How to Call Another Constructor in Java
- Call One Constructor From Another Within the Same Class in Java
- Call One Constructor From Another From the Base Class in Java
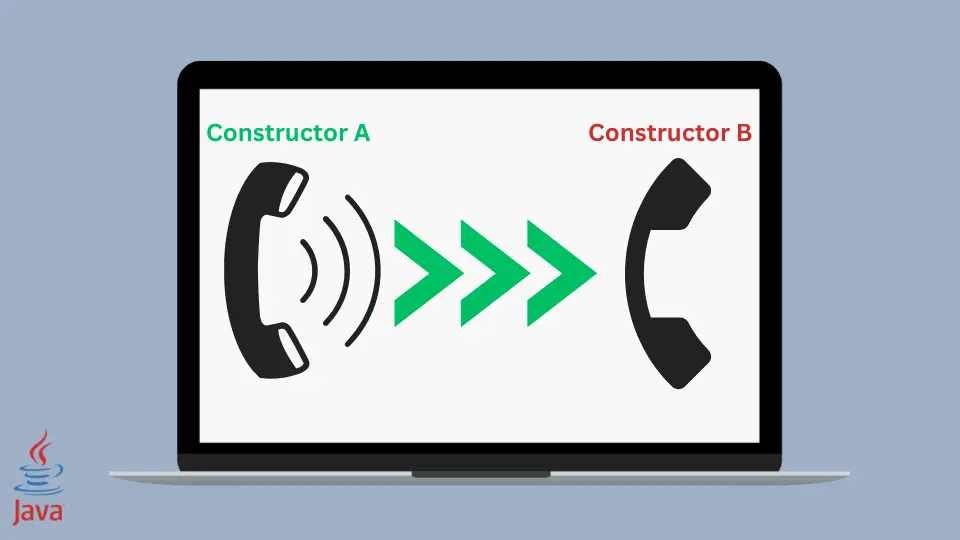
In Java, the sequence of invoking constructors upon initialization of the object is called constructor chaining. It is used when we want to invoke one constructor after another by using one instance.
Call One Constructor From Another Within the Same Class in Java
When we want to call one constructor from another constructor within the same class, we use the this
keyword. An expression that uses the this
keyword must be the first line of the constructor. The order doesn’t matter in the constructor chaining. It must have at least one constructor that doesn’t use the this
keyword.
In the below example, we have a class Test
that has 3 constructors. One is the default one without any parameters, while the other two are parameterized. In the main
method, we instantiate the class object with the new
keyword without any parameters passed.
It invokes the default constructor Test()
which redirects the call to the parameterized constructor Test(String name)
using this("Steve")
. The constructor Test(String name)
further redirects the call to the other parameterized constructor Test(int age, String place)
using this(30,"Texas")
.
The statements inside the Test(int age, String place)
are executed first. After that, the rest of the Test(String name)
constructor’s statements are executed. Finally, the default constructor’s remaining statement is executed, and the object is initialized successfully.
public class Test {
Test() {
this("Steve");
System.out.println("Default Constructor");
}
Test(String name) {
this(30, "Texas");
System.out.println("Name - " + name);
}
Test(int age, String place) {
System.out.println("Age- " + age + ", place- " + place);
}
public static void main(String[] args) {
Test test = new Test();
}
}
Output:
Age- 30, place- Texas
Name - Steve
Default Constructor
Call One Constructor From Another From the Base Class in Java
When there is more than one class with the inheritance relationship, we need to use the super
keyword to call the parent class constructor from the child class. Unlike the this
keyword, the JVM automatically puts the super
keyword.
The base class is the parent class that is extended by the derived class. The main method in which we instantiate the class object invokes the same class’s default constructor, which further redirects the call to the child class’s parameterized constructor using this("Adam")
.
super(name)
invokes the parameterized constructor Base(String name)
of the Base
class. The default constructor of the Base
class is invoked using this()
. The class variable is also initialized here using this.name = name
.
The code within the Base
class default constructor executes. Later the statements inside the parameterized constructor Base(String name)
executes. Finally, the remaining statement inside the parameterized constructor of the Derived
class is executed.
class Base {
String name;
Base() {
System.out.println("Default Constructor Base Class");
}
Base(String name) {
this();
this.name = name;
System.out.println("Parameterized Constructor Base Class called:- " + name);
}
}
class Derived extends Base {
Derived() {
this("Adam");
}
Derived(String name) {
super(name);
System.out.println("Parameterized Constructor Derived Class called: " + name);
}
public static void main(String[] args) {
Derived derived = new Derived();
}
}
Output:
Default Constructor Base Class
Parameterized Constructor Base Class called:- Adam
Parameterized Constructor Derived Class called: Adam
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn