in the output below.
Output:
0
the Parameterized Constructor in Java
The use of parameterized constructors is to pass the number of parameters at the time of the object creation. Below is the code block that demonstrates its function.
public class Main {
public static void main(String[] args) {
A a = new A(1, "One");
System.out.println(a.a + " " + a.b);
// A a1= new A();
}
}
class A {
int a;
String b;
A(int a, String b) {
this.a = a;
this.b = b;
}
}
In the code block above, A
is a class to demonstrate the parameterized constructor. And another is the class that holds the main
method. In the function, the new A(1, "One")
statement internally calls the parameterized constructor of the A
class. The created object gets assigned to the reference of the class, and the definition of the constructor gets defined in the class.
The constructor, A(int a, String b)
, initializes the value of the a
and b
variables. The values passed in the parameters will get copied in the variables. When in the println
function, using the reference variable calls the class variables of A
class; it prints the instantiated value in the output with a space added in between.
Next to it, there is a commented statement. We comment on the statements from getting executed using the //
operator. The statement new A()
internally tries to find the no-argument
constructor of the class. If the compiler does not find any default constructor, it can create a default constructor.
This ability gets restricted to the case when there is no explicit constructor defined. So, in this case, a user has to create the no-args
constructor manually. The A a1= new A();
function throws a compile-time error. The two options to resolve the issue are to create a default constructor or change the signature of the existing parameterized constructor that leads the existing statement to a break. Hence, the line is commented.
Output:
1 One
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn