How to Print Contents of Text File to Screen in Java
- Importance of Printing Text File Contents in Java
- Methods to Print Text File Contents in Java
- Conclusion
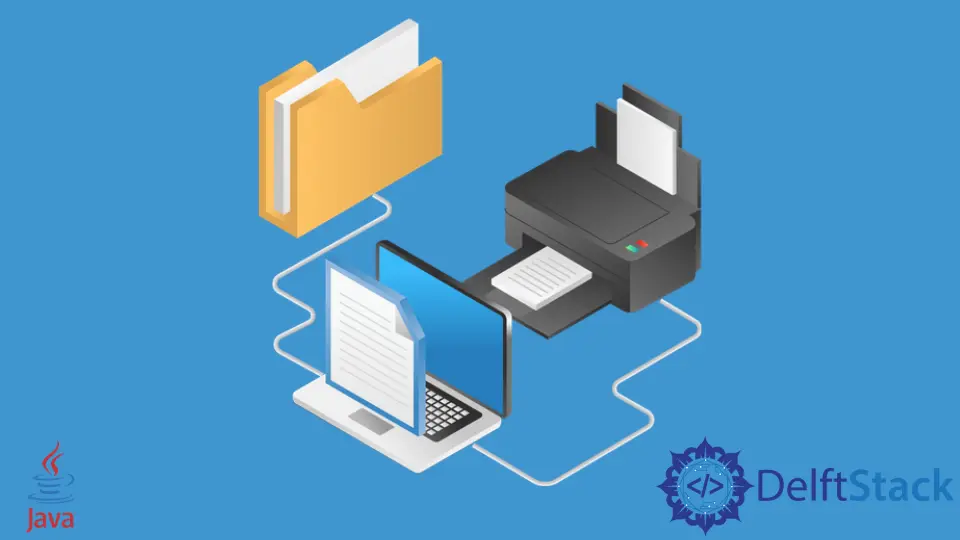
This article discusses the significance of printing text file contents in Java, exploring various methods, such as BufferedReader
, Scanner
, Files
, Paths
, FileInputStream
, and DataInputStream
, providing developers with insights and best practices for efficient file handling.
Importance of Printing Text File Contents in Java
Printing text file contents in Java is essential for applications that require reading and interpreting data stored in external files. This operation allows developers to access, analyze, and manipulate textual information, enabling the integration of file data into Java programs for diverse real-world applications.
Methods to Print Text File Contents in Java
BufferedReader
and FileReader
BufferedReader
and FileReader
are classes in Java commonly used for reading the contents of a file. Unlike other methods that directly operate on the raw file data, these classes provide a more efficient and convenient approach.
FileReader
is specifically designed for reading character data from files. It simplifies the process by reading data character by character but lacks the buffering mechanism.
On the other hand, BufferedReader
is a higher-level class that wraps around a FileReader
or another Reader
implementation. It introduces buffering, which means it reads data in larger chunks, enhancing performance by reducing the number of interactions with the file system.
Compared to methods using Scanner
, Files
and Paths
, or FileInputStream
and DataInputStream
, the combination of BufferedReader
and FileReader
is straightforward and commonly employed for reading text files line by line. This approach is particularly suitable when dealing with large files, offering a good balance between simplicity and efficiency.
Example.txt
:
Hello! My name is Pillow and I am a Chihuahua!
Let’s start with a complete working example that demonstrates how to print the contents of a text file to the screen using BufferedReader
and FileReader
:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadingExample {
public static void main(String[] args) {
try (BufferedReader br = new BufferedReader(new FileReader("Example.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
The Java code utilizes the BufferedReader
and FileReader
classes to efficiently read and print the contents of a text file. The import
statements introduce these classes, where BufferedReader
facilitates efficient character stream reading, and FileReader
is specifically designed for reading the characters of a file stream.
The main
method serves as the program’s entry point, employing the try-with-resources
statement for automatic resource management. Within this block, a BufferedReader
object is created by wrapping it around a FileReader
initialized with the target file’s name.
A string variable is used to store each line read from the file, and a while
loop iterates through each line until the end of the file is reached. The readLine()
method of BufferedReader
is employed to read each line, and System.out.println()
is used to print the contents to the console.
Exception handling is incorporated to manage potential IO exceptions that might arise during file processing. If an exception occurs, the details are caught, and the stack trace is printed to the console using e.printStackTrace()
.
This comprehensive approach to file reading offers a balance of simplicity and efficiency, making it suitable for various applications, particularly when dealing with large text files.
Output:
Assuming the file Example.txt
exists and contains some text, running this program will print each line of the file to the console.
Scanner
Scanner
is a class in Java commonly used for reading input, including file contents. It provides a flexible and user-friendly approach to parsing and processing data.
Unlike other methods that involve lower-level operations, such as FileInputStream
or BufferedReader
, Scanner
simplifies reading by allowing developers to specify the type of data they want to retrieve (e.g., integers, strings). While it might be slightly less efficient for large files, Scanner
is often preferred for its simplicity and versatility, making it a suitable choice when dealing with various data types within a file.
Example.txt
:
Hello! My name is Pillow and I am a Chihuahua!
Let’s dive into a complete working example that demonstrates how to print the contents of a text file using the Scanner
class:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileReadingExample {
public static void main(String[] args) {
try (Scanner scanner = new Scanner(new File("Example.txt"))) {
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine());
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
The Java code begins with import
statements introducing the necessary classes for file handling: File
for representing paths, FileNotFoundException
for signaling file opening failures, and Scanner
for simple text scanning.
