How to Use Regex in the String.contains() Method in Java
- Understanding String.contains() Method
- Using Regex with String.matches()
- Using Pattern and Matcher Classes
- Regex for Validating Input
- Conclusion
- FAQ
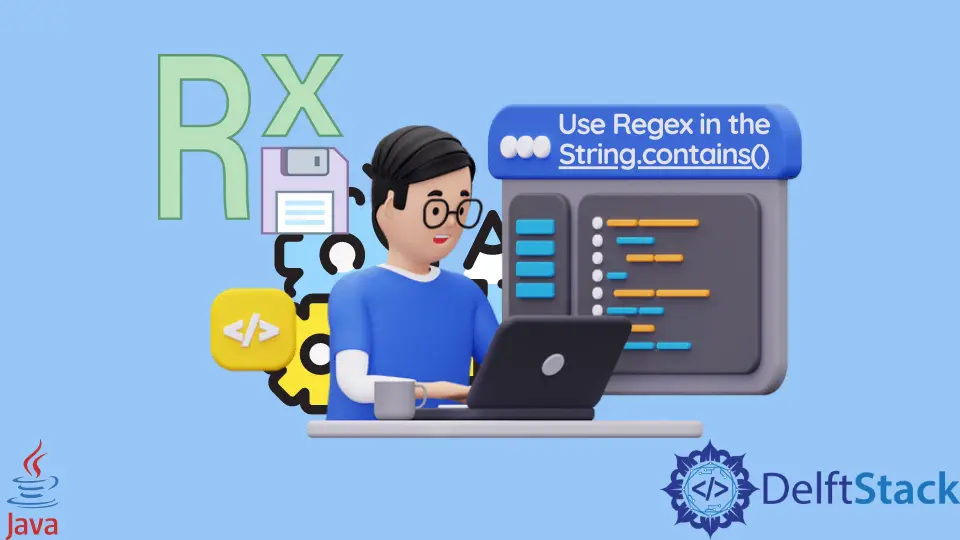
When working with strings in Java, the ability to search for specific patterns can be incredibly useful. While the String.contains()
method is a straightforward way to check for the presence of a substring, it lacks the power of regular expressions (regex). Regex allows for more complex pattern matching, enabling developers to perform sophisticated searches within strings.
In this article, we will explore how to effectively utilize regex in Java’s string handling, enhancing your programming toolkit. Whether you’re validating input, searching for specific patterns, or manipulating text, understanding how to combine regex with string methods can significantly improve your coding efficiency. Let’s dive into the world of regex in Java!
Understanding String.contains() Method
Before we dive into regex, it’s essential to understand the String.contains()
method. This method checks if a string contains a specified sequence of characters. The syntax is simple:
boolean contains(CharSequence sequence)
The method returns true
if the sequence is found, and false
otherwise. However, this method does not support regex, which limits its functionality for more complex string searches. If you’re looking for patterns rather than fixed sequences, regex is the way to go.
Using Regex with String.matches()
While String.contains()
does not support regex, you can achieve similar results with the String.matches()
method. This method checks if the entire string matches a regex pattern. To use it effectively, you’ll need to ensure that your regex pattern is well-defined.
Here’s a simple example:
String text = "Hello, World!";
String regex = ".*World.*";
boolean matches = text.matches(regex);
In this code, we define a string text
and a regex pattern regex
that checks if “World” exists within the string. The matches()
method returns true
because the pattern is found.
Output:
true
The matches()
method is powerful because it allows you to define complex patterns. The .*
in the regex pattern means “zero or more characters” before and after “World,” making it flexible. However, remember that matches()
checks the entire string against the regex. If you want to check for a substring, you might need to adjust your regex accordingly.
Using Pattern and Matcher Classes
For more advanced regex functionality in Java, you can use the Pattern
and Matcher
classes. This approach allows you to search for patterns within a string without needing to match the entire string.
Here’s how you can use these classes:
import java.util.regex.*;
String text = "Hello, World!";
String regex = "World";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
boolean found = matcher.find();
In this example, we create a Pattern
object with the regex “World” and a Matcher
object that checks the string text
. The find()
method returns true
if the pattern is found within the string.
Output:
true
Using Pattern
and Matcher
gives you more control over your regex operations. You can find multiple occurrences, replace text, and perform more complex searches. This method is particularly useful when you’re dealing with large texts or need to extract specific information based on patterns.
Regex for Validating Input
Regex is also invaluable for validating user input. For instance, if you want to ensure that a user enters a valid email address, you can use regex patterns to check the input format.
Here’s an example of how to validate an email address:
String email = "example@domain.com";
String regex = "^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$";
boolean isValidEmail = email.matches(regex);
In this code, we define a regex pattern for a basic email format and check if the email
string matches it.
Output:
true
The regex pattern used here checks for common email components, including letters, numbers, and symbols. While this pattern covers many typical email formats, remember that email validation can get quite complex. Adjust your regex as needed to match the specific criteria you want to enforce.
Conclusion
Incorporating regex into your Java string handling can significantly enhance your ability to search, validate, and manipulate text. While the String.contains()
method is useful for simple substring checks, utilizing methods like String.matches()
, Pattern
, and Matcher
opens up a world of possibilities for more complex string operations. By mastering regex, you can write more efficient and powerful Java applications that handle text with precision. So, start experimenting with regex patterns today, and take your Java programming skills to the next level!
FAQ
-
Can I use regex with String.contains() in Java?
You cannot use regex directly with String.contains(). Instead, consider using String.matches() or the Pattern and Matcher classes for regex operations. -
What is the difference between String.matches() and Pattern?
String.matches() checks if the entire string matches a regex pattern, while Pattern allows for more complex operations like finding, replacing, and splitting strings based on regex. -
How do I validate a phone number using regex in Java?
You can define a regex pattern for the phone number format and use String.matches() or the Matcher class to validate the input against that pattern. -
Are there any performance considerations when using regex in Java?
Yes, regex operations can be computationally expensive, especially with complex patterns. It’s essential to optimize your regex for performance when working with large datasets. -
Where can I learn more about regex in Java?
There are many online resources, including tutorials and documentation, that can help you learn regex in Java. Websites like Oracle’s Java documentation and regex101.com are excellent places to start.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn