Java の後置式
MD Aminul Islam
2023年10月12日
Java
Java Expression
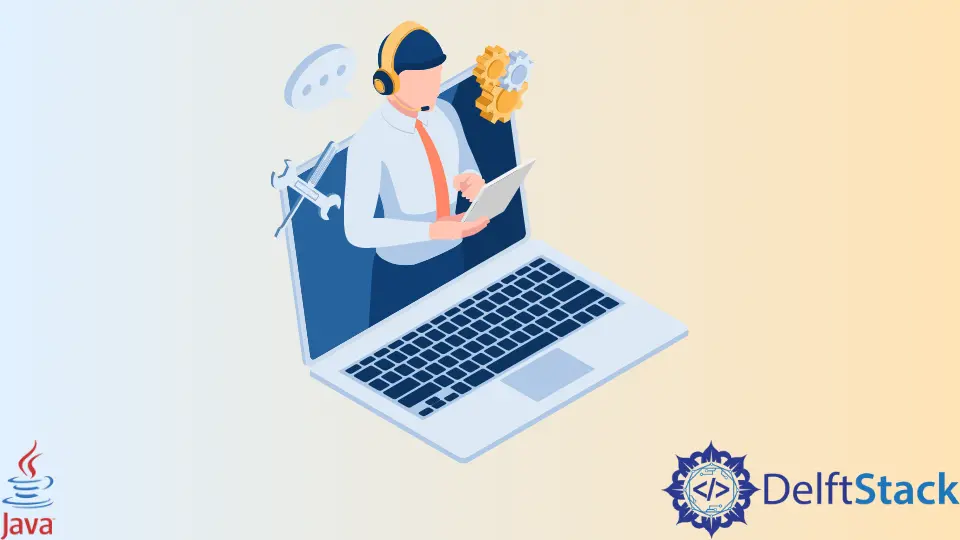
後置式は評価が簡単で、演算子の優先順位規則を処理したり従ったりする必要がないため、中置式よりも高速です。 さらに、後置式には括弧が含まれていません。
Java の後置式をすばやく解決できます。 この記事では、トピックを簡単にするために必要な例と説明とともに、Java で Postfix 式を評価する方法を学びます。
Java で後置式を評価する
始める前に、Postfix 式がどのように計算されるかを理解する必要があります。 以下の表に従って、Postfix アルゴリズムを段階的に解決してみましょう。
Expression: 82*9+
Character | Stack | Explanation
-------------------------------------------
8 8 8 is an Operand and pushed to Stack
2 8 2 2 is an Operand and pushed to Stack
* 16 (8*2) * is an Operator.
Poped 8 and 2 and multiply
them. Lastly pushed the result to Stack
9 16 9 9 is an Operand and pushed to Stack
+ 25 (16+9) + is an operator,
Stack popped 12 and 9 and added them.
Lastly pushed the result to Stack.
Result: 25
後置アルゴリズムをどのように解決できるかの実用的な例をすでに学びました。 それでは、Java を使用してプログラムで上記の式を解いてみましょう。 Java で後置アルゴリズムを解決するには、次の例に従います。
import java.util.Stack;
public class Postfix {
// Method to evaluate the postfix expression
static int EvaluatePostfix(String EXP) {
// Creating a stack
Stack<Integer> EqStack = new Stack<>();
// Scanning all the characters from the expression string
for (int i = 0; i < EXP.length(); i++) {
char ch = EXP.charAt(i);
// If the character is an operator then push it to the stack
if (Character.isDigit(ch))
EqStack.push(ch - '0');
// If the character is the operator, pop two elements for
// the stack and performs the mathematical operation.
else {
int Val_1 = EqStack.pop(); // Getting the first element
int Val_2 = EqStack.pop(); // Getting the second element
switch (ch) {
case '+':
EqStack.push(Val_2 + Val_1); // Perform add operation
break;
case '-':
EqStack.push(Val_2 - Val_1); // Perform subtraction operation
break;
case '/':
EqStack.push(Val_2 / Val_1); // Perform divide operation
break;
case '*':
EqStack.push(Val_2 * Val_1); // Perform multiply operation
break;
}
}
}
return EqStack.pop();
}
public static void main(String[] args) {
String EXP = "82*9+"; // The expression string
System.out.println("Postfix evaluation result: " + EvaluatePostfix(EXP));
}
}
各行の目的についてはすでに説明しました。 上記のサンプル コードを実行すると、コンソールに以下の出力が表示されます。
Postfix evaluation result: 25
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: MD Aminul Islam
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn