Postfix-Ausdruck in Java
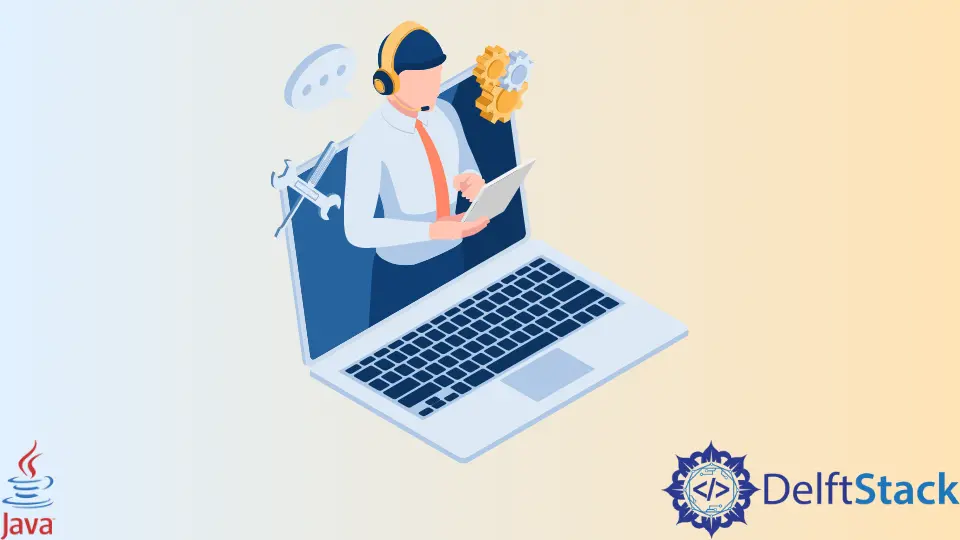
Postfix-Ausdrücke sind einfach auszuwerten und schneller als die Infix-Ausdrücke, da wir keine Vorrangregeln für Operatoren behandeln oder befolgen müssen. Außerdem enthalten Postfix-Ausdrücke keine Klammern.
Wir können alle Postfix-Ausdrücke in Java schnell lösen. In diesem Artikel lernen wir, wie man einen Postfix-Ausdruck in Java auswertet, zusammen mit einigen notwendigen Beispielen und Erklärungen, um das Thema zu vereinfachen.
Postfix-Ausdruck in Java auswerten
Bevor wir beginnen, müssen wir verstehen, wie ein Postfix-Ausdruck berechnet wird. Lassen Sie uns Schritt für Schritt einen Postfix-Algorithmus lösen, indem Sie der folgenden Tabelle folgen:
Expression: 82*9+
Character | Stack | Explanation
-------------------------------------------
8 8 8 is an Operand and pushed to Stack
2 8 2 2 is an Operand and pushed to Stack
* 16 (8*2) * is an Operator.
Poped 8 and 2 and multiply
them. Lastly pushed the result to Stack
9 16 9 9 is an Operand and pushed to Stack
+ 25 (16+9) + is an operator,
Stack popped 12 and 9 and added them.
Lastly pushed the result to Stack.
Result: 25
Wir haben bereits ein praktisches Beispiel kennengelernt, wie wir einen Postfix-Algorithmus lösen können. Lassen Sie uns nun den obigen Ausdruck programmgesteuert mit Java lösen. Um einen Postfix-Algorithmus in Java zu lösen, können Sie dem folgenden Beispiel folgen:
import java.util.Stack;
public class Postfix {
// Method to evaluate the postfix expression
static int EvaluatePostfix(String EXP) {
// Creating a stack
Stack<Integer> EqStack = new Stack<>();
// Scanning all the characters from the expression string
for (int i = 0; i < EXP.length(); i++) {
char ch = EXP.charAt(i);
// If the character is an operator then push it to the stack
if (Character.isDigit(ch))
EqStack.push(ch - '0');
// If the character is the operator, pop two elements for
// the stack and performs the mathematical operation.
else {
int Val_1 = EqStack.pop(); // Getting the first element
int Val_2 = EqStack.pop(); // Getting the second element
switch (ch) {
case '+':
EqStack.push(Val_2 + Val_1); // Perform add operation
break;
case '-':
EqStack.push(Val_2 - Val_1); // Perform subtraction operation
break;
case '/':
EqStack.push(Val_2 / Val_1); // Perform divide operation
break;
case '*':
EqStack.push(Val_2 * Val_1); // Perform multiply operation
break;
}
}
}
return EqStack.pop();
}
public static void main(String[] args) {
String EXP = "82*9+"; // The expression string
System.out.println("Postfix evaluation result: " + EvaluatePostfix(EXP));
}
}
Wir haben bereits den Zweck jeder Zeile erklärt. Nachdem Sie den obigen Beispielcode ausgeführt haben, erhalten Sie die folgende Ausgabe in Ihrer Konsole.
Postfix evaluation result: 25
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn