How to Create a Transparent Scene in JavaFX
-
Create a Transparent Background Using
-fx-background-color: transparent
- Create a Transparent Background Using External CSS file
-
Create a Transparent
Stage
Background -
Create a Transparent
Scene
andStage
Background - Conclusion
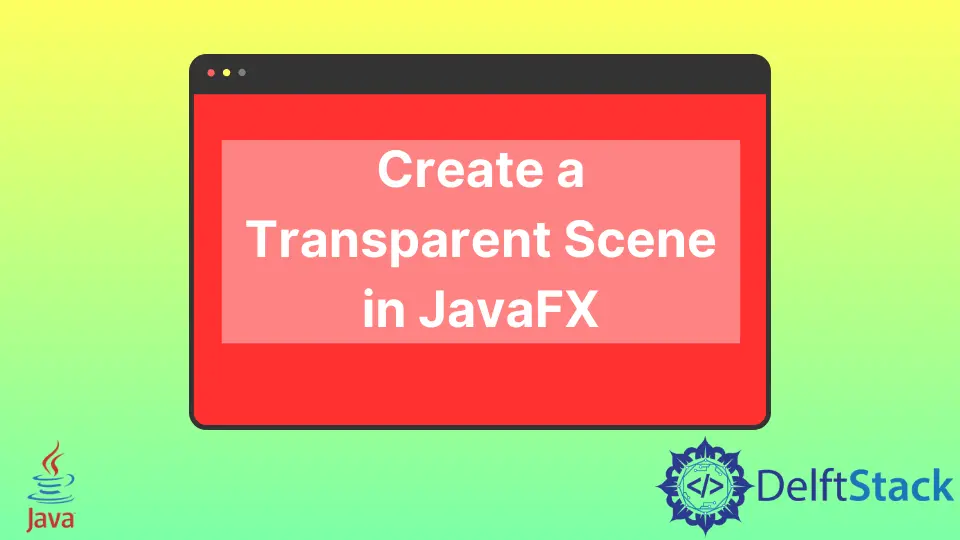
Explore a diverse array of techniques for achieving a transparent background in JavaFX applications. From setting transparent colors and employing CSS styling, this guide offers a thorough exploration of methods that cater to various preferences and application requirements.
Making a background transparent is crucial for enhancing the visual appeal and user experience of graphical interfaces. It allows seamless integration of application elements, eliminating visual barriers and enabling a more modern and aesthetically pleasing design that can adapt to diverse styles and contexts.
In this article, you can anticipate a detailed exploration of diverse methods to achieve a transparent background in JavaFX applications. The guide covers a range of techniques, providing insights into each approach’s strengths and applications.
Whether you’re a beginner or an experienced JavaFX developer, this article aims to equip you with the knowledge to enhance the visual appeal of your applications through transparent backgrounds.
Create a Transparent Background Using -fx-background-color: transparent
To achieve a transparent background in JavaFX, utilize the CSS property -fx-background-color: transparent;
. This setting, applied to a UI element, renders its background completely see-through, allowing for modern and visually appealing designs.
This simple and effective CSS rule is instrumental in creating transparent backgrounds for various JavaFX components, enhancing the overall aesthetics of graphical user interfaces.
Example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class transparent extends Application {
@Override
public void start(Stage stage) {
// Create a button with a label
Button transparentButton = new Button("Click me!");
// Set transparent background color for the button
transparentButton.setStyle(
"-fx-background-color: transparent; -fx-border-color: white; -fx-border-width: 2;-fx-text-fill: white");
// Create a layout pane and add the button
StackPane layout = new StackPane();
layout.getChildren().add(transparentButton);
layout.setStyle("-fx-background-color: black");
// Create a scene with a light gray background
Scene scene = new Scene(layout, 300, 200);
// Set the scene for the stage
stage.setScene(scene);
// Set the stage title
stage.setTitle("Transparent Button Example");
// Show the stage
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
The code initiates with JavaFX import statements, essential for GUI creation. The transparent
class, extending Application
, is declared to build a JavaFX app.
The overridden start
method serves as the entry point, taking a Stage
parameter. Within, a button named transparentButton
is created, styled with a transparent background, white border, and text.
The transparency of the button is achieved through the use of the setStyle
method.
The button’s transparency is established through the application of specific style rules. Firstly, -fx-background-color: transparent;
ensures the background color is transparent, rendering it see-through.
Subsequently, -fx-border-color: white;
dictates a white border for the button, while -fx-border-width: 2;
determines the width of the border as 2 pixels.
Lastly, -fx-text-fill: white;
specifies the text color, setting it to white.
Together, these style properties contribute to the creation of a button with a transparent background, a white border, and white text, collectively enhancing its visual appeal.
A StackPane
layout, layout
, is established, incorporating the button. The layout’s background is set to black.
A scene
, associated with the layout is created with specified dimensions.
The scene
is set for the stage
, titled Transparent Button Example
, and the stage
is displayed using the show
method.
Output:
Upon running this JavaFX application, a stage
will appear with a single button labeled Click me!
. The button has a transparent background, white borders, and white text.
It is positioned on a black background, creating a visually appealing and modern UI. The concept of styling a button with a transparent background is effectively demonstrated through this example, showcasing how CSS styles can be applied to JavaFX elements to achieve a desired visual effect.
Create a Transparent Background Using External CSS file
Leveraging an external CSS file is pivotal for making backgrounds transparent in JavaFX. This approach streamlines code organization, promotes maintainability, and allows effortless customization.
By isolating styling in a separate file, developers can efficiently manage transparency settings and effortlessly enhance visual aesthetics, fostering a clean and modular design in JavaFX applications.
Do not forget to include a CSS file in your JavaFX application.
For the example source code below, styles.css
, you could include the following CSS rules.
.transparent-button {
-fx-background-color: transparent;
-fx-text-fill: black;
-fx-border-color: black;
-fx-border-width: 2;
}
Explanation of the CSS rules:
CSS Rule | Explanation |
---|---|
-fx-background-color: transparent; |
Sets the background color to transparent. |
-fx-text-fill: COLOR; |
Sets the text color to the color stated. |
-fx-border-color: COLOR; |
Sets the border color to the color stated. |
-fx-border-width: 2; |
Sets the width border to 2 pixels. |
We will explore how to apply CSS styling to create a transparent background with a black-bordered button, all within a white background StackPane
layout.
import java.util.Objects;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class transparent extends Application {
@Override
public void start(Stage stage) {
// Create a layout pane
StackPane layout = new StackPane();
// Create a button with black text and border
Button transparentButton = new Button("Click me!");
transparentButton.getStyleClass().add("transparent-button");
// Add the button to the layout
layout.getChildren().add(transparentButton);
// Create a scene with a white background
Scene scene = new Scene(layout, 300, 200);
scene.getStylesheets().add(
Objects.requireNonNull(transparent.class.getResource("styles.css")).toExternalForm());
// Set the scene for the stage
stage.setScene(scene);
// Set the stage title
stage.setTitle("Transparent Background CSS Example");
// Show the stage
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this JavaFX example, the process of crafting a transparent button with black text and borders, utilizing CSS styling, is methodically outlined.
Commencing with standard import statements and class declaration, the start
method initializes a StackPane
layout named layout
. A button, transparentButton
, is created and added to the layout.
CSS styling is applied through the addition of a custom style class, transparent-button
. The scene
is then established, associating it with the layout.
External CSS is linked via the styles.css
file, which contains styling rules.
The transparent.class.getResource("styles.css")
part fetches the resource location of the CSS file associated with the transparent
class.
The Objects.requireNonNull()
ensures that the resource is not null.
Finally, toExternalForm()
converts the resource URL to an external form that can be used to link the stylesheet to the scene.
The scene
is set for the stage
, titled accordingly, and the stage
is displayed using the show
method.
This systematic approach ensures the creation of a visually appealing and transparent button in JavaFX.
Output:
Upon running this JavaFX application, a stage
will appear with a button labeled Click me!
.
The button has a transparent background, black text, and a black border, as specified in the linked styles.css
file.
The use of external CSS allows for a clean separation of styling and Java code, making it easy to customize the appearance of UI elements.
This example demonstrates the flexibility and modularity offered by CSS styling in JavaFX, enabling developers to create visually appealing and customizable user interfaces.
Remember that if you are encountering a Resource not found
warning for your CSS file, it indicates that the application is unable to locate the specified stylesheet.
Ensure that your CSS file is in the correct location relative to your Java source files. It should be in the same directory or in a subdirectory.
If it’s in a subdirectory, make sure to adjust the file path accordingly.
Create a Transparent Stage
Background
Making a JavaFX stage
transparent is crucial for creating modern and visually appealing user interfaces.
This transparency allows developers to overlay UI components seamlessly, introduce creative design elements, and integrate applications seamlessly with the desktop environment. Transparent stages
provide a sleek and immersive user experience, enhancing the overall aesthetics and usability of JavaFX applications.
Example:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import javafx.stage.StageStyle;
public class transparent extends Application {
@Override
public void start(Stage stage) {
// Create a layout pane
StackPane layout = new StackPane();
// Create a scene with a transparent background
Scene scene = new Scene(layout, 300, 200);
Text text = new Text("The Stage is Transparent");
text.setFont(new Font(20));
layout.getChildren().add(text);
// Set the stage style to transparent
stage.initStyle(StageStyle.TRANSPARENT);
// Set the scene for the stage
stage.setScene(scene);
// Set the stage title
stage.setTitle("Transparent Stage Example");
// Show the stage
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In this JavaFX code, the process of creating a transparent stage
with a text message is systematically outlined. Commencing with standard import statements, the transparent
class extends the Application
class, a prerequisite for JavaFX development.
The overridden start
method, taking a Stage
parameter, initializes a StackPane
layout named layout
as the root for the scene. A scene
, associated with the layout and displaying a text message is set to specific dimensions.
The pivotal step involves invoking the initStyle
method to designate the stage style as StageStyle.TRANSPARENT
, rendering the entire stage
, including the title bar, transparent.
Subsequently, the scene
is set for the stage
, its title is defined, and the stage is displayed using the show
method. This methodical approach ensures the creation of a transparent stage
seamlessly integrated with the JavaFX application, providing a visually appealing user interface.
Output:
The window was positioned against a black background to illustrate that the stage
is transparent while the scene
is not.
Upon running this JavaFX application, a stage
will appear with a transparent background, and the message The Stage is Transparent
will be displayed in the center. The text is set in a larger font size, enhancing visibility.
The transparency of the stage
allows for a clean and modern visual effect, and this technique is particularly useful for creating overlay effects or unique UI designs.
The concept of setting the stage
style to transparent is effectively demonstrated through this example, showcasing the versatility of JavaFX in creating visually appealing applications.
Create a Transparent Scene
and Stage
Background
In JavaFX, achieving transparency in both the stage
and scene
is a powerful technique for crafting modern and visually engaging user interfaces.
This capability allows developers to create sleek designs, overlay effects, or seamlessly integrate their applications with the user’s desktop environment.
In this section, we will explore how to make both the stage
and scene
transparent and display a simple text message, The stage and scene are transparent!
.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import javafx.stage.StageStyle;
public class transparent extends Application {
public void start(Stage PrimaryStage) {
PrimaryStage.initStyle(
StageStyle.TRANSPARENT); // Set the initial style to the stage to transparent
Text txt = new Text("Scene and Stage are \ntransparent..."); // Creating a text element.
txt.setFont(new Font(30)); // Set the font size to 30 for the text.
txt.setFill(Color.WHITE);
VBox vbox = new VBox(); // Create a Vbox
vbox.getChildren().add(txt); // Add the text to the Vbox
Scene scene = new Scene(vbox, 400, 250); // Create a scene with Vbox and dimension
scene.setFill(null); // Set the scene to no fill
PrimaryStage.setScene(scene); // Add the scene to stage
PrimaryStage.show(); // Visualize the stage
}
public static void main(String[] args) {
launch(args); // Launch the application
}
}
This JavaFX code systematically achieves transparency in both the stage
and scene
.
Starting with standard import statements, the transparent
class extends Application
, necessary for JavaFX applications. The overridden start
method sets the stage
’s style to transparent using initStyle
.
A text element is then created with a white font and added to a vertical layout (VBox
). A scene
is formed with this layout, and its fill is set to null, ensuring scene
transparency.
The setScene
method associates the scene
with the stage
, and finally, the show
method displays the transparent stage
, effectively illustrating transparency in both the stage
and scene
.
Output:
The window was positioned against a black background to illustrate that the scene
and stage
are both transparent.
Upon running this JavaFX application, a stage will appear with a transparent background. The message Scene and Stage are transparent...
will be displayed in the center of the stage
, using a white color and a larger font size of 30.
The transparency of both the stage
and the scene
is achieved, providing a sleek and modern appearance. This technique is beneficial for creating visually engaging UIs or when integration with the desktop environment is desired.
The output demonstrates the effectiveness of setting the stage
and scene
to be transparent in JavaFX.
Conclusion
In conclusion, employing transparent backgrounds through external CSS files and utilizing features like -fx-background-color: transparent
, making the stage
transparent, and achieving transparency in both scene
and stage
in JavaFX is essential for enhancing visual aesthetics and creating modern, seamless user interfaces.
External CSS files promote code organization and ease of customization, allowing developers to efficiently manage transparency settings.
Making the stage
and scene
transparent offers flexibility in design, enabling overlay effects and integration with the desktop environment.
These practices collectively contribute to creating visually appealing and user-friendly JavaFX applications.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn