Postfix Expression in Java
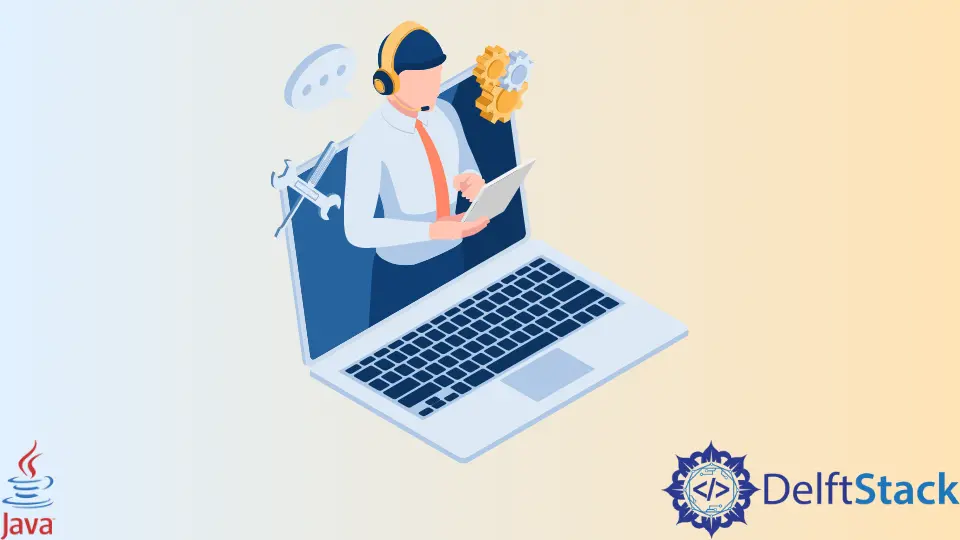
Postfix expressions are easy to evaluate and faster than the infix expressions as we don’t need to handle or follow any operator precedence rule. In addition, postfix expressions don’t contain any brackets.
We can quickly solve any postfix expressions in Java. In this article, we will learn how to evaluate a Postfix expression in Java, along with some necessary examples and explanations to make the topic easier.
Evaluate Postfix Expression in Java
Before we start, we must understand how a Postfix expression is calculated. Let’s solve a Postfix algorithm step by step by following the below table:
Expression: 82*9+
Character | Stack | Explanation
-------------------------------------------
8 8 8 is an Operand and pushed to Stack
2 8 2 2 is an Operand and pushed to Stack
* 16 (8*2) * is an Operator.
Poped 8 and 2 and multiply
them. Lastly pushed the result to Stack
9 16 9 9 is an Operand and pushed to Stack
+ 25 (16+9) + is an operator,
Stack popped 12 and 9 and added them.
Lastly pushed the result to Stack.
Result: 25
We have already learned a practical example of how we can solve a postfix algorithm. Now let’s solve the above expression programmatically using java. To solve a postfix algorithm in Java, you can follow the below example:
import java.util.Stack;
public class Postfix {
// Method to evaluate the postfix expression
static int EvaluatePostfix(String EXP) {
// Creating a stack
Stack<Integer> EqStack = new Stack<>();
// Scanning all the characters from the expression string
for (int i = 0; i < EXP.length(); i++) {
char ch = EXP.charAt(i);
// If the character is an operator then push it to the stack
if (Character.isDigit(ch))
EqStack.push(ch - '0');
// If the character is the operator, pop two elements for
// the stack and performs the mathematical operation.
else {
int Val_1 = EqStack.pop(); // Getting the first element
int Val_2 = EqStack.pop(); // Getting the second element
switch (ch) {
case '+':
EqStack.push(Val_2 + Val_1); // Perform add operation
break;
case '-':
EqStack.push(Val_2 - Val_1); // Perform subtraction operation
break;
case '/':
EqStack.push(Val_2 / Val_1); // Perform divide operation
break;
case '*':
EqStack.push(Val_2 * Val_1); // Perform multiply operation
break;
}
}
}
return EqStack.pop();
}
public static void main(String[] args) {
String EXP = "82*9+"; // The expression string
System.out.println("Postfix evaluation result: " + EvaluatePostfix(EXP));
}
}
We already explained the purpose of each line. After running the above example code, you will get the output below in your console.
Postfix evaluation result: 25
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn