Java system.out.println() Method
-
What Is
System.out.println()
Method? -
How Does
System.out.println()
Work? -
Role of
toString()
in theprint()
Method - Summary
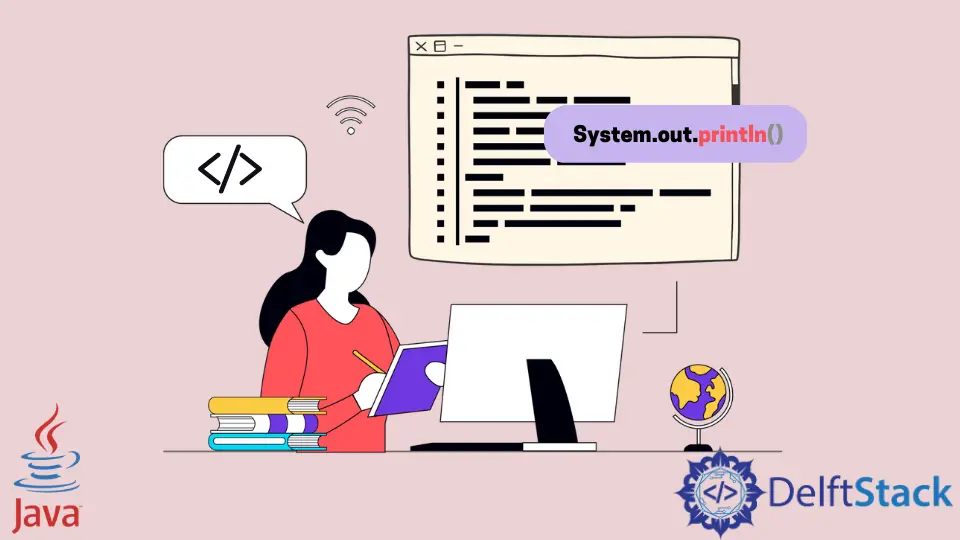
This tutorial introduces how the System.out.println()
method works in Java and lists some example codes to understand the topic.
The System.out.print()
is a very frequently used method for printing to the console or the standard output. This method is sometimes called the print line method. In addition to printing to console, the println()
method moves the cursor to a new line.
In this tutorial, we will try to understand the internal working of this method.
What Is System.out.println()
Method?
- The
System.out.println()
can be broken into three parts. - The
System
is afinal
class of thejava.lang
package and it is automatically initialised when the JVM starts. TheinitializeSystemClass()
is used to initialise it. - The
System
class contains an instance of thePrintStream
class. This instance variable is calledout
. It is defined with the modifierspublic
,static
, andfinal
.
class System {
public static final PrintStream out;
// More Code Below
}
The PrintStream
class contains the print()
and the println()
methods. These methods are overloaded.
class PrintStream {
public void print(argument) {
// implementation
}
public void println() {
// implementation
}
// Overloaded print() and println() methods below
}
So, System.out
gives us the out
instance variable of the PrintStream
class. We can then call the print()
or println()
method on this instance variable.
How Does System.out.println()
Work?
- The
PrintStream
class contains multiple overloadedprint()
andprintln()
methods. They differ in the type of parameters accepted. - The return type of all of them is void.
- An overloaded method exists for all the primitive types.
- It also contains an overloaded method to print strings and another one for objects.
The code below demonstrates the working of the overloaded println()
methods.
public class PrintDemo {
public static void main(String args[]) {
int i = 10;
short s = 10;
long l = 10;
char c = 'A';
char[] charArr = {'A', 'B', 'C'};
boolean bool = true;
double d = 10.0;
float f = 10.0f;
String str = "hello";
Object o = new Object();
System.out.println(); // terminate the current line
System.out.println(i); // print integer
System.out.println(s); // print short
System.out.println(l); // print long
System.out.println(c); // print char
System.out.println(charArr); // print char array
System.out.println(bool); // print boolean
System.out.println(d); // print double
System.out.println(f); // print float
System.out.println(str); // print String
System.out.println(o); // print Object
}
}
Output:
10
10
10
A
ABC
true
10.0
10.0
hello
java.lang.Object@433c675d
Arguments Passed to the println()
Method
- A beginner may think that the
print()
andprintln()
methods take a variable number of arguments (varargs
), but this is not the case. - For example, in the code below, we are trying to print an integer, a string, and a character.
public class PrintDemo {
public static void main(String args[]) {
int i = 10;
String s = "hello";
char c = 'O';
System.out.println(i + s + c);
}
}
Output:
10helloO
- But we are not passing three different arguments. A comma separates the arguments of a method.
- Instead, they are concatenated together using the
+
operator in theprintln()
method. - The
+
operator used with a string will lead to string concatenation and returns a string. - In the above code, the integer is first concatenated with the string, and the resulting string is again concatenated with the char variable.
Another thing to note is that the argument passed to the method is evaluated from left to right. So if the first two variables passed are integers, then normal arithmetic addition takes place, and the result of addition is concatenated with the string.
public class PrintDemo {
public static void main(String args[]) {
System.out.println(10 + 10 + "hello"); // first integer addition and then string concatenation
}
}
Output:
20hello
But string concatenation will take place if two more integers are present after the string.
public class PrintDemo {
public static void main(String args[]) {
System.out.println(10 + 10 + "hello" + 1 + 1); // 20hello11 not 20hello2
}
}
Output:
20hello11
We can apply the rule shown below from left to right to compute the output. If no strings are present, then normal arithmetic addition takes place.
(any data type + string) = (string + any data type) = (concatenated string)
Role of toString()
in the print()
Method
The print()
and println()
methods implicitly call the toString()
method on the argument. It converts the argument into a string. This is very useful if we want to print user-defined class instances in a particular way.
We can override the toString()
method in our class to print our object in different formats. The following example demonstrates this.
Example: Without Overriding the toString()
method.
class Demo {
String field1;
String field2;
Demo(String f1, String f2) {
this.field1 = f1;
this.field2 = f2;
}
}
public class PrintDemo {
public static void main(String args[]) {
Demo d = new Demo("f1", "f2");
System.out.print(d);
}
}
Output:
Demo@433c675d
Example: After Overriding the toString()
Method.
class Demo {
String field1;
String field2;
Demo(String f1, String f2) {
this.field1 = f1;
this.field2 = f2;
}
@Override
public String toString() {
return field1 + " " + field2;
}
}
public class PrintDemo {
public static void main(String args[]) {
Demo d = new Demo("f1", "f2");
System.out.print(d);
}
}
Output:
f1 f2
Summary
The print()
and println()
methods are part of the PrintStream
class. They are accessed by using the System.out
instance variable. These methods are overloaded to deal with different argument types. Remember that these methods do not take a variable number of arguments. In this tutorial, we learned how the System.out.print()
and System.out.println()
methods work.