How to Clear the Canvas in JavaFX
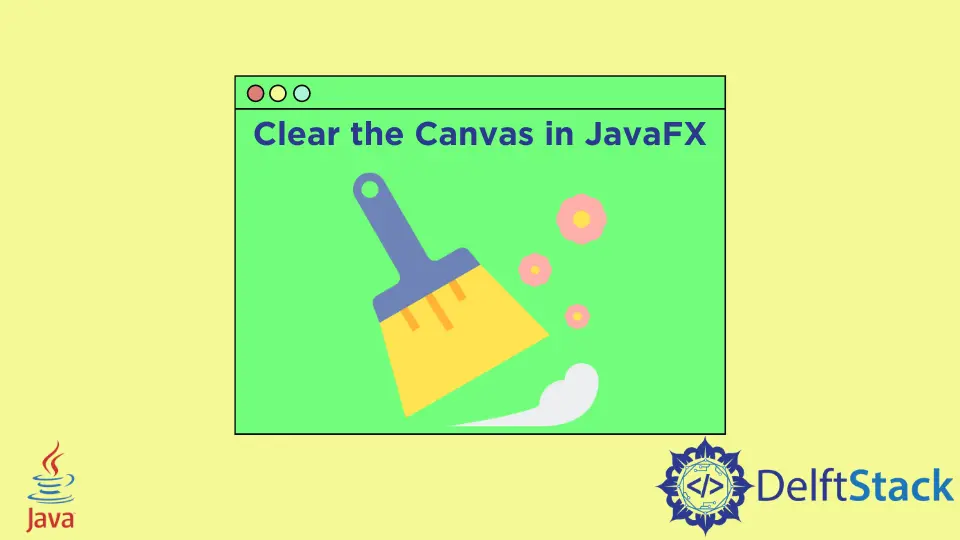
In JavaFX, the canvas is something like the image that draws various shapes and components using a set of graphics commands. This node is constructed with the necessary height and weight.
Using JavaFX canvas, you can create a UI component on which you can draw various shapes like rectangles, ovals, circles, etc., on screen. That special UI component is called canvas.
But sometimes, we need to remove or modify our drawing on canvas.
In this article, we will see how we can remove or clear a canvas. We also discuss this topic with necessary codes and explanations to make it easier to understand.
Clear the Canvas in JavaFX
A method named clearRect()
allows us to remove a specific component or clear the canvas. Let’s discuss it in detail.
To create an oval on the canvas, we can use the below code,
Diameter = Math.max(Width,
Height); // Create a mathematical calculation for the ovel with necessary height an weight
if (filledColor == null)
g.drawOval(x, y, Width, Height); // Draw the oval without fill it with color
else
g.fillOval(x, y, Width, Height); // Draw the oval and fill it with color
break;
After including this code on your program, you will see an oval drawn on your canvas. To remove the oval from the canvas or to clear the canvas, we can use the below code.
g.clearRect(0, 0, canvas.getWidth(), canvas.getHeight());
The code shared above will remove your drawing and clear the screen.
The general format of this method is:
clearRect( X_Position, Y_Position, Canvas_Height, Canvas_Width )
You can include this as an action of a button that will only use for clearing the canvas.
Below is an example of a simple canvas with this method
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
public class FxCanvas extends Application {
public static void main(String[] args) {
Application.launch(args); // Launch the application
}
@Override
public void start(Stage PrimaryStage) {
Canvas canvas = new Canvas(500, 200); // Create the Canvas
GraphicsContext g = canvas.getGraphicsContext2D(); // Create a 2D graphics context on the canvas
g.setLineWidth(3.0); // Set line width
g.setFill(Color.RED); // Set fill color
g.fillRoundRect(50, 50, 300, 100, 10, 10); // Draw a rounded Rectangle on the canvas
g.clearRect(80, 80, 140, 50); // Clear the rectangular area from the canvas
Pane pn = new Pane(); // Create a Pane
// Provide necessary styles
pn.setStyle(
"-fx-padding: 10; -fx-border-style: solid inside; -fx-border-width: 2; -fx-border-insets: 5; -fx-border-radius: 5; -fx-border-color: blue;");
pn.getChildren().add(canvas); // Add the canvas to the Pane
Scene scene = new Scene(pn); // Create a Scene
PrimaryStage.setScene(scene); // Add the Scene to the Stage
PrimaryStage.setTitle("Clearing Canvas area"); // Set the title of the application
PrimaryStage.show(); // Display the Stage
}
}
After compiling the above example code and running it in your environment, you will get the below output.
Output:
Remember, if your IDE doesn’t support the automatic inclusion of Libraries and Packages. Then you may need to include these necessary Libraries and Packages before compiling them manually.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn