How to Implement Multiple Interfaces in Java
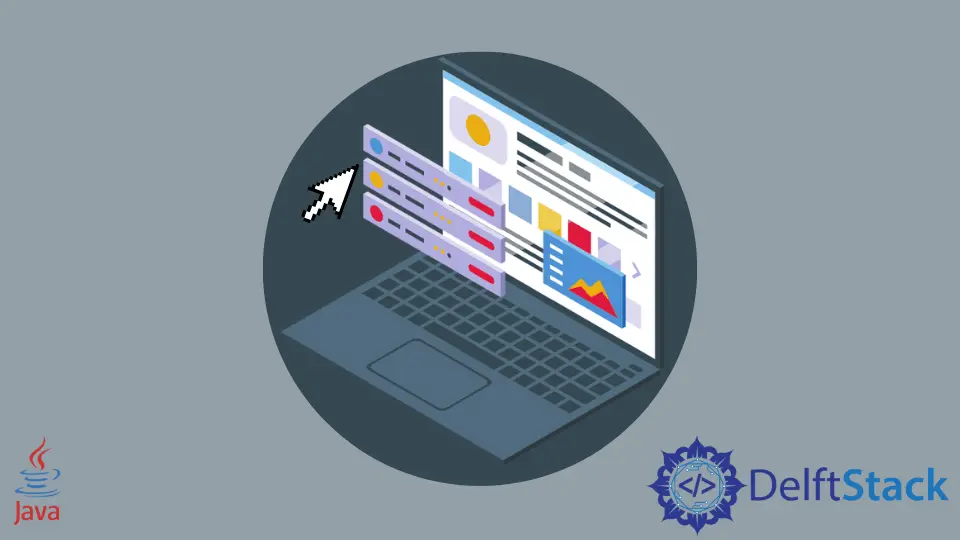
This tutorial introduces how a class can implement multiple interfaces in Java and also lists some example codes to understand the topic.
In Java, an interface is similar to a class except that it can have only abstract methods. An interface is known as a blueprint for a class, and the class that implements an interface must provide an implementation for all the abstract methods or declare the abstract itself.
In Java, the class can extend only a single class but can implement multiple interfaces. So, if somebody asks you, can a class implement multiple interfaces? Then, say YES.
Let’s start with some code examples to understand the concept. This is a general structure of multiple interface implementation.
class A implements B, C, D
....Z
Existing classes in Java collections that implement multiple interfaces are:
Apart from developer code, if we notice JDK source, we will find that Java used multiple interface implementations such as ArrayList, HashMap class, etc.
ArrayList<E>
Implements Multiple Interfaces
public class ArrayList<E>
extends AbstractList<E> implements List<E>, RandomAccess, Cloneable, Serializable
// Implements multiple interfaces
HashMap<K,V>
Implements Multiple Interfaces
public class HashMap<K, V> extends AbstractMap<K, V> implements Map<K, V>, Cloneable, Serializable
// Implements multiple interfaces
Implements Multiple Interface in Java
Java allows a class to implement multiple interfaces. So, we can implement as much as we want. In this example, we created 3 interfaces and then implemented them by using a class.
While working with the interface, make sure the class implements all its abstract methods. See the example below where we implemented all the methods of all 3 interfaces.
package javaexample;
interface A {
void showA();
}
interface B {
void showB();
}
interface C {
void showC();
}
public class SimpleTesting implements A, B, C {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting();
st.showA();
st.showB();
st.showC();
}
@Override
public void showA() {
System.out.println("Interface A");
}
@Override
public void showB() {
System.out.println("Interface B");
}
@Override
public void showC() {
System.out.println("Interface C");
}
}
Output:
Interface A
Interface B
Interface C
Interface Extends Multiple Interface in Java
An interface can also implement (extend) multiple interfaces. Java allows to interface like class and can implement multiple interfaces.
In the case of interface, we should use the externds
keyword in place of implements
to implement interfaces. See the example below.
package javaexample;
interface A {
void showA();
}
interface B {
void showB();
}
interface C {
void showC();
}
interface D extends A, B, C {
void showD();
}
public class SimpleTesting implements D {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting();
st.showA();
st.showB();
st.showC();
st.showD();
}
@Override
public void showA() {
System.out.println("Interface A");
}
@Override
public void showB() {
System.out.println("Interface B");
}
@Override
public void showC() {
System.out.println("Interface C");
}
@Override
public void showD() {
System.out.println("Interface D");
}
}
Output:
Interface A
Interface B
Interface C
Interface D
It is important that if you implement an interface in a class, then you must provide implements of abstract methods else java compiler will raise an error. See the example below.
package javaexample;
interface A {
void showA();
}
interface B {
void showB();
}
public class SimpleTesting implements A, B {
public static void main(String[] args) {
SimpleTesting st = new SimpleTesting();
st.showA();
st.showB();
}
@Override
public void showA() {
System.out.println("Interface A");
}
}
Output:
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
The type SimpleTesting must implement the inherited abstract method B.showB()