How to Add a Background Image in JavaFX
- Add a JavaFX Background Image Using CSS
-
Add a JavaFX Background Image Using
BackgroundImage
in Java - Summary
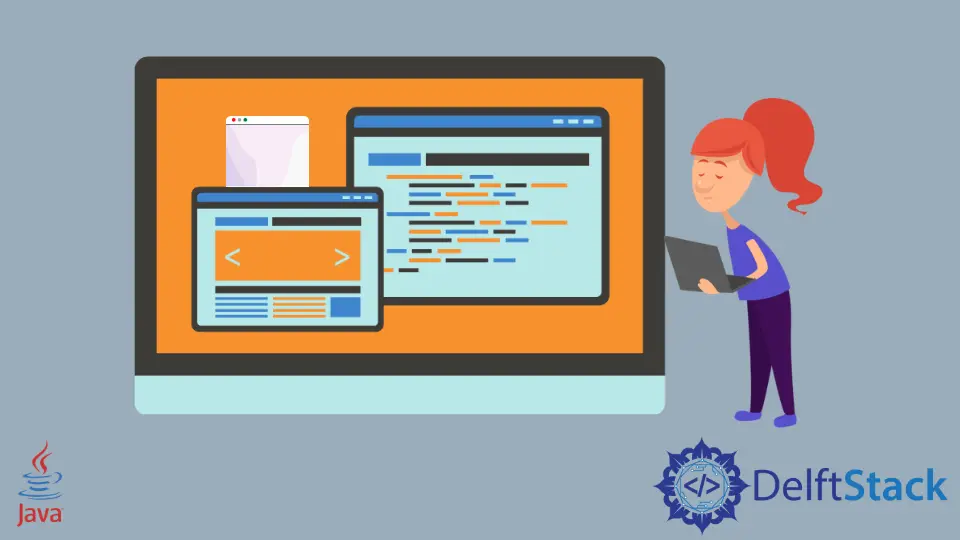
This tutorial introduces how to add background images in the JavaFX application. You can also follow the example codes we included to help you understand the topic.
The basic JavaFX application contains a primary stage, a scene, and individual nodes. The scene organizes the roots in a tree format, and the root of this tree is called the Root Node
.
Our class must extend the Application
class of the javafx.application
to create a JavaFX application. Then, we need to override the start()
method.
Here, we will show you how to add an image to the background of a JavaFX application. There are two ways to do this process: using CSS and using the BackgroundImage
class.
Add a JavaFX Background Image Using CSS
CSS is an abbreviation for Cascading Style Sheets and is used for styling web pages. Additionally, CSS can also be used to style JavaFX applications. We will use the following CSS rules to set and style the background image. You can add more rules according to your needs.
-fx-background-image: url('image-url');
-fx-background-repeat: no-repeat;
-fx-background-size: 500 500;
-fx-background-position: center center;
We can use inline CSS rules with the help of the setStyle()
method on the root node. Inline CSS is great if we just want to add a few rules. The complete JavaFX code is shown below.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 650, 650);
root.setStyle(
"-fx-background-image: url('https://cdn.app.compendium.com/uploads/user/e7c690e8-6ff9-102a-ac6d-e4aebca50425/ed5569e8-c0dd-458c-8450-cde6300093bd/File/a5023b0f0fb67f59176a0499af9021ed/java_horz_clr.png'); -fx-background-repeat: no-repeat; -fx-background-size: 500 500; -fx-background-position: center center;");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Inline CSS can become a bit cumbersome and difficult to understand if we have a lot of rules. Instead, we can create a separate CSS file and add these rules to that file. The contents of the CSS file are shown below.
#stack-pane{
-fx-background-image: url("https://cdn.app.compendium.com/uploads/user/e7c690e8-6ff9-102a-ac6d-e4aebca50425/ed5569e8-c0dd-458c-8450-cde6300093bd/File/a5023b0f0fb67f59176a0499af9021ed/java_horz_clr.png");
-fx-background-repeat: no-repeat;
-fx-background-size: 500 500;
-fx-background-position: center center;
}
We have used an ID selector in the CSS file, so we must set the same ID for the root of our JavaFX application. This process can be done by using the setId()
property.
We can simply add a reference to this CSS file, and the rules will be applied to our application. We will use the getStylesheets()
and add()
methods on the application scene to use the CSS file. The complete code for this is shown below.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
root.setId("stack-pane");
Scene scene = new Scene(root, 650, 650);
scene.getStylesheets().add(String.valueOf(this.getClass().getResource("sample.css")));
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Add a JavaFX Background Image Using BackgroundImage
in Java
JavaFX provides a BackgroundImage
class, a convenient option to use if we do not want to add CSS. The constructor of this class takes an Image
class object and other background image properties. The signature of the constructor of this class is shown below.
BackgroundImage(Image img, BackgroundRepeat repeatXAxis, BackgroundRepeat repeatYAxis,
BackgroundPosition pos, BackgroundSize size)
We will use the default position and size, and the image should not be repeated. We need to use the BackgroundImage
object to create a Background
class instance. Finally, we can use the setBackground()
on the root node to set the image on the background. The complete code for this is shown below.
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.layout.*;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
StackPane root = new StackPane();
Scene scene = new Scene(root, 650, 650);
Image img = new Image(
"https://cdn.app.compendium.com/uploads/user/e7c690e8-6ff9-102a-ac6d-e4aebca50425/ed5569e8-c0dd-458c-8450-cde6300093bd/File/a5023b0f0fb67f59176a0499af9021ed/java_horz_clr.png");
BackgroundImage bImg = new BackgroundImage(img, BackgroundRepeat.NO_REPEAT,
BackgroundRepeat.NO_REPEAT, BackgroundPosition.DEFAULT, BackgroundSize.DEFAULT);
Background bGround = new Background(bImg);
root.setBackground(bGround);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Summary
JavaFX may be outdated and replaced by other newer technologies, but it can still be used for various purposes. We can set the background image in a JavaFX application by using CSS rules. We can also apply CSS directly to the nodes by using the setStyle()
method.
If we have more rules, then we can also use an external CSS file. JavaFX also provides a BackgroundImage
class to set the background by using just plain Java easily.