String Matches Regex in Java
-
Use the
matches()
Method to Match String in Java -
Use the
regionMatches()
Method to Match String in Java
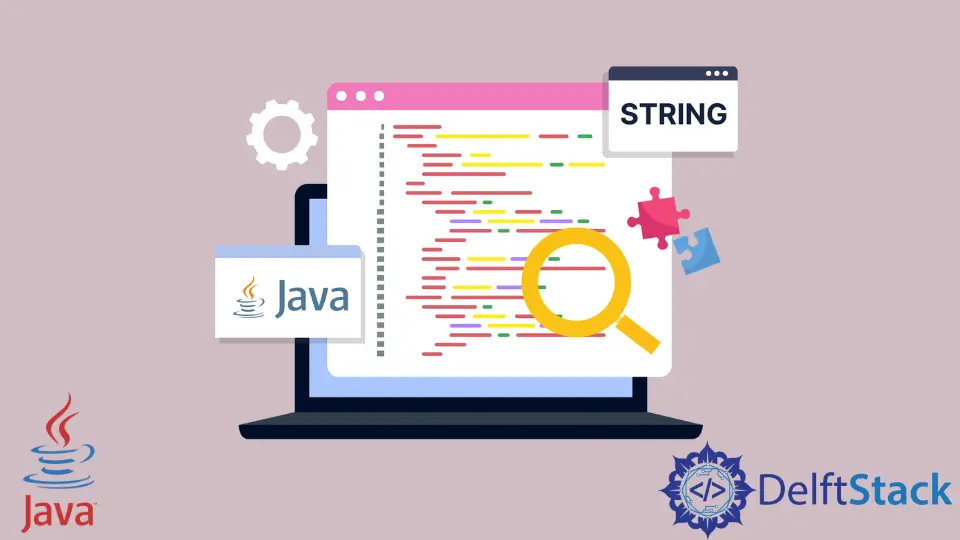
The String
class is one of the most used classes in Java. It provides many methods to perform various operations, and
In this article, we will be talking about the methods called matches()
and regionMatches()
of the String
class.
Use the matches()
Method to Match String in Java
The matches()
method matches a string with the value passed in the function. The value to pass in the function as argument should be a regular expression.
The function Pattern.matches()
returns the same result as String.matches()
.
In the example below, we create three String
variables, and we use regex (short for Regular Expression) to find out if all the characters in the String
are in lowercase and valid alphabets from a to z.
The first print
statement calls the matches()
method and passes [a-z]+
, the regex to match if the characters are lower case alphabets. The first statement outputs true
because the String exampleStr1
contains the characters matching the regex.
The second statement checks the exampleStr2
with the same regex and returns false
because the String’s first character is upper case.
The last print
statement also returns false
that checks the exampleStr3
as non-alphabetical characters exist.
public class ExampleClass1 {
public static void main(String[] args) {
String exampleStr1 = "guardian";
String exampleStr2 = "Guardian";
String exampleStr3 = "[abc]";
System.out.println("First String: " + exampleStr1.matches("[a-z]+"));
System.out.println("Second String: " + exampleStr2.matches("[a-z]+"));
System.out.println("Third String: " + exampleStr3.matches("[a-z]+"));
}
}
Output:
First String: true
Second String: false
Third String: false
Use the regionMatches()
Method to Match String in Java
Another method to match a string using a regex is regionMatches()
, which matches regions of two strings. The example has two Strings, the first is a five-word statement, and the second String is a single word.
Using the regionMatches()
method, we match if the word production
contains the substring duct
. We pass four arguments in the regionMatches()
function to perform this.
The first argument is the start position of the word from where to start scanning; in our case, our word is at 19th position, so we set it as starting position.
The second argument is the exampleStr2
input string we want to match.
We pass the starting position of exampleStr2
as the third argument, and the last argument specifies the number of characters to match.
public class ExampleClass1 {
public static void main(String[] args) {
String exampleStr1 = "this site is in production";
String exampleStr2 = "duct";
System.out.println(exampleStr1.regionMatches(19, exampleStr2, 0, 4));
}
}
Output:
true
The above code only matches the substring if the matching String is of the same case. We pass another argument in the regionMatches()
that ignores the case of the characters.
public class ExampleClass1 {
public static void main(String[] args) {
String exampleStr1 = "this site is in production";
String exampleStr2 = "DUCT";
System.out.println(exampleStr1.regionMatches(true, 19, exampleStr2, 0, 4));
}
}
Output:
true
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn