How to Split a Comma-Separated String in Java
-
Use the
Indexof()
andSubstring()
Method to Split Comma-Separated String in Java - Use the String List and Split Method to Split a Comma-Separated String in Java
- Use the Array List and Split Method to Comma Separate a String Value in Java
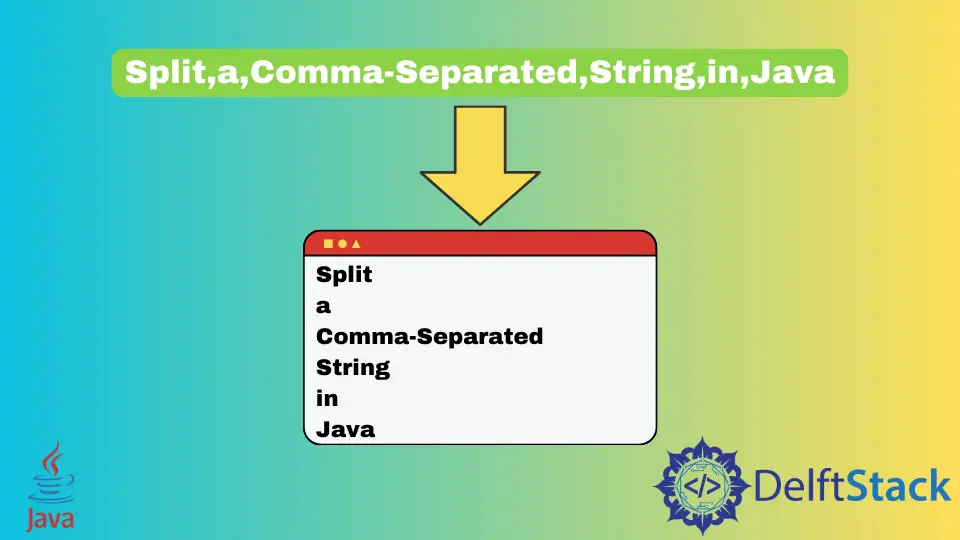
Java string
class is full of rich resources that you can use to create elementary programs to solve complicated problem statements.
This tutorial will show some cool ways to split a string from a comma-separated starting point or any division you might use in the string value set.
Also, we will use the IndexOf();
method for our first program and integrate Split()
with Java’s dynamic array and string classes.
Use the Indexof()
and Substring()
Method to Split Comma-Separated String in Java
Check out the string: String java = "Java, is, a, fun, programming, language!";
. This example is comma-separated, but this can be any character otherwise.
string
class.But, doing that will not aid your understanding of constructing logic as a newbie. The following core concepts are revised for you at the intermediate level.
Understand the indexof()
Method in Java
It returns the position of the first occurrence of the specified value in a user-specified string.
Parameters:
String |
String demoStr = "I, am, a, string"; |
Suppose the starting index is set to 0 , the indexOf() searches from "I" . |
fromIndex |
An int value specifying the index position to begin the search. |
It will let you play with index(s). |
char |
An int value that contains a particular character. |
Optional |
Returns: An int
value denotes the value’s first appearance index in the string, or -1
if it never occurred.
Importance of the substring()
Method in Java
It returns a string that is a substring of this string. It starts at the given point and ends with the character at the specified point.
We hope you have successfully understood the documentation of these methods. Let’s run our first program now.
Code:
// Example 1
public class UseIndexofAndSubString {
public static void main(String[] args) {
// Specified string to search comma from and then split it in a substring
String java = "Java, is, a, fun, programming, language!";
String comma = ","; // To search ',' from the first occurrence of the specified string
// Our starting index is 0 since we do not want to miss any occurrence in the
// given string value while the end index is also initialized
int start = 0, end;
// Now the indexOf will find the specified character which in this case is a
// comma (,)
// Note: The second parameter (start) determines that the method starts from the
// beginning, otherwise it will
// Basically, we will be able to locate the occurrence of (comma) from where we
// want it to be split
end = java.indexOf(comma, start);
// If the loop does not find (comma)
// We already mentioned the return type of indexOf in case if the given char
// never occurred, right? That is what the loop searches for!
while (end != -1) {
//// Substring will give us each occurrence until the loop is unable to find
//// comma
String split = java.substring(start, end);
System.out.println(split); // print each sub string from the given string
// Then add 1 to the end index of the first occurrence (search more until the
// condition is set false)
start = end + 1;
end = java.indexOf(comma, start);
}
// Since there is no comma after the last index of the string (language!), the
// loop could not find the end index; thus, it never printed the last word!
// We will get the last instance of the given string using substring and pass
// start position (Starts from L and ends at the !)
String split = java.substring(start);
// Brave, you got it all right!
System.out.println(split);
}
}
Output:
Java
is
a
fun
programming
language!
Suppose you want to change the comma
to a dollar $
sign.
All you need to do:
String java = "Using$ another$ Character$ to$ split$ from!";
String sepFrom = "$"; // To search from the specified string
Output:
Using
another
Character
to
split
from!
Use the String List and Split Method to Split a Comma-Separated String in Java
When we discussed the importance of elementary-level understanding, we talked about arrays.
The following program is easy. You do not need to find starting and ending positions, nor do you need to run a while
loop and compare whether the return value is -1.
Java’s string
and arrays
classes sum it all up in four lines of clean code. Meanwhile, you need to comprehend two required methods that will help you along the way.
the Arrays.aslist())
Function in Java
Parameters:
- The class of the array elements’ values.
- The user-specified array uses to store the list.
- It gives you a list view of the specified array you defined.
Syntax:
List<String> ArraySting = Arrays.asList(IwillBeSplit.split(","));
the Split()
Function in Java
It uses the two parameters in the split method that we as users specify as a regular expression and a limit parameter of 0
.
Therefore, it does not include the trailed empty strings in the output set.
- Parameter: Regular expression that serves as a separator.
- Returns: An array of strings generated by separating this string of the
regex expression.
Code:
// Example 3 using Lists' String with Split Method to Split String From a Given demarcation
import java.util.Arrays;
import java.util.List;
public class UseStingListWithSplitMthd {
public static void main(String[] args) {
String IwillBeSplit = "There,are,seven,days,!&#^*,in,a,weak";
// List is an interface in Java
List<String> ArraySting = Arrays.asList(IwillBeSplit.split(","));
// Using for each loop
ArraySting.forEach(System.out::println);
}
}
Output:
There
are
seven
days
!&#^*
in
a
weak
Suppose the string is:
String IwillBeSplit = "You%replaced%the%comma%with%a%percentage";
List<String> ArraySting = Arrays.asList(IwillBeSplit.split("%"));
We will get:
Output:
You
replaced
the
comma
with
a
percentage
Use the Array List and Split Method to Comma Separate a String Value in Java
The following code block should not confuse you. Since, you are already familiar with the Arrays.asList());
and the split()
.
Nevertheless, the one thing different here is the approach to the solution, which is using ArrayList<String>
instead of just List<String>
.
So, what is the difference?
It’s not that hard. The list
is an interface, while an ArrayList
is a Java collection class.
The former provides a static array, while the latter generates a dynamic array to store the elements.
Code:
// Example 2 using ArrayLists and split method
import java.util.ArrayList;
import java.util.Arrays;
public class ArrayListSplitMethod {
public static void main(String[] args) {
String ALstr = "So,many,ways,to,separate,a,string,from,a,specified,position";
ArrayList<String> Spliter = new ArrayList<>(Arrays.asList(ALstr.split(",")));
Spliter.forEach(System.out::println);
}
}
Output:
So
many
ways
to
separate
a
string
from
a
specified
position
Sarwan Soomro is a freelance software engineer and an expert technical writer who loves writing and coding. He has 5 years of web development and 3 years of professional writing experience, and an MSs in computer science. In addition, he has numerous professional qualifications in the cloud, database, desktop, and online technologies. And has developed multi-technology programming guides for beginners and published many tech articles.
LinkedIn