오류: Java의 정적 컨텍스트에서 비정적 변수 수를 참조할 수 없음
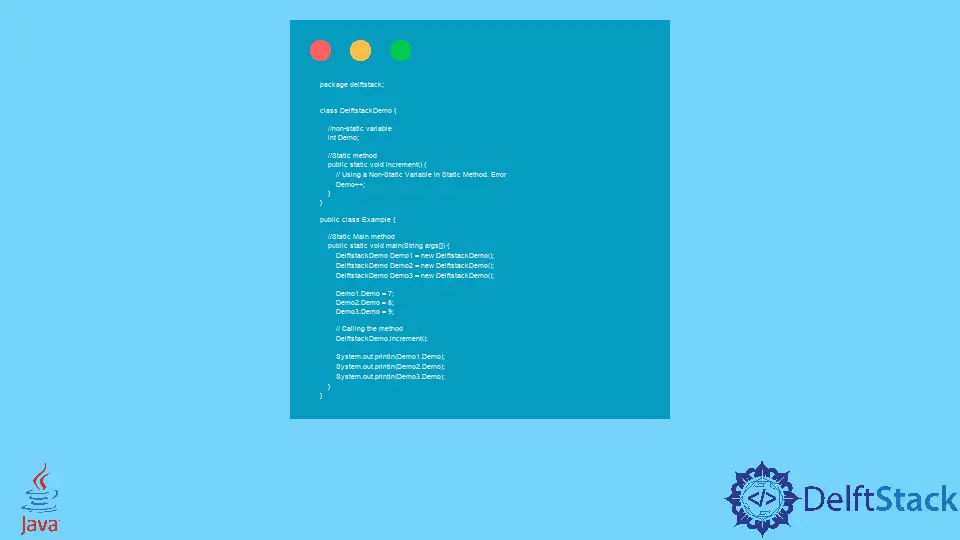
이 자습서에서는 Java의 오류: 비정적 변수 수를 정적 컨텍스트에서 참조할 수 없습니다
를 보여 줍니다.
Java의 오류: 정적 컨텍스트에서 비정적 변수 수를 참조할 수 없습니다
대부분의 경우 main()
메소드가 정적이고 자동으로 호출됩니다. main()
메소드를 호출하기 위해 객체를 생성할 필요가 없습니다.
오류: 정적 컨텍스트에서 비정적 변수 수를 참조할 수 없음
을 이해하려면 먼저 정적 및 비정적 메소드의 개념을 이해해야 합니다.
정적 메서드
정적 메서드는 클래스에 속하지만 해당 클래스의 인스턴스에는 속하지 않습니다. 정적 메서드는 클래스의 인스턴스나 개체 없이 호출됩니다.
정적 메서드는 정적 변수와 해당 클래스 및 기타 클래스의 정적 메서드의 다른 멤버에만 액세스할 수 있습니다. 정적 메서드는 클래스의 비정적 멤버에 액세스할 수 없습니다.
비정적 방법
Static
키워드를 포함하지 않는 모든 메서드는 비정적 메서드입니다. 비정적 메서드는 정적 및 비정적 변수와 동일한 클래스 또는 다른 클래스의 멤버에 액세스할 수 있습니다.
비정적 메서드는 정적 데이터의 값을 변경할 수도 있습니다.
Java의 정적 및 비정적 메서드의 예
정적 메서드와 비정적 메서드를 보여 주는 예제를 살펴보겠습니다.
package delftstack;
class DelftstackDemo {
// non-static variable
int Demo;
// Static method
public static void increment() {
// Using a Non-Static Variable in Static Method. Error
Demo++;
}
}
public class Example {
// Static Main method
public static void main(String args[]) {
DelftstackDemo Demo1 = new DelftstackDemo();
DelftstackDemo Demo2 = new DelftstackDemo();
DelftstackDemo Demo3 = new DelftstackDemo();
Demo1.Demo = 7;
Demo2.Demo = 8;
Demo3.Demo = 9;
// Calling the method
DelftstackDemo.increment();
System.out.println(Demo1.Demo);
System.out.println(Demo2.Demo);
System.out.println(Demo3.Demo);
}
}
위의 코드에는 두 개의 클래스가 있습니다. 첫 번째 클래스에서 코드는 정적 메서드의 비정적 멤버 변수를 사용하고 두 번째 클래스는 기본 메서드의 인스턴스에서 비정적 멤버의 값을 변경하려고 시도합니다.
코드에서 오류가 발생합니다.
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
Cannot make a static reference to the non-static field Demo
at delftstack.DelftstackDemo.increment(Example.java:12)
at delftstack.Example.main(Example.java:29)
위의 코드는 정적 메서드에서 비정적 멤버인 Demo
를 사용하기 때문에 오류가 발생합니다. increment
메서드를 비정적으로 변경하고 첫 번째 클래스 인스턴스로 호출하면 문제가 해결됩니다.
해결 방법 보기:
package delftstack;
class DelftstackDemo {
// non-static variable
int Demo;
// change to a non-static method
public void increment() {
// No error
Demo++;
}
}
public class Example {
// Static Main method
public static void main(String args[]) {
DelftstackDemo Demo1 = new DelftstackDemo();
DelftstackDemo Demo2 = new DelftstackDemo();
DelftstackDemo Demo3 = new DelftstackDemo();
Demo1.Demo = 7;
Demo2.Demo = 8;
Demo3.Demo = 9;
// Calling the method
Demo1.increment();
System.out.println(Demo1.Demo);
System.out.println(Demo2.Demo);
System.out.println(Demo3.Demo);
}
}
이제 비정적 메서드에서 비정적 멤버를 사용하고 첫 번째 클래스의 인스턴스에 의해 비정적 메서드를 기본 정적 메서드로 호출합니다. 그것은 문제를 해결합니다.
출력 참조:
8
8
9
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook