How to Print New Line in Java
-
Print a New Line Using the
println()
Function in Java -
Print a New Line Using the Escape Sequence
\n
Character in Java -
Print a New Line Using
getProperty()
Method in Java -
Print a New Line Using the
lineSeparator()
Method in Java -
Print a New Line Using
%n
Newline Character in Java
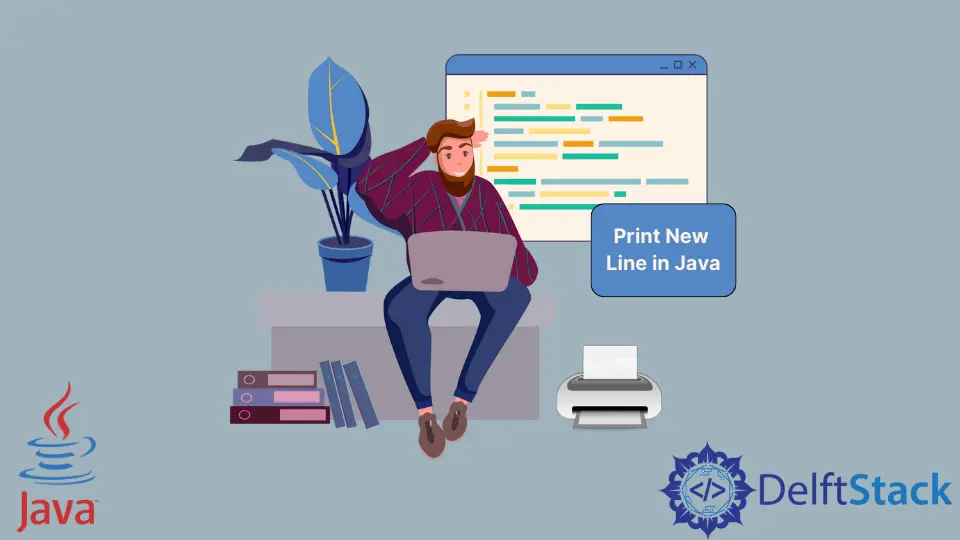
A new line signifies the end of a line or the start of a new line. It is also known as linebreak, EOL
(end Of line), or a line feed character.
In the Java language, there are various ways to print a new line. We can use escape sequence or predefined methods of the System
class.
Print a New Line Using the println()
Function in Java
In the below code block, we use the println()
function to print a new line. The System
class contains various useful methods and fields, and we cannot instantiate this class. The out
is the standard output stream object present in the System
class. Typically this stream corresponds to display output specified by the host environment or user. println()
is a method in the PrintStream
class. It prints a String and then terminates the line. It takes a string as a parameter that we want to print on the console output. The print()
function prints a string. If the argument is null, then the string null
is printed. Otherwise, the string’s characters get converted into bytes according to the platform’s default character encoding.
package new_line;
public class WaysToPrintNewLine {
public static void main(String[] args) {
System.out.println("Line1");
System.out.print("Line2");
}
}
Below is the output of the above two lines.
Line1
Line2
Print a New Line Using the Escape Sequence \n
Character in Java
There are some cases where we want to print a new line within the text in the console output. Using the println()
method for such a case would be a redundant task. In such cases, we can use the escape sequence for better code readability. An escape sequence is a character that consists of a backslash \
just before it. Examples of such characters are:
- We use
\t
to insert a tab in the text. - We use
\b
to insert a backspace in the text. - We use
\n
to insert a new line in the text.
In the below code, \n
escape sequence is used to break inline statements into two different lines.
package new_line;
public class WaysToPrintNewLine {
public static void main(String[] args) {
System.out.println("Hi, I am Lee"
+ "\n"
+ "I will help you write the code.");
}
}
The output of the above code is similar to the System.getProperty
code output.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn