Java의 후위 표현식
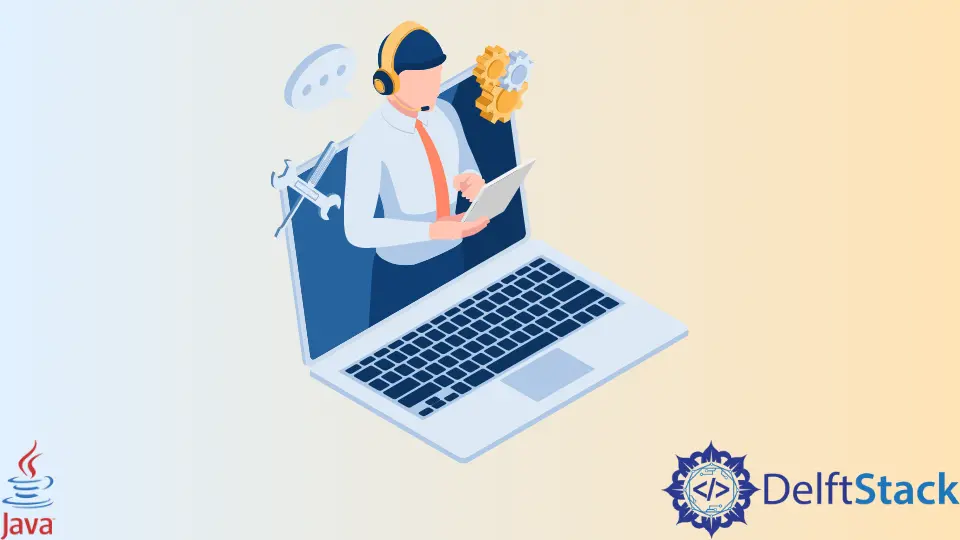
후위 표현식은 연산자 우선 순위 규칙을 처리하거나 따를 필요가 없기 때문에 중위 표현식보다 평가하기 쉽고 빠릅니다. 또한 접미사 식에는 대괄호가 포함되어 있지 않습니다.
우리는 자바에서 접미사 표현을 빠르게 풀 수 있습니다. 이 기사에서는 Java에서 Postfix 표현식을 평가하는 방법과 주제를 더 쉽게 만드는 데 필요한 몇 가지 예제 및 설명을 배웁니다.
Java에서 후위 표현식 평가
시작하기 전에 Postfix 표현식이 어떻게 계산되는지 이해해야 합니다. 아래 표에 따라 Postfix 알고리즘을 단계별로 해결해 봅시다.
Expression: 82*9+
Character | Stack | Explanation
-------------------------------------------
8 8 8 is an Operand and pushed to Stack
2 8 2 2 is an Operand and pushed to Stack
* 16 (8*2) * is an Operator.
Poped 8 and 2 and multiply
them. Lastly pushed the result to Stack
9 16 9 9 is an Operand and pushed to Stack
+ 25 (16+9) + is an operator,
Stack popped 12 and 9 and added them.
Lastly pushed the result to Stack.
Result: 25
우리는 이미 후위 알고리즘을 풀 수 있는 방법에 대한 실용적인 예를 배웠습니다. 이제 Java를 사용하여 프로그래밍 방식으로 위 식을 해결해 보겠습니다. Java에서 접미사 알고리즘을 해결하려면 아래 예제를 따를 수 있습니다.
import java.util.Stack;
public class Postfix {
// Method to evaluate the postfix expression
static int EvaluatePostfix(String EXP) {
// Creating a stack
Stack<Integer> EqStack = new Stack<>();
// Scanning all the characters from the expression string
for (int i = 0; i < EXP.length(); i++) {
char ch = EXP.charAt(i);
// If the character is an operator then push it to the stack
if (Character.isDigit(ch))
EqStack.push(ch - '0');
// If the character is the operator, pop two elements for
// the stack and performs the mathematical operation.
else {
int Val_1 = EqStack.pop(); // Getting the first element
int Val_2 = EqStack.pop(); // Getting the second element
switch (ch) {
case '+':
EqStack.push(Val_2 + Val_1); // Perform add operation
break;
case '-':
EqStack.push(Val_2 - Val_1); // Perform subtraction operation
break;
case '/':
EqStack.push(Val_2 / Val_1); // Perform divide operation
break;
case '*':
EqStack.push(Val_2 * Val_1); // Perform multiply operation
break;
}
}
}
return EqStack.pop();
}
public static void main(String[] args) {
String EXP = "82*9+"; // The expression string
System.out.println("Postfix evaluation result: " + EvaluatePostfix(EXP));
}
}
우리는 이미 각 줄의 목적을 설명했습니다. 위의 예제 코드를 실행하면 콘솔에 아래와 같은 출력이 표시됩니다.
Postfix evaluation result: 25
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn