Interface Default Method in Java
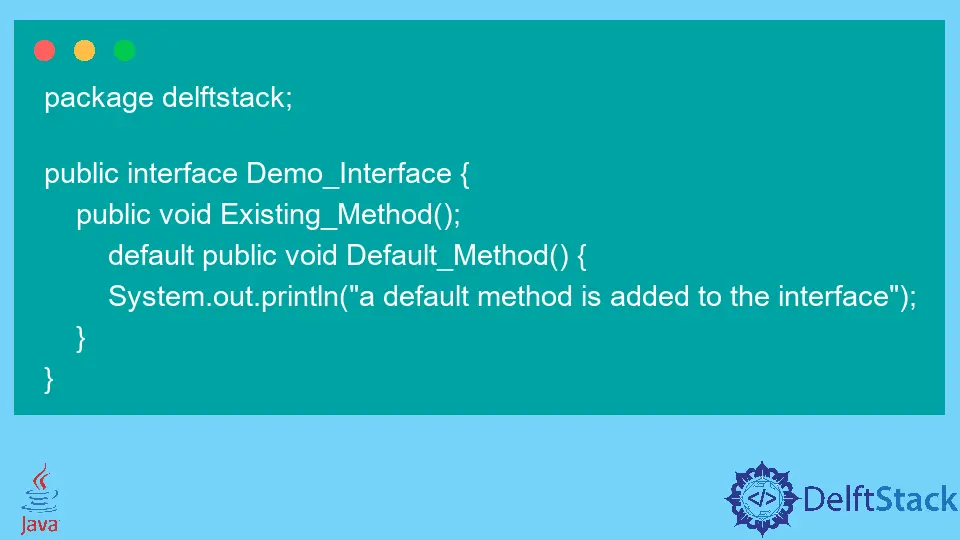
This tutorial demonstrates how to use the default
method in the interface in Java.
Interface Default Method in Java
The default
methods were introduced in Java 8 before the interfaces only had abstract methods. The default
or defender methods allow the developer to add new methods to the interface without breaking the implementation.
The default
method provides the flexibility to allow the interface to define the implementation. This implementation will be considered the default in this situation where a class has failed to provide that method’s implementation.
Let’s try to create an interface with a default
method:
package delftstack;
public interface Demo_Interface {
public void Existing_Method();
default public void Default_Method() {
System.out.println("a default method is added to the interface");
}
}
The code above adds a default
method to the interface. Let’s try another example that shows how the default
methods work:
package delftstack;
interface Demo_Interface {
// The abstract method
public void cube(int x);
// The default method
default void show() {
System.out.println("Default Method is Executed");
}
}
public class Example implements Demo_Interface {
// The implementation of an abstract method
public void cube(int x) {
System.out.println(x * x * x);
}
public static void main(String args[]) {
Example Demo = new Example();
Demo.cube(6);
// execute default method.
Demo.show();
}
}
The code above creates an abstract method to calculate the cube of an integer and a default
method to show the output. See the output:
216
Default Method is Executed
Default Methods vs. Abstract Classes
The default methods in interfaces and the abstract classes both behave in the same way, but they have a few differences:
- The abstract class can define constructors, while the
default
method can be implemented to invoke other interfaces. - The abstract class is more structured, and states can be associated with them. At the same time,
default
methods do not reference a particular implementation’s state.
Default Methods vs. Regular Methods
The default method is different from the regular method. The main differences are:
- The regular methods in classes can modify the method arguments and fields of the class. In contrast, the
default
methods can only access their own arguments because the interface doesn’t have any state. - The regular methods need to break the implementation to add new functionality to the class, while the
default
methods can add new functionality to the interface without breaking the implementation. - We can re-declare the
default
methods as abstract methods, forcing the sub-class to override them.
Default Methods and Multiple Inheritance
As we know, multiple inheritances are only possible through interfaces in Java. Now, the Java class can have multiple interfaces, and each interface can define a default
method with the same method signature, creating a conflict between the inherited methods.
See example:
package delftstack;
interface Interface_One {
default void defaultMethod() {
System.out.println("Interface One default method");
}
}
interface Interface_Two {
default void defaultMethod() {
System.out.println("Interface Two default method");
}
}
public class Example implements Interface_One, Interface_Two {}
The code above will have a compilation error. See the output:
java: class Example inherits unrelated defaults for defaultMethod() from types Interface_One and Interface_Two
There is a simple solution to this error; we need to provide a default
method implementation in the class Example
. See example:
package delftstack;
interface Interface_One {
default void defaultMethod() {
System.out.println("Interface One default method");
}
}
interface Interface_Two {
default void defaultMethod() {
System.out.println("Interface Two default method");
}
}
public class Example implements Interface_One, Interface_Two {
public void defaultMethod() {}
}
This will solve the problem. Furthermore, if you want to invoke the default
method from the super interface in the class, you can use this:
package delftstack;
interface Interface_One {
default void defaultMethod() {
System.out.println("Interface One default method");
}
}
interface Interface_Two {
default void defaultMethod() {
System.out.println("Interface Two default method");
}
}
public class Example implements Interface_One, Interface_Two {
public void defaultMethod() {
// other code
Interface_One.super.defaultMethod();
}
}
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook