Java 中的字串匹配正規表示式
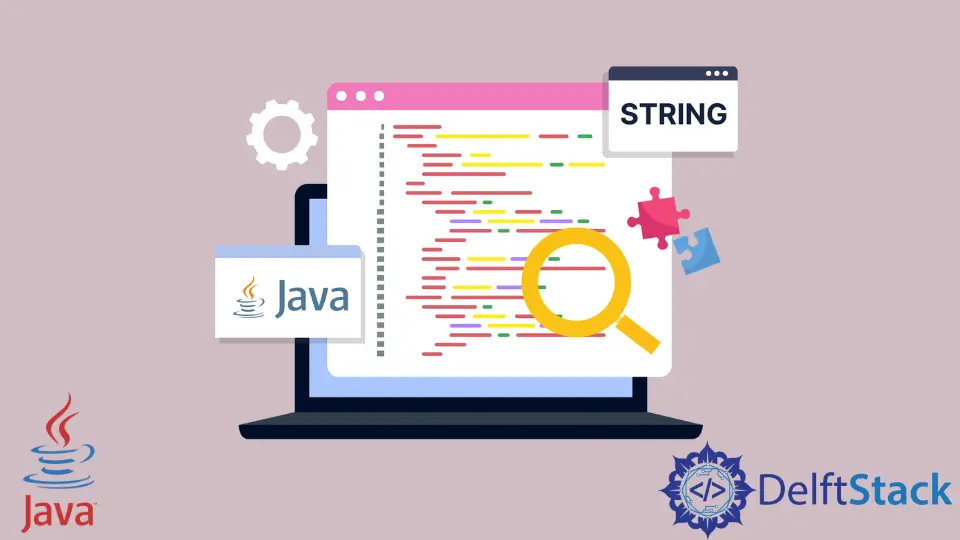
String
類是 Java 中最常用的類之一。它提供了許多方法來執行各種操作,在本文中,我們將討論 String
類的 matches()
和 regionMatches()
方法。
在 Java 中使用 matches()
方法匹配字串
matches()
方法將字串與函式中傳遞的值匹配。作為引數傳入函式的值應該是正規表示式。
Pattern.matches()
函式返回與 String.matches()
相同的結果。
在下面的示例中,我們建立了三個 String
變數,並使用 regex(正規表示式的縮寫)來確定 String
中的所有字元是否都是小寫且從 a 到 z 的有效字母。
第一個 print
語句呼叫 matches()
方法並傳遞 [a-z]+
,如果字元是小寫字母,則匹配的正規表示式。第一條語句輸出 true
,因為字串 exampleStr1
包含與正規表示式匹配的字元。
第二條語句使用相同的正規表示式檢查 exampleStr2
並返回 false
,因為字串的第一個字元是大寫的。
最後的 print
語句還返回 false
,檢查 exampleStr3
是否存在非字母字元。
public class ExampleClass1 {
public static void main(String[] args) {
String exampleStr1 = "guardian";
String exampleStr2 = "Guardian";
String exampleStr3 = "[abc]";
System.out.println("First String: " + exampleStr1.matches("[a-z]+"));
System.out.println("Second String: " + exampleStr2.matches("[a-z]+"));
System.out.println("Third String: " + exampleStr3.matches("[a-z]+"));
}
}
輸出:
First String: true
Second String: false
Third String: false
在 Java 中使用 regionMatches()
方法匹配字串
另一種使用正規表示式匹配字串的方法是 regionMatches()
,它匹配兩個字串的區域。該示例有兩個字串,第一個是五個單詞的語句,第二個字串是一個單詞。
使用 regionMatches()
方法,我們匹配單詞 production
是否包含子字串 duct
。我們在 regionMatches()
函式中傳遞四個引數來執行此操作。
第一個引數是單詞的起始位置,從哪裡開始掃描;在我們的例子中,我們的單詞在第 19 位,所以我們將它設定為起始位置。
第二個引數是我們想要匹配的 exampleStr2
輸入字串。
我們將 exampleStr2
的起始位置作為第三個引數傳遞,最後一個引數指定要匹配的字元數。
public class ExampleClass1 {
public static void main(String[] args) {
String exampleStr1 = "this site is in production";
String exampleStr2 = "duct";
System.out.println(exampleStr1.regionMatches(19, exampleStr2, 0, 4));
}
}
輸出:
true
上面的程式碼僅在匹配的字串大小寫相同時才匹配子字串。我們在 regionMatches()
中傳遞了另一個引數,它忽略了字元的大小寫。
public class ExampleClass1 {
public static void main(String[] args) {
String exampleStr1 = "this site is in production";
String exampleStr2 = "DUCT";
System.out.println(exampleStr1.regionMatches(true, 19, exampleStr2, 0, 4));
}
}
輸出:
true
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn