How to Handle Regex Special Characters in Java
- Introduction to Regex Special Characters
- Common Methods and Techniques to Use Regex Special Characters
- Best Practices for Using Regex Special Characters
- Conclusion
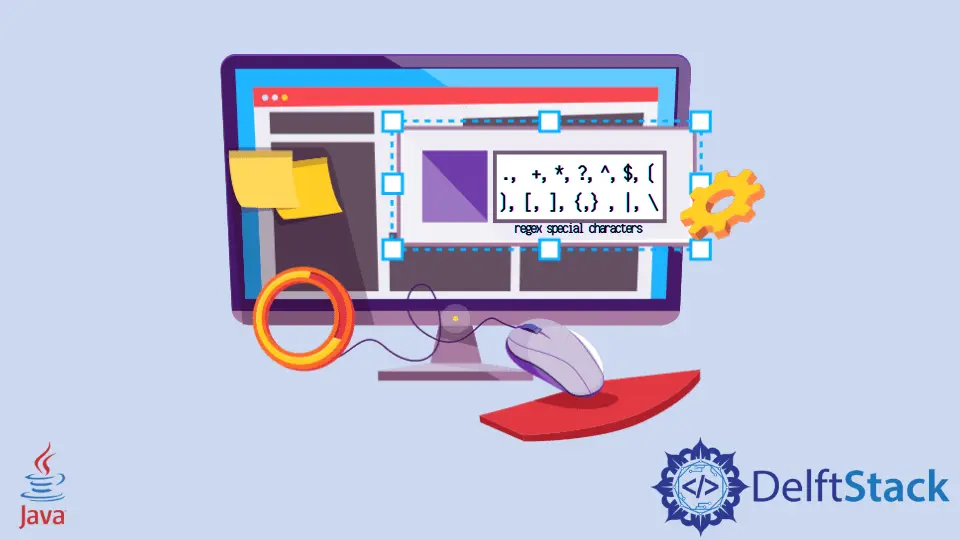
Regex (Regular Expression) is a useful tool for manipulating, searching, and processing text strings. It simplifies and reduces the number of lines in a program.
In this article, we will explore various methods of utilizing regex special characters in Java, including escaping with backslash, character classes, negation in character classes, the dot metacharacter, asterisk quantifier, plus quantifier, question mark quantifier, anchors, pipe alternation, and parentheses for grouping.
Introduction to Regex Special Characters
In Java programming, special characters play a pivotal role in the realm of regular expressions, providing a powerful mechanism for pattern matching within strings. These characters serve as building blocks for constructing intricate search patterns, enabling developers to define flexible and precise criteria for string manipulation.
Whether it’s validating user input, parsing complex data structures, or extracting information from text, the use of special characters in regular expressions empowers Java programmers to create efficient and versatile solutions for a wide range of string-processing tasks. Understanding and harnessing these special characters is essential for mastering the art of effective pattern matching in Java applications.
Common Methods and Techniques to Use Regex Special Characters
Regex is a type of textual syntax representing patterns for text matching. Regular expressions make use of special characters such as .
, +
, *
, ?
, ^
, $
, (
, )
, [
, ]
, {
, }
, |
, \
.
Characters in a regular expression (those in the string representing its pattern) are either metacharacters with a special meaning or regular characters with a literal meaning.
Metacharacter | Use | Example |
---|---|---|
^ |
Anchors the regex at the start of the line. | ^a matches a at the start of the string |
. |
Matches any single character except a newline. | a.[0-9] matches a string that has an a followed by a character and a digit |
[] |
Denotes a character class - matches any one of the characters inside the brackets. | [a-c] equals to either a or b or c , i.e., a|b|c also [abc] |
[^] |
Negates a character class - matches any character NOT inside the brackets. | [^abc] matches any character other than a , b , or c |
$ |
Anchors the regex at the end of the line. | ^abc$ matches a string that starts and ends with abc |
() |
Groups expressions together. | (ab)\1 matches abab |
* |
Matches 0 or more occurrences of the preceding character. | ab*c matches ac , abc , abbbc , etc. |
{m,n} |
Matches the preceding element at least m times and not more than n times | a{3,5} matches aaa , aaaa , aaaaa |
? |
Matches 0 or 1 occurrence of the preceding character. | ab?c matches ac , abc |
+ |
Matches 1 or more occurrences of the preceding character. | ab+c matches abc , abbc , abbbc , etc., but not ac |
| |
The choice operator. It matches either the expression before or expression after the operator | |
ab|def matches either ab or def |
\ |
Escapes a special character, allowing it to be treated as a literal. | common escape sequences like \n or newline, \t for tab |
These are just a subset of the special characters used in Java regular expressions. Depending on your specific needs, there might be additional special characters or combinations.
Feel free to adapt these examples to your specific needs or explore further with the rich set of features provided by the java.util.regex
package.
Understanding these methods is paramount for developers aiming to master the art of precise pattern matching in Java.
Let’s dive into a comprehensive example that incorporates all these methods:
import java.util.regex.*;
public class RegexSpecialCharactersExample {
public static void main(String[] args) {
// Sample input string
String input = "The colors: red, blue, and green.";
// Escaping with backslash
String regexBackslash = "red\\, blue"; // Matches "red, blue"
// Character classes
String regexCharClasses = "[rgb]"; // Matches any of 'r', 'g', 'b'
// Negation in character classes
String regexNegation = "[^aeiou]"; // Matches any non-vowel character
// Dot metacharacter
String regexDot = "b.."; // Matches "boo", "bee", etc.
// Asterisk quantifier
String regexAsterisk = "re*d"; // Matches "red", "reed", "reed", etc.
// Plus quantifier
String regexPlus = "bl+ue"; // Matches "blue", "bluee", "blueee", etc.
// Question mark quantifier
String regexQuestion = "colo?rs"; // Matches both "color" and "colours"
// Anchors
String regexAnchors =
"^The.*green\\.$"; // Matches lines starting with "The" and ending with "green."
// Pipe alternation
String regexAlternation = "red|blue"; // Matches either "red" or "blue"
// Parentheses for grouping
String regexGrouping = "(re)+"; // Matches "re", "rere", "rere", etc.
// Iterate through methods and print matches
applyRegexAndPrintMatches(input, regexBackslash);
applyRegexAndPrintMatches(input, regexCharClasses);
applyRegexAndPrintMatches(input, regexNegation);
applyRegexAndPrintMatches(input, regexDot);
applyRegexAndPrintMatches(input, regexAsterisk);
applyRegexAndPrintMatches(input, regexPlus);
applyRegexAndPrintMatches(input, regexQuestion);
applyRegexAndPrintMatches(input, regexAnchors);
applyRegexAndPrintMatches(input, regexAlternation);
applyRegexAndPrintMatches(input, regexGrouping);
}
// Method to apply regex and print matches
private static void applyRegexAndPrintMatches(String input, String regex) {
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(input);
System.out.println("Matches for \"" + regex + "\":");
while (matcher.find()) {
System.out.print(" " + matcher.group());
}
System.out.println();
}
}
In the exploration of various methods involving regex special characters in Java, several techniques were applied to create precise search patterns within strings.
The first method involved escaping special characters using backslashes, as demonstrated by the regex red\\, blue
. This approach ensures the exact matching of the literal sequence red, blue
.
Next, character classes were employed with the regex [rgb]
, allowing for the matching of any single character among r
, g
, or b
. The third method utilized negation in character classes, exemplified by the regex [^aeiou]
, which matches any non-vowel character.
The dot metacharacter (b..
) was employed to match any three-character sequence starting with b
. The asterisk quantifier (re*d
) and the plus quantifier (bl+ue
) were used to match variable occurrences of characters, showcasing patterns like red
, reed
, reeed
, and blue
, bluee
, blueee
, respectively.
The question mark quantifier was demonstrated with the regex colo?rs
, allowing for the matching of both color
and colours
. Anchors were introduced using the regex ^The.*green\\.$
, ensuring matches occurred only at the start of lines and at the end with green
.
Pipe alternation (red|blue
) facilitated the matching of either red
or blue
, offering a versatile approach. Lastly, parentheses for grouping were showcased in the regex (re)+
, allowing matches for patterns like re
, rere
, and rere
.
Each of these methods contributes to the flexibility and precision of regex patterns, providing developers with powerful tools for effective string pattern matching in Java.
The output will showcase the matches for each regex, demonstrating the effectiveness of these methods in handling various string patterns.
This comprehensive example illustrates how each method involving regex special characters contributes to accurate and versatile string pattern matching in Java. Understanding these techniques empowers developers to create robust and efficient regex patterns for diverse applications.
Best Practices for Using Regex Special Characters
Escape Special Characters
Always escape special characters using the backslash \
to ensure they are treated as literal characters in the regular expression pattern.
Use Character Classes Wisely
Utilize character classes ([ ]
) to represent a set of characters, making your regex more concise and expressive. Remember that the order of characters within the brackets does not matter.
Mindful of Metacharacters
Be aware of metacharacters like .
, *
, +
, ?
, and use them judiciously based on your matching requirements. Escaping when needed ensures precise interpretation.
Anchoring for Positioning
Employ ^
to anchor the regex at the start and $
to anchor at the end. This ensures that the pattern matches only at the specified positions within the input string.
Quantifiers for Repetition
Effectively use *
, +
, and ?
to denote repetition, but be cautious not to overuse them, as excessive greediness can lead to unexpected matches.
Grouping for Logical Organization
Leverage parentheses ()
for grouping and logical organization of expressions. This is essential when applying quantifiers or alternation to specific parts of the pattern.
Understand Alternation
Master the use of |
for alternation to match multiple patterns. Grouping with parentheses is often necessary when dealing with alternation.
Utilize Escape Sequences
Familiarize yourself with escape sequences like \d
, \w
, and \s
for digit, word character, and whitespace, respectively. These can simplify character class definitions.
Consider Precompiled Patterns
For improved performance in repetitive use, precompile your regex patterns using Pattern.compile()
. This minimizes pattern compilation overhead.
Document Your Regex Patterns
Provide clear and concise comments to document complex regex patterns, making them more understandable for both yourself and others who may read the code.
Test Rigorously
Thoroughly test your regex patterns with various input scenarios, including edge cases, to ensure they behave as expected and handle all possible cases.
Conclusion
Understanding and adeptly utilizing regex special characters in Java are integral skills for developers. The common methods and techniques explored, such as escaping with backslashes, character classes, negation, and quantifiers, offer a robust toolkit for crafting precise search patterns within strings.
Best practices involve careful consideration of special characters, utilizing escape sequences, and testing rigorously to ensure accurate pattern matching. Mastering these methods empowers developers to navigate the intricacies of string manipulation, enhancing the effectiveness and efficiency of Java applications.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn