How to Fix Error: Non-Static Variable Count Cannot Be Referenced From a Static Context in Java
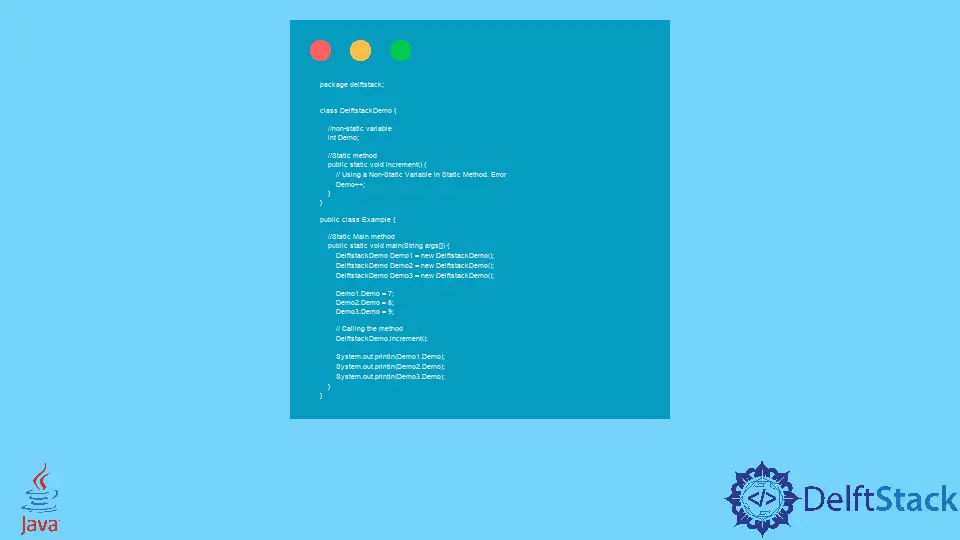
This tutorial demonstrates the error: non-static variable count cannot be referenced from a static context
in Java.
the Error: non-static variable count cannot be referenced from a static context
in Java
Most of the time, the error: non-static variable count cannot be referenced from a static context
occurs when we try to use a non-static member variable in the main method because the main()
method is static and is invoked automatically. We don’t need to create an object to invoke the main()
method.
To understand the error: non-static variable count cannot be referenced from a static context
, first, we need to understand the concept of static and non-static methods.
Static Methods
The static methods belong to a class but not to the instance of that class. The static method is called without the instance or object of the class.
The static methods can only access the static variables and other members of the static methods of their class and other classes. The static methods cannot access the non-static members of a class.
Non-Static Methods
Any method which doesn’t contain a Static
keyword is a non-static method. The non-static methods can access the static and non-static variables and members of the same class or another class.
The non-static methods can also change the value of static data.
Example of Static and Non-Static Methods in Java
Let’s try an example to demonstrate static and non-static methods.
package delftstack;
class DelftstackDemo {
// non-static variable
int Demo;
// Static method
public static void increment() {
// Using a Non-Static Variable in Static Method. Error
Demo++;
}
}
public class Example {
// Static Main method
public static void main(String args[]) {
DelftstackDemo Demo1 = new DelftstackDemo();
DelftstackDemo Demo2 = new DelftstackDemo();
DelftstackDemo Demo3 = new DelftstackDemo();
Demo1.Demo = 7;
Demo2.Demo = 8;
Demo3.Demo = 9;
// Calling the method
DelftstackDemo.increment();
System.out.println(Demo1.Demo);
System.out.println(Demo2.Demo);
System.out.println(Demo3.Demo);
}
}
The code above has two classes. In the first class, the code uses a non-static member variable in a static method, and the second class tries to change the values of the non-static member from the instance in the main method.
The code will throw an error.
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot make a static reference to the non-static field Demo
at delftstack.DelftstackDemo.increment(Example.java:12)
at delftstack.Example.main(Example.java:29)
The code above throws the error because a non-static member Demo
is used in the static method. Changing the method increment
to non-static and calling it with the first class instance will solve the problem.
See solution:
package delftstack;
class DelftstackDemo {
// non-static variable
int Demo;
// change to a non-static method
public void increment() {
// No error
Demo++;
}
}
public class Example {
// Static Main method
public static void main(String args[]) {
DelftstackDemo Demo1 = new DelftstackDemo();
DelftstackDemo Demo2 = new DelftstackDemo();
DelftstackDemo Demo3 = new DelftstackDemo();
Demo1.Demo = 7;
Demo2.Demo = 8;
Demo3.Demo = 9;
// Calling the method
Demo1.increment();
System.out.println(Demo1.Demo);
System.out.println(Demo2.Demo);
System.out.println(Demo3.Demo);
}
}
Now we are using a non-static member in the non-static method and calling a non-static method into the main static by the instance of the first class. It solves the problem.
See output:
8
8
9
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack