How to Print Variables in Java
-
Print Variable Using the
print()
Function in Java -
Print Variable Using the
println()
Function in Java -
Print Variable Using the
printf()
Function - Conclusion
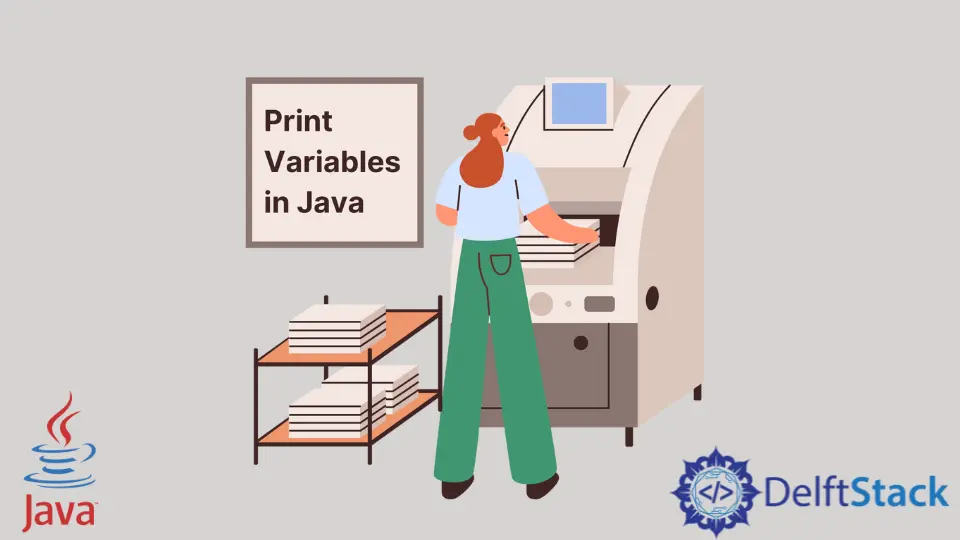
We can store data in a computer program using a variable. A variable is the name of a memory region that holds the value while we execute the Java program.
Also, each variable has a data type assigned to it. This article will discuss different ways to print variables in Java.
If we are given a variable in Java, we can print it by using the print()
method, the println()
method, and the printf()
method. Let’s go and take a closer look at how they work.
Print Variable Using the print()
Function in Java
We can print variables using the print()
method. The first element is the System
, a final class specified in the java.lang
package.
Then we have the second element as the out
, public, and static member field of the System
class. The last element that we have is the print()
method, which is a public method that we can use on the out
member field.
Let’s consider a scenario where we want to print the details of a product, including its name, price, and quantity, all on the same line without introducing line breaks. Here’s a simple Java code snippet using the print()
method.
public class VariablePrintingExample {
public static void main(String[] args) {
String productName = "Laptop";
double price = 899.99;
int quantity = 3;
// Printing variables on the same line
System.out.print("Product: " + productName + ", Price: $" + price + ", Quantity: " + quantity);
}
}
In this example, we first declare three variables: productName
(a String), price
(a double), and quantity
(an integer), representing the name, price, and quantity of a product, respectively.
The key part of the code is the System.out.print()
statement. Instead of using println()
, which appends a newline character after printing, we use print()
to keep the output on the same line.
The string within the print()
method is constructed using concatenation. We concatenate literal strings and the variables themselves, using the +
operator to join them together.
For example, "Product: " + productName
combines the literal string Product:
with the value stored in the productName
variable.
The result is a single line of output that displays the concatenated information about the product, including its name, price, and quantity.
Output:
Product: Laptop, Price: $899.99, Quantity: 3
Print Variable Using the println()
Function in Java
We can also use System.out.println()
to print a variable in Java. The only difference is that when we utilize the println()
method, the cursor moves to the next line after printing the output.
Consider a scenario where we want to display the details of a product—its name, price, and quantity—each on a new line. Below is a Java code snippet that utilizes the println()
method to achieve this.
public class VariablePrintingExample {
public static void main(String[] args) {
String productName = "Laptop";
double price = 899.99;
int quantity = 3;
// Printing variables on separate lines
System.out.println("Product: " + productName);
System.out.println("Price: $" + price);
System.out.println("Quantity: " + quantity);
}
}
In this example, we start by declaring three variables: productName
(a String), price
(a double), and quantity
(an integer), representing the name, price, and quantity of a product, respectively.
To print these variables on separate lines, we use three consecutive System.out.println()
statements. Each statement is responsible for printing a specific piece of information.
Within each println()
statement, we use concatenation to build a string that combines a literal description (e.g., Product:
) with the value stored in the corresponding variable. For instance, "Product: " + productName
combines the literal string Product:
with the value stored in the productName
variable.
The use of println()
ensures that each piece of information is printed on a new line. This is particularly useful for enhancing the readability of the output and creating a structured presentation.
Output:
Product: Laptop
Price: $899.99
Quantity: 3
Print Variable Using the printf()
Function
We can also use the printf()
method to print a variable in Java. Unlike the above two ways, when invoked, the printf()
method needs format specifiers (like %d
, %f
, %c
, %s
etc.).
Let’s delve into a scenario where we want to display information about a product—its name, price, and quantity—using the printf()
method to format the output precisely.
public class VariablePrintingExample {
public static void main(String[] args) {
String productName = "Laptop";
double price = 899.99;
int quantity = 3;
// Using printf() for formatted output
System.out.printf("Product: %s%n", productName);
System.out.printf("Price: $%.2f%n", price);
System.out.printf("Quantity: %d%n", quantity);
}
}
In this example, we begin by declaring three variables: productName
(a String), price
(a double), and quantity
(an integer), representing the name, price, and quantity of a product, respectively.
The printf()
method allows us to format the output with placeholders. The %s
in the first printf()
statement is a placeholder for a string.
Similarly, %f
in the second statement is a placeholder for a floating-point number, and %d
in the third statement is a placeholder for an integer.
Within each printf()
statement, the placeholders are followed by the variables or values that should replace them in the final output. For example, %s
is replaced by the value stored in the productName
variable.
In the second printf()
statement, %.2f
specifies that the floating-point number (price, in this case) should be displayed with two decimal places. This level of precision can be crucial in scenarios like representing currency values.
The %n
at the end of each printf()
statement represents a newline character, ensuring that each piece of information is printed on a new line.
Output:
Product: Laptop
Price: $899.99
Quantity: 3
Conclusion
In the realm of Java programming, this tutorial explored three vital methods—print()
, println()
, and printf()
—each offering unique capabilities for variable printing. The print()
method ensures a continuous output on the same line, employing concatenation for a concise result.
println()
introduces newline characters, fostering structured readability. Meanwhile, the powerful printf()
method enables precise formatting with format specifiers, ideal for meticulous control over variable presentation.
Mastering these methods equips developers to tailor output, whether it’s a streamlined display, structured readability, or precise formatting. The tutorial emphasizes the versatility these methods bring to effective information communication in Java programs.