Extends Comparable in Java
- Understanding the Comparable Interface
- Extending Comparable in Java
- Customizing the Comparison Logic
- Conclusion
- FAQ
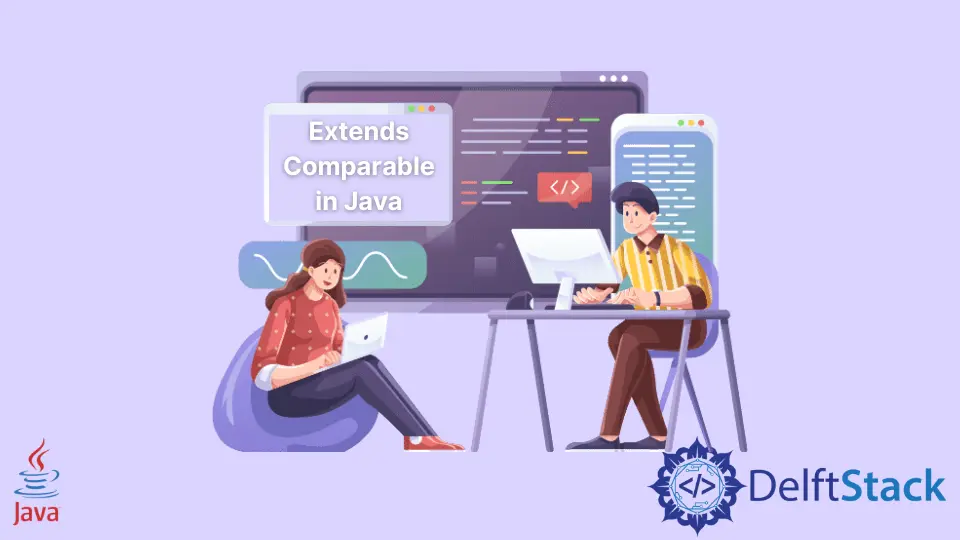
In the world of Java programming, the Comparable
interface plays a vital role in defining a natural order for objects. By implementing the Comparable
interface, you can customize how instances of your classes are compared to one another.
This tutorial delves into the intricacies of extending Comparable
in Java, providing you with the knowledge to create your own classes that can be sorted and compared effectively. Whether you are a beginner looking to understand the concept or an experienced developer seeking to refine your skills, this guide offers practical insights and code examples to help you master the art of comparison in Java.
Understanding the Comparable Interface
The Comparable
interface is part of the Java Collections Framework and is essential for sorting and comparing objects. When a class implements Comparable
, it must override the compareTo
method, which defines the natural ordering of its instances. This method returns an integer value: a negative number if the current object is less than the compared object, zero if they are equal, and a positive number if it is greater.
Key Points:
- The
Comparable
interface is generic, allowing you to specify the type of objects it compares. - It is crucial for sorting collections, such as lists and arrays, using methods like
Collections.sort()
.
Extending Comparable in Java
To extend Comparable
in Java, you will create a class that implements the interface and overrides the compareTo
method. Here’s how you can do it:
Example 1: Extending Comparable for a Custom Class
class Person implements Comparable<Person> {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public int compareTo(Person other) {
return this.age - other.age;
}
}
In this example, the Person
class implements Comparable<Person>
, allowing it to compare Person
objects based on their age. The compareTo
method subtracts the age of the current object from the age of the other object. If the result is negative, the current object is younger; if positive, it is older; and if zero, they are of the same age.
Creating Instances and Sorting
To see the comparison in action, we can create instances of the Person
class and sort them using a list.
import java.util.ArrayList;
import java.util.Collections;
public class Main {
public static void main(String[] args) {
ArrayList<Person> people = new ArrayList<>();
people.add(new Person("Alice", 30));
people.add(new Person("Bob", 25));
people.add(new Person("Charlie", 35));
Collections.sort(people);
for (Person person : people) {
System.out.println(person.name + " - " + person.age);
}
}
}
When you run this code, it creates a list of Person
objects, sorts them by age, and prints the sorted list.
Output:
Bob - 25
Alice - 30
Charlie - 35
The output demonstrates that the Person
objects are now sorted in ascending order based on their ages. The Collections.sort()
method utilizes the compareTo
implementation to arrange the objects correctly.
Customizing the Comparison Logic
You may want to extend the Comparable
interface further by customizing the comparison logic. For instance, you can compare by name instead of age or even combine multiple fields for comparison.
Example 2: Multi-field Comparison
class Employee implements Comparable<Employee> {
String name;
int age;
Employee(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public int compareTo(Employee other) {
int nameComparison = this.name.compareTo(other.name);
if (nameComparison != 0) {
return nameComparison;
}
return this.age - other.age;
}
}
In this example, the Employee
class compares employees first by their names and then by age if the names are the same. The compareTo
method first compares the names using the String
comparison method. If the names are equal, it falls back to comparing ages.
Sorting Employees
Now, let’s create instances of Employee
and sort them.
import java.util.ArrayList;
import java.util.Collections;
public class Main {
public static void main(String[] args) {
ArrayList<Employee> employees = new ArrayList<>();
employees.add(new Employee("Alice", 30));
employees.add(new Employee("Bob", 25));
employees.add(new Employee("Alice", 35));
Collections.sort(employees);
for (Employee employee : employees) {
System.out.println(employee.name + " - " + employee.age);
}
}
}
When executed, the program sorts the employees first by name and then by age.
Output:
Alice - 30
Alice - 35
Bob - 25
This output shows that the employees are sorted alphabetically by name, and if two names are the same, they are then ordered by age.
Conclusion
Extending Comparable
in Java allows you to define custom sorting logic for your objects, making it easier to manage collections of data. By implementing the compareTo
method, you can specify how instances of your classes should be compared, whether by a single field or multiple attributes. This powerful feature enhances the flexibility of your Java applications and streamlines data handling. With the examples provided, you can now confidently implement the Comparable
interface in your own projects.
FAQ
- What is the purpose of the Comparable interface in Java?
The Comparable interface allows objects of a class to be compared with each other, enabling sorting and ordering based on a natural order defined in thecompareTo
method.
-
How do you implement the compareTo method?
You override thecompareTo
method in your class to define how instances should be compared, typically returning a negative number, zero, or a positive number based on the comparison criteria. -
Can you compare objects based on multiple fields?
Yes, you can customize thecompareTo
method to compare objects based on multiple fields, allowing for more complex sorting logic. -
What happens if a class does not implement Comparable?
If a class does not implement Comparable, you cannot use sorting methods like Collections.sort() on instances of that class. -
Is it necessary to implement the Comparable interface for every class?
No, it is not necessary. You only implement Comparable for classes where you want to define a natural ordering for its instances.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn