How to Fix Java.IO.Ioexception
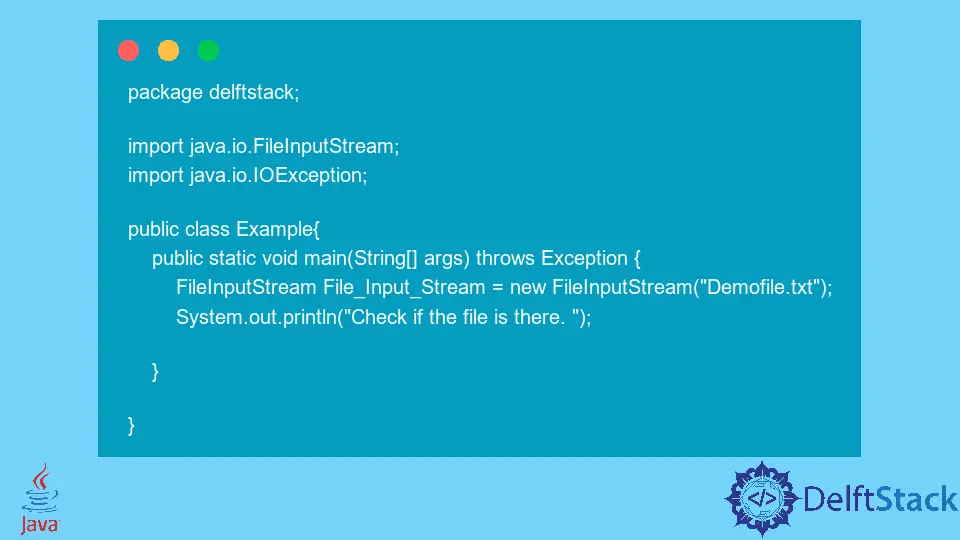
This tutorial demonstrates the java.io.ioexception
in Java.
the java.io.IOException
in Java
The IOexception
is the most common exception in Java. The most common reasons for this exception are:
- Reading a file, but that file doesn’t exist.
- Reading a file but don’t have permission.
- Writing a file, but the disc space is not available.
- When reading a stream, but that stream is closed by some other process.
- When reading a network file, but that network is disconnected.
The Java.IO.IOexception
provides exceptions for system input and output data streams and serialization.
There are also many other reasons for the IO exceptions in Java. The table below shows the types or subclasses of exceptions in Java:
Interface | Description |
---|---|
InvalidClassException |
This occurs when serialization runtime detects an invalid class. |
InvalidObjectException |
This indicates that the serialization objects failed the validation. |
NotActiveException |
This indicates that serialization or deserialization is not active. |
NotSerializableException |
This indicates that an instance is required to have a serializable interface. |
ObjectStreamException |
This indicates a superclass to all object stream classes. |
CharConversionException |
Used for character conversion exceptions. |
EOFException |
This indicates that while input, the end of the file of the stream has reached. |
FileNotFoundException |
This indicates that the file you are trying to access doesn’t exist. |
InterruptedIOException |
This indicates that the input-output operation has been interrupted. |
OptionalDataException |
This indicates the failure of an object read because of unread primitive data or the end of the data belonging to the stream’s serializable object. |
StreamCorruptedException |
This indicates that the control information read from the object stream violates the internal consistency check. |
SyncFailedException |
This indicates that a sync operation has failed. |
UnsupportedEncodingException |
This indicates that the given character encoding is not supported. |
UTFDataFormatException |
This indicates that malformed UTF-8 data has been read in the input stream, or this data is read by any class which is implementing the data input interface. |
WriteAbortedException |
This indicates that an ObjectStreamException was thrown during the write operation. |
The above exceptions are the types of subclasses of the main IO exception. Let’s try an example that throws the IOException
in Java:
package delftstack;
import java.io.FileInputStream;
import java.io.IOException;
public class Example {
public static void main(String[] args) throws Exception {
FileInputStream File_Input_Stream = new FileInputStream("Demofile.txt");
System.out.println("Check if the file is there. ");
}
}
The code above will throw the IO FileNotFoundException because the file we are accessing doesn’t exist. See output:
Exception in thread "main" java.io.FileNotFoundException: Demofile.txt (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:216)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:111)
at delftstack.Example.main(Example.java:8)
To handle these exceptions, we use try-catch
blocks to stop JVM from crashing the code. See the solution:
package delftstack;
import java.io.FileInputStream;
import java.io.IOException;
public class Example {
public static void main(String[] args) throws Exception {
try {
FileInputStream File_Input_Stream = new FileInputStream("Demofile.txt");
System.out.println("Check if the file is there. ");
} catch (IOException e) {
// e.printStackTrace(); or
System.out.println(e.getMessage());
}
}
}
Now the code above will print the same error information but without crashing the code. See output:
Demofile.txt (The system cannot find the file specified)
Let’s try another example that throws the End of File exception (EOFException
) in Java:
package delftstack;
import java.io.DataInputStream;
import java.io.EOFException;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class Example {
public void DemoCheck() {
File file = new File("delftstack.txt");
DataInputStream Data_Input_Stream = null;
try {
Data_Input_Stream = new DataInputStream(new FileInputStream(file));
while (true) {
Data_Input_Stream.readInt();
}
} catch (EOFException E_O_F_Exception) {
E_O_F_Exception.printStackTrace();
} catch (IOException I_O_Exception) {
I_O_Exception.printStackTrace();
} finally {
try {
if (Data_Input_Stream != null) {
Data_Input_Stream.close();
}
} catch (IOException I_O_Exception) {
I_O_Exception.printStackTrace();
}
}
}
public static void main(String[] args) {
Example DemoExample = new Example();
DemoExample.DemoCheck();
}
}
The code throws EOFException
because it’s trying to read a file, and the end of the file has arrived. See output:
java.io.EOFException
at java.base/java.io.DataInputStream.readInt(DataInputStream.java:398)
at delftstack.Example.DemoCheck(Example.java:16)
at delftstack.Example.main(Example.java:39)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack