Expresión Postfix en Java
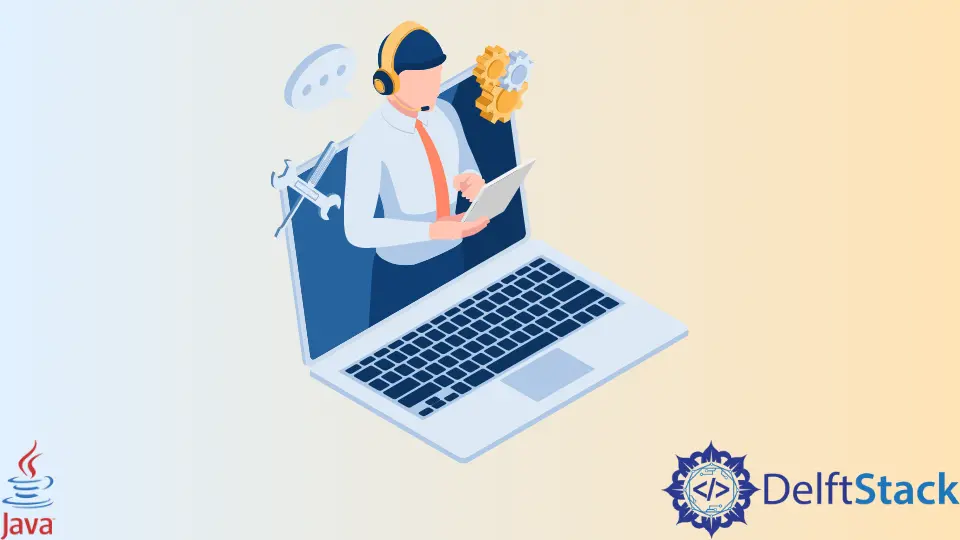
Las expresiones de sufijo son fáciles de evaluar y más rápidas que las expresiones de infijo, ya que no necesitamos manejar ni seguir ninguna regla de precedencia de operadores. Además, las expresiones de sufijo no contienen corchetes.
Podemos resolver rápidamente cualquier expresión de sufijo en Java. En este artículo, aprenderemos cómo evaluar una expresión de Postfix en Java, junto con algunos ejemplos y explicaciones necesarios para facilitar el tema.
Evaluar la expresión Postfix en Java
Antes de comenzar, debemos comprender cómo se calcula una expresión Postfix. Resolvamos un algoritmo de Postfix paso a paso siguiendo la siguiente tabla:
Expression: 82*9+
Character | Stack | Explanation
-------------------------------------------
8 8 8 is an Operand and pushed to Stack
2 8 2 2 is an Operand and pushed to Stack
* 16 (8*2) * is an Operator.
Poped 8 and 2 and multiply
them. Lastly pushed the result to Stack
9 16 9 9 is an Operand and pushed to Stack
+ 25 (16+9) + is an operator,
Stack popped 12 and 9 and added them.
Lastly pushed the result to Stack.
Result: 25
Ya hemos aprendido un ejemplo práctico de cómo podemos resolver un algoritmo de sufijo. Ahora resolvamos la expresión anterior mediante programación usando java. Para resolver un algoritmo de postfijo en Java, puede seguir el siguiente ejemplo:
import java.util.Stack;
public class Postfix {
// Method to evaluate the postfix expression
static int EvaluatePostfix(String EXP) {
// Creating a stack
Stack<Integer> EqStack = new Stack<>();
// Scanning all the characters from the expression string
for (int i = 0; i < EXP.length(); i++) {
char ch = EXP.charAt(i);
// If the character is an operator then push it to the stack
if (Character.isDigit(ch))
EqStack.push(ch - '0');
// If the character is the operator, pop two elements for
// the stack and performs the mathematical operation.
else {
int Val_1 = EqStack.pop(); // Getting the first element
int Val_2 = EqStack.pop(); // Getting the second element
switch (ch) {
case '+':
EqStack.push(Val_2 + Val_1); // Perform add operation
break;
case '-':
EqStack.push(Val_2 - Val_1); // Perform subtraction operation
break;
case '/':
EqStack.push(Val_2 / Val_1); // Perform divide operation
break;
case '*':
EqStack.push(Val_2 * Val_1); // Perform multiply operation
break;
}
}
}
return EqStack.pop();
}
public static void main(String[] args) {
String EXP = "82*9+"; // The expression string
System.out.println("Postfix evaluation result: " + EvaluatePostfix(EXP));
}
}
Ya explicamos el propósito de cada línea. Después de ejecutar el código de ejemplo anterior, obtendrá el siguiente resultado en su consola.
Postfix evaluation result: 25
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn