Regular Expression \\s in Java
-
the Regular Expression
\s
in Java -
Expand
\s
With Quantifiers in Java -
Use
\s
in Regular Expressions in Java -
Escaping
\s
in Java - Conclusion
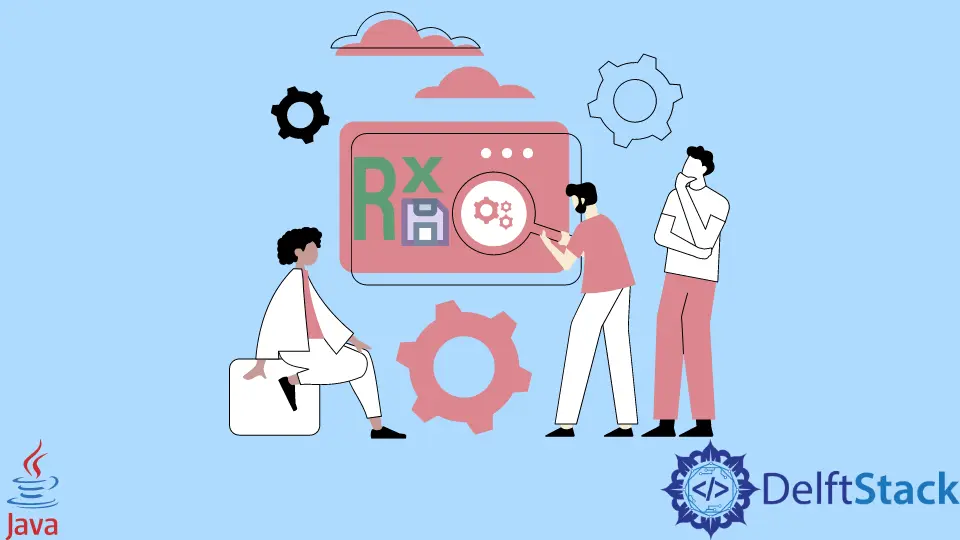
Regular expressions are a powerful tool in Java for pattern matching and manipulation of strings. Among the many metacharacters and special sequences used in regular expressions, \\s
holds a significant place.
In this article, we’ll delve deep into what \\s
means, its applications, and how it can be utilized effectively in Java programming.
the Regular Expression \s
in Java
Regular expressions are the sequence of characters used to develop a pattern for data. In Java regular expressions, \\s
is a predefined character class that represents any whitespace character.
Whitespace characters include spaces, tabs, and line breaks. This allows you to match and manipulate whitespace in strings, making it a versatile tool for tasks like tokenizing text or validating user input.
We use patterns sometimes when we search data in the text, and \\s
is used in those patterns when space is required.
We use double backslash because Java syntax does not support the single backslash. In reality, the syntax for a single whitespace character is \s
.
Here’s a simple example to demonstrate the use of \\s
:
import java.util.regex.*;
public class Main {
public static void main(String[] args) {
String text = "Hello World";
String[] words = text.split("\\s");
for (String word : words) {
System.out.println(word);
}
}
}
In this example, the string contains multiple spaces between "Hello"
and "World"
. The regular expression \\s+
matches one or more consecutive whitespace characters, effectively treating them as a single delimiter.
The output will be the same as before:
Hello
World
Use \s
in Regular Expressions in Java
\\s
can also be employed in more complex regular expressions for tasks like pattern matching, substitution, or validation.
For instance, let’s say you want to validate if a string contains only letters and spaces:
import java.util.regex.*;
public class Main {
public static void main(String[] args) {
String text = "Hello World";
boolean isValid = text.matches("[a-zA-Z\\s]+");
System.out.println(isValid); // Output: true
}
}