Abstract Class Constructor in Java
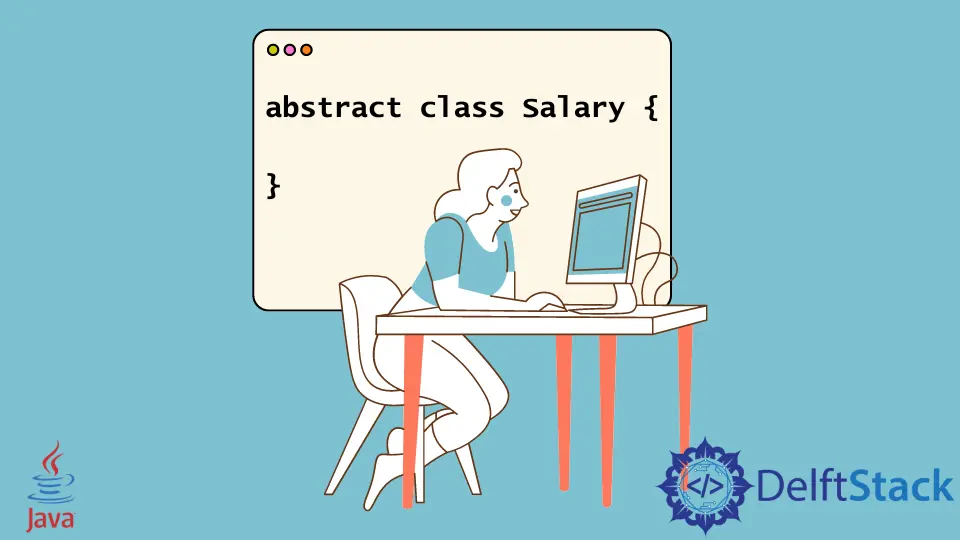
A constructor is used to initialize an object called by its name, and the constructor’s name is the same as the class’s name.
The abstract class is declared with an abstract keyword, abstract classes also have a constructor, and if we don’t define any constructor in the abstract class, then JVM will give us a default one.
This tutorial demonstrates how to create a constructor in an abstract class in Java.
Abstract Class Constructor in Java
When we indicate that a class is abstract, it can not be instantiated, but that doesn’t mean it cannot have the constructor. The abstract class can have a constructor in two conditions.
- When we want to perform initialization to the fields of an abstract class before the instantiation of a subclass is done.
- When we have defined final fields in the abstract class, but they are not initialized in the declaration, the constructor can be used to initialize these fields.
If we don’t define a constructor, the JVM will define a default one for us. Before defining a constructor, we must consider the following points.
- The subclass constructors can call one constructor from the abstract class, and they will have to call it if there is no argument constructor in the abstract class.
- All the constructors should be defined as protected in the abstract class because defining them as the public has no benefit.
- We can also define more than one constructor with different arguments.
Let’s have an example for the abstract class constructor that will calculate the gross salary, tax, and net salary by extending the abstract class with the constructor.
Code Example:
package delftstack;
abstract class SalaryCal {
double salary;
protected SalaryCal() {
salary = 0;
}
protected SalaryCal(double a) {
salary = a;
}
// Calculate is now an abstract method
abstract double Calculate();
}
class Salary extends SalaryCal {
Salary(double a) {
super(a);
}
// override calculate for Salary
double Calculate() {
System.out.println("Inside the Calculate method for Salary.");
double allowance = salary * 0.1; // 10 Percent other allowances
return salary + allowance;
}
}
class Tax extends SalaryCal {
Tax(double a) {
super(a);
}
// override calculate for Tax
double Calculate() {
System.out.println("Inside the Calculate method for Tax.");
return salary * 0.2; // 20 percent tax
}
}
public class Abstract_Constructor {
public static void main(String args[]) {
// SalaryCal Demo = new SalaryCal(1000); // illegal now because we can not instantiate an object
double Salary = 1000;
Salary S = new Salary(Salary);
Tax T = new Tax(Salary);
SalaryCal Salary_Calculation; // this is OK, no object is created
// Gross Salary Calculation
Salary_Calculation = S;
double gross_salary = Salary_Calculation.Calculate();
System.out.println("The Gross Salary is " + gross_salary);
// Tax Calculation
Salary_Calculation = T;
double salary_tax = Salary_Calculation.Calculate();
System.out.println("The total Tax on Salary is " + salary_tax);
// Net Salary
double net_salary = gross_salary - salary_tax;
System.out.println("The Net Salary is " + net_salary);
}
}
The superclass SalaryCal
is declared abstract and has two constructors, one without arguments which is the default, and the other with arguments. The two concrete classes, Salary
and Tax
, calculate the gross salary and tax.
Abstract constructors are frequently used for salary calculation. The main method also demonstrates that we can not instantiate the Abstract class not.
We can use the abstract class to get the values from concrete classes.
Output:
Inside the Calculate method for Salary.
The Gross Salary is 1100.0
Inside the Calculate method for Tax.
The total Tax on Salary is 200.0
The Net Salary is 900.0
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook