How to Fix Java.Net.ConnectException: Connection Timed Out
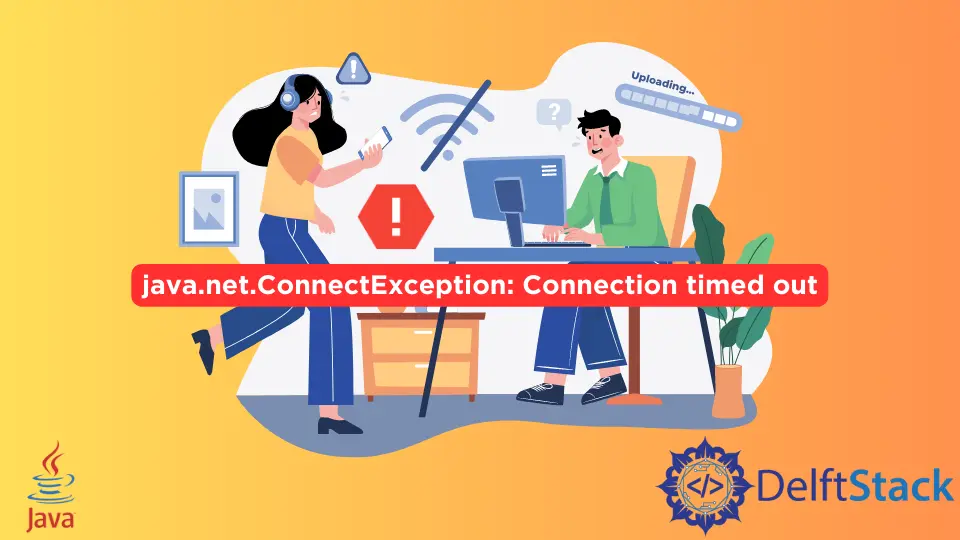
The java.net
package has many functions that can be used to make connections with any server on the internet and can be thus used to make rest calls like GET
or POST
.
This tutorial will focus on using this package to make basic network calls and what errors one can face and solve.
Make Network Calls With java.net
in Java
Making network calls is one of the most important things that Java developers face daily. Correctly making network calls can make the application more robust and give a good user experience.
The java.net
package has many functions associated with making network calls.
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
class Main {
public static void main(String args[]) throws Exception {
HttpURLConnection.setFollowRedirects(true); // Defaults to true
String url = "https://www.delftstack.com/howto/java/";
URL request_url = new URL(url);
HttpURLConnection http_conn = (HttpURLConnection) request_url.openConnection();
http_conn.setConnectTimeout(100000);
http_conn.setReadTimeout(100000);
http_conn.setInstanceFollowRedirects(true);
System.out.println(String.valueOf(http_conn.getResponseCode()));
}
}
The above code segment makes a POST
request to a sample REST API server and returns the response.
Error in the java.net
Package in Java
There may be a Connection Timed Out
problem while making network calls in Java. Fixing those issues is sometimes similar to why a certain URL would not open up on a browser.
They can be due to:
- The IP/domain of the URL is incorrect.
- The server corresponding to the IP/domain is down.
- A firewall may have blocked the requests, or the internet is behind a proxy.
- The server may have blocklist the IP.
For example:
import java.io.*;
import java.net.URL;
import java.net.URLConnection;
class Main {
public static void main(String args[]) throws Exception {
URLConnection urlConnection = null;
OutputStream outputStream = null;
URL url = new URL("http://google.com");
urlConnection = url.openConnection();
urlConnection.setConnectTimeout(1);
}
}
Output:
Exception in thread "main" java.net.SocketTimeoutException: Connect timed out
at java.base/java.net.Socket.connect(Socket.java:648)
at java.base/sun.net.NetworkClient.doConnect(NetworkClient.java:177)
at java.base/sun.net.www.http.HttpClient.openServer(HttpClient.java:474)
at java.base/sun.net.www.http.HttpClient.openServer(HttpClient.java:569)
at java.base/sun.net.www.http.HttpClient.<init>(HttpClient.java:242)
...
Thus the above exception is thrown when an appropriate connection cannot be opened to the socket or the URL, and the port combination or the timeout is insufficient to connect to the URL, which in this case is restricted by urlConnection.setConnectTimeout(1);
.
One can try the following to avoid the Connection Timed Out
error in Java.
- Setting the content length via
setFixedLengthStreamingMode()
. - Making retries to the connection in case the URL connection fails in the first attempt.
- Increasing the Connection Limit to a higher amount by the
setConnectTimeout
function.