How to Show a JavaFX Popup in Java
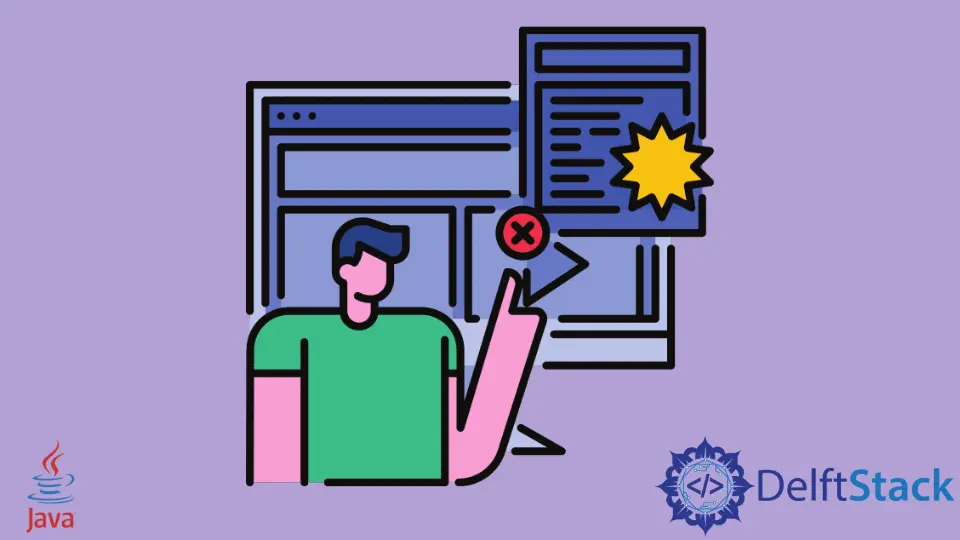
In this article, we will see how we can use one of the components of the JavaFX toolkit called popup. As the name suggests, the popup component shows a simple popup when executed using the GUI features of JavaFX.
This article provides a comprehensive overview of popups with text, delves into their implementation in JavaFX, and outlines best practices for creating effective and user-friendly JavaFX popups.
Popup With a Text
In Java, a popup with text typically refers to a small, transient window that appears temporarily on the screen, displaying text-based information or messages. This UI element is commonly used to provide brief notifications, alerts, or additional information without obstructing the main application window.
Popups with text can be created using various Java GUI frameworks, such as JavaFX or Swing, using dedicated classes like Popup
or JOptionPane
. These popups are useful for enhancing user experience by delivering concise and focused messages in response to specific events or user actions.
JavaFX Popups With the Popup
Class
JavaFX introduces the Popup
class to facilitate the creation of lightweight, transient windows known as popups. These popups serve as a practical solution for displaying supplementary information, notifications, or user prompts without disrupting the main application flow.
By encapsulating the complexities of window management, the Popup
class simplifies the process of generating and positioning these informative overlays. Its versatility allows developers to enhance user experience by presenting contextual information at the right moment, ensuring a seamless and unobtrusive interaction within JavaFX applications.
The Popup
class thus contributes to creating more intuitive and user-friendly graphical user interfaces in JavaFX.
Let’s dive into a complete working example that showcases the use of the Popup
class in a JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Popup;
import javafx.stage.Stage;
public class PopupExample extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("JavaFX Popup Example");
Button showPopupButton = new Button("Show Popup");
showPopupButton.setOnAction(event -> showPopup(showPopupButton));
StackPane root = new StackPane();
root.getChildren().add(showPopupButton);
primaryStage.setScene(new Scene(root, 300, 200));
primaryStage.show();
}
private void showPopup(Button showPopupButton) {
Popup popup = new Popup();
popup.getContent().add(new javafx.scene.control.Label("Hello, this is a Popup!"));
popup.show(showPopupButton.getScene().getWindow());
}
public static void main(String[] args) {
launch(args);
}
}
Now, let’s delve into the details of the code and understand how each component contributes to the creation and display of a popup in a JavaFX application.
The code initiates the configuration of a button labeled Show Popup
and associates an event handler with it. Upon clicking, the event handler, represented by the showPopup
method, is triggered.
The showPopup
method plays a crucial role in this process. It is responsible for instantiating the Popup
class, specifying the content of the popup as a label with the message Hello, this is a Popup!
.
The method then utilizes the show
method to display the popup, positioning it relative to the window that contains the showPopupButton
.
Proceeding to the primary stage configuration, this section tailors the primary stage by defining its scene with a root layout of StackPane
and setting its dimensions to 300x200 pixels. Subsequently, the primary stage is presented to the user.
Lastly, the main
method serves as the entry point for the JavaFX application. It invokes the launch
method, initiating the execution of the application.
Output:
(Popup Before)
(Popup After)
Upon running the application, a window titled JavaFX Popup Example
will appear. Clicking the Show Popup
button triggers the display of a popup with the message Hello, this is a Popup!
.
The popup appears in a position relative to the Show Popup
button, providing a simple yet effective way to present dynamic information to the user. The Popup
class empowers developers to enhance the interactivity of JavaFX applications by incorporating responsive and visually appealing popups.
Best Practices for JavaFX Popup
Consistent Styling
Ensure that the appearance of your popups aligns with the overall visual style of your JavaFX application. Consistency in styling helps maintain a cohesive user interface.
Appropriate Triggers
Use popups judiciously and tie their appearance to relevant user actions or events. Popups should appear in response to specific triggers, providing contextually meaningful information.
Clear and Concise Content
Keep the content of your popups clear, concise, and focused. Use succinct language to convey the intended message, avoiding unnecessary verbosity.
Responsive Sizing
Dynamically adjust the size of the popup based on its content. This ensures that the popup accommodates the information without being excessively large or cramped.
Mindful Placement
Carefully choose the placement of your popups on the screen. Position them in a way that avoids obscuring critical elements and provides a seamless user experience.
Appropriate Lifespan
Set an appropriate lifespan for your popups. They should appear long enough for users to read the content but not linger unnecessarily, preventing interruptions.
Consistent Interaction
Maintain consistency in how users interact with popups. If popups require user input, ensure that the interaction follows established patterns within your application.
Graceful Fading
Consider incorporating subtle animations or fading effects when showing or hiding popups. This adds a touch of elegance and helps avoid abrupt transitions that might disrupt the user’s flow.
Thematic Branding
If applicable, integrate thematic branding elements into your popups. This helps reinforce your application’s identity and provides a visually cohesive experience.
Testing Across Resolutions
Test the behavior of popups across different screen resolutions and sizes. Ensure that popups are responsive and function as expected on various devices.
Accessibility Considerations
Keep accessibility in mind when designing popups. Ensure that the content is readable, and provide alternatives for users who may rely on assistive technologies.
Memory Management
Be mindful of memory usage, especially if your application frequently generates popups. Properly manage the lifecycle of popups to prevent memory leaks.
User-Friendly Closures
Allow users to close popups easily, either by providing a close button or allowing them to click outside the popup. Ensure that closure mechanisms are intuitive and user-friendly.
Logging and Error Handling
Implement robust logging and error-handling mechanisms for scenarios where popups may fail to appear or encounter issues. This facilitates troubleshooting during development and maintenance.
Documentation
Document the usage and behavior of your popups, making it easier for other developers to understand and work with your code. Clear documentation contributes to a smoother collaborative development process.
Conclusion
Understanding the concept of popups with text is fundamental for creating informative and user-friendly interfaces. In the realm of JavaFX, leveraging the Popup
class provides a powerful mechanism for seamlessly implementing popups.
This article has explored the essential aspects of JavaFX popups. By adhering to best practices, developers can ensure that JavaFX popups are not only effective in conveying messages but also contribute to a cohesive and engaging user experience.
Employing clear content, thoughtful design, and appropriate triggers, developers can harness the potential of popups to deliver concise and relevant information, enhancing the overall usability of JavaFX applications.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn