Java Singleton Class
- Singleton Class in Java
- Use Various Methods to Utilize Singleton Class in Java
- Java Singleton Class Using Lazy Initialization Approach
- Java Singleton Class Using Early Initialization Approach
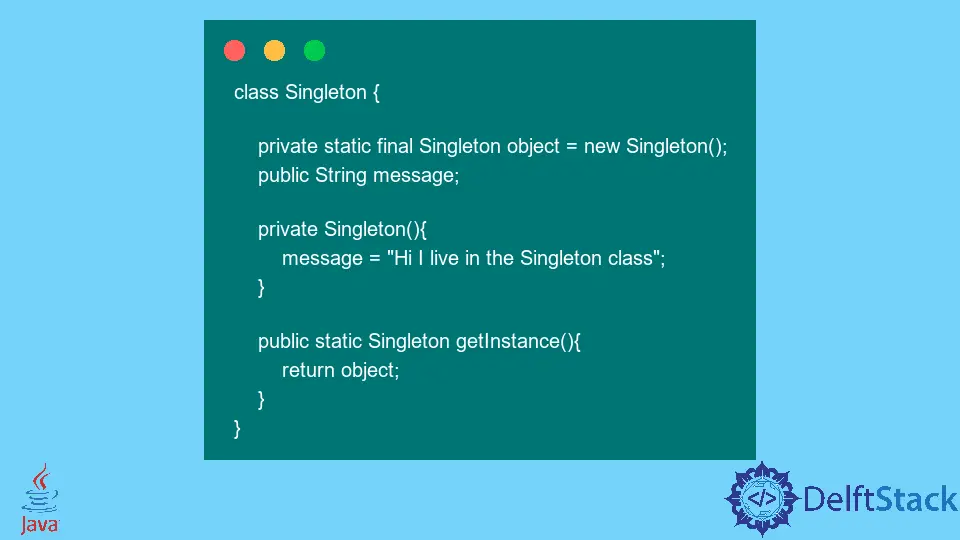
This tutorial will discuss the singleton class on a definition level and highlights its importance. We’ll describe the various design patterns to write Java singleton class and explains them with the help of code examples.
Singleton Class in Java
A Java singleton class lets us create only one instance of the class that serves globally to all classes via this single instance (also called an object of the class) at a time. The singleton classes are different from normal classes used for specific purposes.
It includes avoiding a class’s unnecessary instantiation and ensuring that a single object exists at a time. It saves memory and speeds up the work.
See the following code where we have the ABC
and XYZ
classes. The class ABC
has a method named method1
, which prints the string "Hi"
.
We create an instance of class ABC
in XYZ
class to call method1
that resides in class ABC
, and we create another instance named ABC
and call method1
again to print "Hi"
under XYZ
class. Now, we have two instances (objects) named object1
and object2
that hold different references but call the same function of the same class.
We created two objects of class ABC
which are the unnecessary instantiation of the class. We could do the same work with a single object.
Secondly, whenever we create an object, it occupies a space in memory, which means we are wasting the memory by creating multiple objects of the same class. This is where we use the singleton class to avoid these kinds of situations.
Example Code:
public class ABC {
// constructor
public ABC() {}
// method1
public void method1() {
System.out.println("Hi");
}
}
public class XYZ {
// first instance of ABC class
ABC object1 = new ABC();
object1.method1();
// second instance of ABC class
ABC object2 = new ABC();
object2.method1();
}
The singleton classes are very useful in various applications, including logging, thread pooling, configuration settings, caching, database applications, etc.
Use Various Methods to Utilize Singleton Class in Java
There are various methods to write a singleton class in Java programming that computer programmers can select from. But all of the methods fall in any one of the following design patterns of a singleton class.
- Lazy Initialization
- Early Initialization
The following factors should be considered for whatever approach we use while writing the singleton class.
- The constructor of the class must be
private
. - The singleton class must have a
static
method to return this (singleton) class instance.
Java Singleton Class Using Lazy Initialization Approach
Example Code (Singleton.java
):
class Singleton {
private static Singleton object = null;
public String message;
private Singleton() {
message = "Hi I live in the Singleton class";
}
public static Singleton getInstance() {
if (object == null)
object = new Singleton();
return object;
}
}
Example Code (Test.java
):
public class Test {
public static void main(String args[]) {
Singleton obj1 = Singleton.getInstance();
Singleton obj2 = Singleton.getInstance();
Singleton obj3 = Singleton.getInstance();
System.out.println("Hashcode of obj1 is " + obj1.hashCode());
System.out.println("Hashcode of obj2 is " + obj2.hashCode());
System.out.println("Hashcode of obj3 is " + obj3.hashCode());
if (obj1 == obj2 && obj2 == obj3) {
System.out.println("Three instances reference to the same memory location");
} else {
System.out.println("All are not referencing to the same memory location");
}
}
}
Output:
Hashcode of obj1 is 1490180672
Hashcode of obj2 is 1490180672
Hashcode of obj3 is 1490180672
Three instances reference to the same memory location
In this method, the class object is only created when it is needed. Using this method, we can avoid the unnecessary instantiation of the Singleton.java
class.
In the Singleton.java
class, we create a private static
object (instance) of the class but don’t initialize it. Then, we write a private
constructor that ensures no other class can call its default constructor (public
) to create an object.
Finally, we write a static
method that checks the instance of the Singleton.java
class, and it only creates the instance of this class if it is null
. In the Test.java
class, we can confirm by creating different objects to ensure that only one instance is created and called every time.
Java Singleton Class Using Early Initialization Approach
Example Code (Singleton.java
):
class Singleton {
private static final Singleton object = new Singleton();
public String message;
private Singleton() {
message = "Hi I live in the Singleton class";
}
public static Singleton getInstance() {
return object;
}
}
Example Code (Test.java
):
public class Test {
public static void main(String args[]) {
Singleton obj1 = Singleton.getInstance();
Singleton obj2 = Singleton.getInstance();
Singleton obj3 = Singleton.getInstance();
System.out.println("Hashcode of obj1 is " + obj1.hashCode());
System.out.println("Hashcode of obj2 is " + obj2.hashCode());
System.out.println("Hashcode of obj3 is " + obj3.hashCode());
if (obj1 == obj2 && obj2 == obj3) {
System.out.println("Three instances reference to the same memory location");
} else {
System.out.println("All are not referencing to the same memory location");
}
}
}
Output:
Hashcode of obj1 is 1490180672
Hashcode of obj2 is 1490180672
Hashcode of obj3 is 1490180672
Three instances reference to the same memory location
In the Singleton.java
class, we create a private static final
instance of the class and initialize it. Then, we write a private
constructor and a static method to return the instance of this class.
Remember, the Signleton.java
class instance would be created at load time. We can also make the instance of the class as public static final
rather than private static final
to get direct access out of the class.
Further, we have the same Test.java
class that prints and makes sure that only one instance is being called every time.