Static Interface in Java
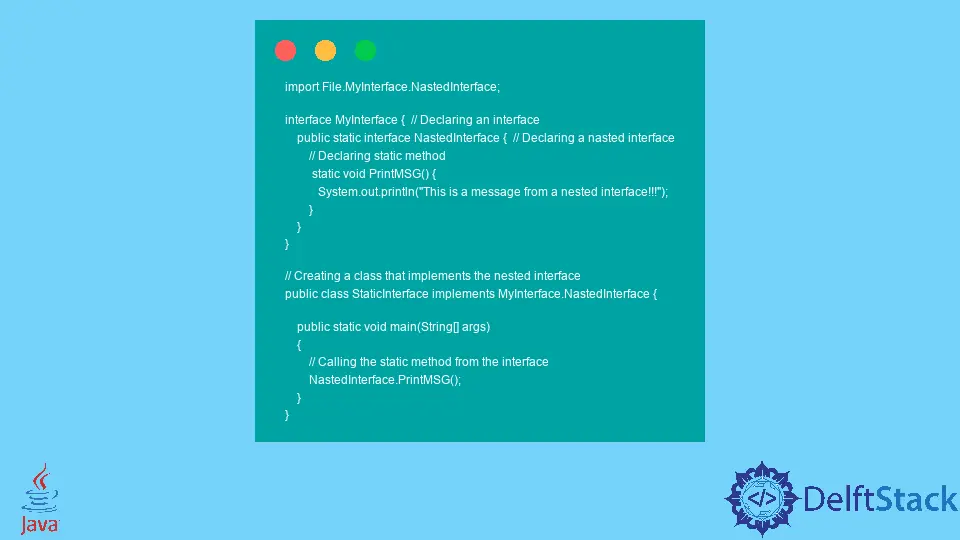
The interface
is the most commonly used element for object-oriented programming languages like Java, and the keyword static
is used to make the element fixed.
This article will show how we can declare a static interface. Also, we will discuss the topic by using necessary examples and explanations to make the topic easier.
Note that you can declare an interface
to static when the interface
is nested or a child of another interface.
Use static
in Nested Interface in Java
In our below example, we demonstrate the use of static
in the nested interface
. But it’s optional to use the keyword static
when declaring a nested interface
as it will show the same result when the keyword static
is not used.
Now the code for our example will be like the below.
import File.MyInterface.NastedInterface;
interface MyInterface { // Declaring an interface
public static interface NastedInterface { // Declaring a nasted interface
// Declaring static method
static void PrintMSG() {
System.out.println("This is a message from a nested interface!!!");
}
}
}
// Creating a class that implements the nested interface
public class StaticInterface implements MyInterface.NastedInterface {
public static void main(String[] args) {
// Calling the static method from the interface
NastedInterface.PrintMSG();
}
}
The purpose of each line is left as a comment. Please note that the keyword static
for the nested interface
is optional.
After executing the example above, you will get the below output in your console.
This is a message from a nested interface!!!
Use a static
Method Inside the interface
in Java
You also can create a static interface by declaring each of the elements static
inside the interface. When you declare a method to be static, you need to define the method inside the interface
.
Let’s go through our below example.
// Declaring an interface
interface MyInterface {
// A static method
static void PrintMSG() {
System.out.println("This is a message from the interface!!!"); // Method is defined
}
// An abstract method
void OverrideMethod(String str);
}
// Implementation Class
public class StaticInterface implements MyInterface {
public static void main(String[] args) {
StaticInterface DemoInterface = new StaticInterface(); // Creating an interface object
// An static method from the interface is called
MyInterface.PrintMSG();
// An abstract method from interface is called
DemoInterface.OverrideMethod("This is a message from class!!!");
}
// Overriding the abstract method
@Override
public void OverrideMethod(String str) {
System.out.println(str); // Defining the abstract method
}
}
In the example above, we illustrated the use of static
inside the interface
.
The purpose of each line is left as a comment. We declared two methods in the interface
in the example above, on a static method called PrintMSG
and an abstract method called OverrideMethod
.
You can notice that the method PrintMSG
is defined in the interface as it’s a static method inside the interface.
After executing the example above, you will get the below output in your console.
This is a message from the interface!!!
This is a message from class!!!
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn