How to Parse JSON in Java
-
Use the
json.simple
Library to Parse JSON in Java -
Use the
org.json
Library to Parse JSON in Java -
Use the
gson
Library to Parse JSON in Java -
Use the
JsonPath
Library to Parse JSON in Java
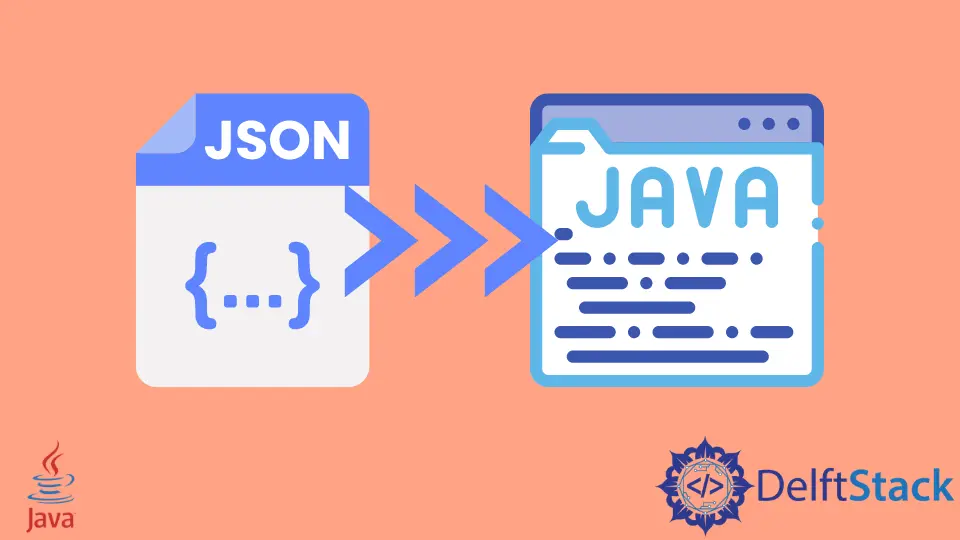
JSON is a light and text-based language for storing and transferring data. It is based on objects in JavaScript. JSON represents two structured types that are objects and arrays.
This tutorial demonstrates how to parse JSON in Java using various methods.
For our examples, we will work with the following JSON file.
{
"firstName": "Ram",
"lastName": "Sharma",
"age": 26
},
"phoneNumbers": [
{
"type": "home",
"phone-number": "212 888-2365"
}
]
}
Use the json.simple
Library to Parse JSON in Java
The first method is by using the json.simple
library to parse JSON in Java. We have to import two classes from java.simple
library, org.json.simple.JSONArray
and org.json.simple.JSONObject
.
The JSONArray
helps us parse elements in the form of an array, and the JSONObject
allows us to parse the objects present in the JSON text.
The following example demonstrates the use of this method.
import org.json.simple.JSONArray;
import org.json.simple.JSONObject;
import org.json.simple.parser.*;
public class JSONsimple {
public static void main(String[] args) throws Exception {
// parsing file "JSONExample.json"
Object ob = new JSONParser().parse(new FileReader("JSONFile.json"));
// typecasting ob to JSONObject
JSONObject js = (JSONObject) ob;
String firstName = (String) js.get("firstName");
String lastName = (String) js.get("lastName");
System.out.println("First name is: " + firstName);
System.out.println("Last name is: " + lastName);
}
}
Output:
First name is: Ram
Ladt name is: Sharma
In the above example, we have read a JSON file that is already there on the system and from that, we are printing the first and last name attributes from that file.
Here the JSONParser().parse()
function present in the org.json.simple.parser.*
parses the JSON text from the file. The js.get()
method here gets the value for the firstName
and the lastName
from the file.
Use the org.json
Library to Parse JSON in Java
This library provides a static method for reading a JSON string directly instead of reading from the file. The getJSONObject
and getJSONArray
methods make the work more simple and provide us the required input from the string entered by the user. Also, we do not have to typecast the object to JSONObject
as done in the earlier example.
See the code below.
import org.json.JSONArray;
import org.json.JSONObject;
public class JSON2 {
static String json = "{\"contacDetails\": {\n" + // JSON text is written here
" \"firstName\": \"Ram\",\n"
+ " \"lastName\": \"Sharma\"\n"
+ " },\n"
+ " \"phoneNumbers\": [\n"
+ " {\n"
+ " \"type\": \"home\",\n"
+ " \"phone-number\": \"212 888-2365\",\n"
+ " }\n"
+ " ]"
+ "}";
public static void main(String[] args) {
// Make a object
JSONObject ob = new JSONObject(json);
// getting first and last name
String firstName = ob.getJSONObject("contacDetails").getString("firstName");
String lastName = ob.getJSONObject("contacDetails").getString("lastName");
System.out.println("FirstName " + firstName);
System.out.println("LastName " + lastName);
// loop for printing the array as phoneNumber is stored as array.
JSONArray arr = obj.getJSONArray("phoneNumbers");
for (int i = 0; i < arr.length(); i++) {
String post_id = arr.getJSONObject(i).getString("phone-number");
System.out.println(post_id);
}
}
}
Output:
FirstName Ram
LastName Sharma
212 888-2365
Use the gson
Library to Parse JSON in Java
This library also has to be downloaded additionally. We have to import three classes from the gson
library that are com.google.gson.JsonArray
, com.google.gson.JsonObject
and com.google.gson.JsonParser
.
We can use both static strings as well as a file to parse JSON. The method names to get the input are slightly different from the previous one. For getting the object data we will be using getAsJsonObject()
and for array inputs, we will be using getAsJsonArray()
.
See the code below.
import com.google.gson.JsonArray;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class GsonMethod {
static String json = "{\"contacDetails\": {\n" + // JSON text is written here
" \"firstName\": \"Ram\",\n"
+ " \"lastName\": \"Sharma\"\n"
+ " },\n"
+ " \"phoneNumbers\": [\n"
+ " {\n"
+ " \"type\": \"home\",\n"
+ " \"phone-number\": \"212 888-2365\",\n"
+ " }\n"
+ " ]"
+ "}";
public static void main(String[] args) {
// Make an object
JsonObject ob = new JsonParser().parse(json).getAsJsonObject();
// getting first and last name
String firstName = ob.getAsJsonObject("contacDetails").get("firstName");
String lastName = ob.getAsJsonObject("contacDetails").get("lastName");
System.out.println("FirstName " + firstName);
System.out.println("LastName " + lastName);
// loop for printing the array as phoneNumber is stored as array.
JsonArray arr = obj.getAsJsonArray("phoneNumbers");
for (int i = 0; i < arr.length(); i++) {
String PhoneNumber = arr.getAsJsonObject(i).get("phone-number");
System.out.println(PhoneNumber);
}
}
}
Output:
FirstName Ram
LastName Sharma
212 888-2365
Use the JsonPath
Library to Parse JSON in Java
In this library, we will be using the read()
method for getting the data inputs. We don’t require to make an object here for calling the JSON text. We will be importing the com.jayway.jsonpath.JsonPath
class for this.
The following example demonstrates this method.
import com.jayway.jsonpath.JsonPath;
public class PathMethod {
static String json = "{\"contacDetails\": {\n" + // JSON text is written here
" \"firstName\": \"Ram\",\n"
+ " \"lastName\": \"Sharma\"\n"
+ " },\n"
+ " \"phoneNumbers\": [\n"
+ " {\n"
+ " \"type\": \"home\",\n"
+ " \"phone-number\": \"212 888-2365\",\n"
+ " }\n"
+ " ]"
+ "}";
public static void main(String[] args) {
// getting input
String firstName = JsonPath.read(json, "$.contactDetails.firstName");
System.out.println("FirstName " + firstName);
String lastName = JsonPath.read(json, "$.contactDetails.lastName");
System.out.println("LastName " + lastName);
Integer phoneNumbers = JsonPath.read(json, "$.phoneNumbers.length()");
for (int i = 0; i < phoneNumbers; i++) {
String number = JsonPath.read(json, "$.phoneNumber[" + i + "].phone-number");
System.out.println(number);
}
}
}
Output:
FirstName Ram
LastName Sharma
212 888-2365