The main
method serves as the program’s entry point, featuring a try-with-resources
block for automatic resource closure. The Scanner
is initialized by providing a File
object representing the target file (Example.txt
).
The while
loop continues as long as there are more lines to read, checking with hasNextLine()
. Within the loop, each line is read using nextLine()
and printed to the console using System.out.println()
.
Exception handling is incorporated to manage the potential occurrence of a FileNotFoundException
. This comprehensive approach using Scanner
provides a readable and straightforward method for reading and printing file contents, making it particularly suitable for scenarios involving various data types within a file.
If the specified file is not found, an exception is caught, and the details are printed to the console using e.printStackTrace()
.
Output:
Assuming the file Example.txt
exists and contains text, running this program will print each line of the file to the console. The simplicity and readability of the Scanner
class make this method an effective choice for basic file reading tasks in Java.
Files
and Paths
Files
and Paths
are classes in Java’s NIO (New I/O) package, offering a modern approach to file handling. Unlike other methods that involve multiple classes or lower-level operations, these classes simplify file operations by providing utility methods.
Paths
offers a convenient way to represent file system paths, allowing for easy conversion between strings and Path
instances. On the other hand, Files
provides static methods for common file operations, such as reading all lines from a file.
Compared to other methods, using Files
and Paths
is concise and expressive. The combination is particularly effective for scenarios where a clean, high-level approach to file handling is desired.
The code is simplified, making it easier to understand and maintain, especially for reading and processing file content in a modern Java application.
Example.txt
:
Hello! My name is Pillow and I am a Chihuahua!
Let’s delve into a complete working example that showcases how to utilize Files
and Paths
to read and print the contents of a text file:
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Paths;
public class FileReadingExample {
public static void main(String[] args) {
try {
Files.lines(Paths.get("Example.txt")).forEach(System.out::println);
} catch (IOException e) {
e.printStackTrace();
}
}
}
The Java code incorporates import
statements to introduce classes relevant to file and path operations, including IOException
for handling input/output errors, Files
for utility methods, and Paths
for converting strings or URIs to Path
instances.
The main
method serves as the program’s entry point, encapsulating the logic for reading and printing the contents of a text file. This includes creating a Path
object using Paths.get("Example.txt")
to represent the file path and utilizing Files.lines()
to read all lines from the file as a Stream<String>
.
The power of the Stream API is demonstrated through the forEach
method, which iterates over each line in the stream and prints it to the console.
Exception handling is incorporated to manage potential input/output exceptions that may occur during file processing. In case of an IOException
, the details are caught, and the stack trace is printed to the console using e.printStackTrace()
.
This approach using Files
and Paths
provides a concise and expressive method for reading and processing file content, particularly suited for scenarios where a clean, high-level approach is desired.
Output:
Assuming the file Example.txt
exists and contains text, running this program will print each line of the file to the console. The concise syntax provided by Files
and Paths
makes this method an elegant and effective choice for reading and processing file content in Java.
FileInputStream
and DataInputStream
Reading and printing the contents of a text file is a fundamental operation in Java programming. While there are various approaches to achieve this, using FileInputStream
and DataInputStream
provides a low-level yet powerful method.
These classes allow for reading the raw bytes of a file and interpreting them as textual data.
Example.txt
:
Hello! My name is Pillow and I am a Chihuahua!
Let’s delve into a complete working example that demonstrates how to use FileInputStream
and DataInputStream
to read and print the contents of a text file:
import java.io.DataInputStream;
import java.io.FileInputStream;
import java.io.IOException;
public class FileReadingExample {
public static void main(String[] args) {
try (DataInputStream dis = new DataInputStream(new FileInputStream("Example.txt"))) {
while (dis.available() > 0) {
System.out.println(dis.readLine());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
FileInputStream
and DataInputStream
are classes in Java used for reading binary data from files. FileInputStream
reads raw bytes from a file, and DataInputStream
extends this functionality by providing methods for reading primitive data types in a machine-independent way.
Compared to other methods, using FileInputStream
and DataInputStream
involves a more low-level approach, dealing with raw bytes and binary data. While this method is efficient for specific use cases, it might be less convenient for reading and processing text files or handling various data types.
In scenarios where the goal is to read and interpret raw binary data or when dealing with specific binary file formats, FileInputStream
and DataInputStream
can provide a suitable solution. However, for general-purpose text file reading or higher-level file processing, other classes like BufferedReader
or Files
and Paths
might be more straightforward and expressive.
Output:
Assuming the file Example.txt
exists and contains text, running this program will print each line of the file to the console. The FileInputStream
and DataInputStream
combination provides a way to read the file at a lower level, interpreting the bytes as lines of text.
Conclusion
We have examined different Java methods for reading and printing file contents. The use of BufferedReader
and FileReader
offers a fundamental and efficient approach suitable for various applications, providing clarity for developers at all levels.
The Scanner
class presents a concise solution for basic text file reading, enhancing the toolkit for Java developers and streamlining input operations. Exploring Files
and Paths
demonstrates the simplicity and expressiveness of Java’s NIO package, improving code readability and efficiency.
Lastly, employing FileInputStream
and DataInputStream
offers a lower-level perspective, valuable for handling binary data or situations requiring precise control over file reading. Each method equips Java developers with diverse skills to address real-world challenges.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn