Java의 인터페이스 기본 메소드
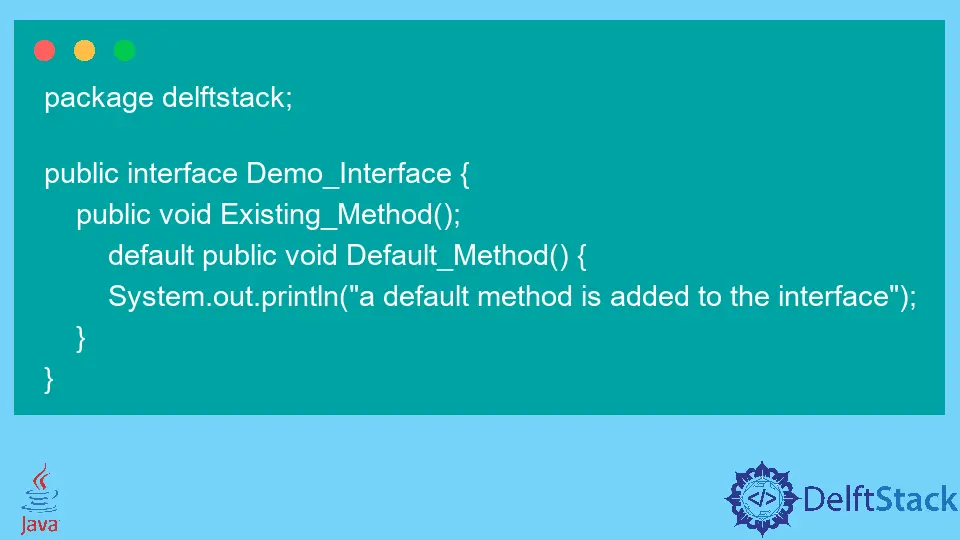
이 자습서는 Java의 인터페이스에서 default
메서드를 사용하는 방법을 보여줍니다.
Java의 인터페이스 기본 메소드
default
메서드는 인터페이스에 추상 메서드만 있기 전에 Java 8에서 도입되었습니다. 기본
또는 방어자 방법을 사용하면 개발자가 구현을 중단하지 않고 인터페이스에 새 방법을 추가할 수 있습니다.
default
메소드는 인터페이스가 구현을 정의할 수 있도록 유연성을 제공합니다. 이 구현은 클래스가 해당 메서드의 구현을 제공하지 못한 상황에서 기본값으로 간주됩니다.
기본
메서드를 사용하여 인터페이스를 생성해 보겠습니다.
package delftstack;
public interface Demo_Interface {
public void Existing_Method();
default public void Default_Method() {
System.out.println("a default method is added to the interface");
}
}
위의 코드는 인터페이스에 default
메서드를 추가합니다. 기본
방법이 작동하는 방식을 보여주는 또 다른 예를 살펴보겠습니다.
package delftstack;
interface Demo_Interface {
// The abstract method
public void cube(int x);
// The default method
default void show() {
System.out.println("Default Method is Executed");
}
}
public class Example implements Demo_Interface {
// The implementation of an abstract method
public void cube(int x) {
System.out.println(x * x * x);
}
public static void main(String args[]) {
Example Demo = new Example();
Demo.cube(6);
// execute default method.
Demo.show();
}
}
위의 코드는 정수의 세제곱을 계산하는 추상 메서드와 출력을 표시하는 기본
메서드를 만듭니다. 출력을 참조하십시오.
216
Default Method is Executed
기본 메서드와 추상 클래스 비교
인터페이스와 추상 클래스의 기본 메서드는 모두 동일한 방식으로 작동하지만 몇 가지 차이점이 있습니다.
- 추상 클래스는 생성자를 정의할 수 있는 반면
default
메서드는 다른 인터페이스를 호출하도록 구현될 수 있습니다. - 추상 클래스는 더 구조화되어 있으며 상태를 연관시킬 수 있습니다. 동시에
기본
메서드는 특정 구현 상태를 참조하지 않습니다.
기본 방법 대 일반 방법
기본 방법은 일반 방법과 다릅니다. 주요 차이점은 다음과 같습니다.
- 클래스의 일반 메서드는 클래스의 메서드 인수 및 필드를 수정할 수 있습니다. 반대로
기본
메서드는 인터페이스에 상태가 없기 때문에 자신의 인수에만 액세스할 수 있습니다. - 일반 메서드는 클래스에 새 기능을 추가하기 위해 구현을 중단해야 하는 반면
기본
메서드는 구현을 중단하지 않고 인터페이스에 새 기능을 추가할 수 있습니다. 기본
메서드를 추상 메서드로 다시 선언하여 하위 클래스가 강제로 재정의하도록 할 수 있습니다.
기본 메서드 및 다중 상속
아시다시피 다중 상속은 Java의 인터페이스를 통해서만 가능합니다. 이제 Java 클래스는 여러 인터페이스를 가질 수 있으며 각 인터페이스는 동일한 메서드 서명을 사용하여 기본
메서드를 정의하여 상속된 메서드 간에 충돌을 일으킬 수 있습니다.
예를 참조하십시오:
package delftstack;
interface Interface_One {
default void defaultMethod() {
System.out.println("Interface One default method");
}
}
interface Interface_Two {
default void defaultMethod() {
System.out.println("Interface Two default method");
}
}
public class Example implements Interface_One, Interface_Two {}
위의 코드에는 컴파일 오류가 있습니다. 출력을 참조하십시오.
java: class Example inherits unrelated defaults for defaultMethod() from types Interface_One and Interface_Two
이 오류에 대한 간단한 해결책이 있습니다. Example
클래스에서 default
메서드 구현을 제공해야 합니다. 예를 참조하십시오:
package delftstack;
interface Interface_One {
default void defaultMethod() {
System.out.println("Interface One default method");
}
}
interface Interface_Two {
default void defaultMethod() {
System.out.println("Interface Two default method");
}
}
public class Example implements Interface_One, Interface_Two {
public void defaultMethod() {}
}
이것은 문제를 해결할 것입니다. 또한 클래스의 수퍼 인터페이스에서 default
메서드를 호출하려는 경우 다음을 사용할 수 있습니다.
package delftstack;
interface Interface_One {
default void defaultMethod() {
System.out.println("Interface One default method");
}
}
interface Interface_Two {
default void defaultMethod() {
System.out.println("Interface Two default method");
}
}
public class Example implements Interface_One, Interface_Two {
public void defaultMethod() {
// other code
Interface_One.super.defaultMethod();
}
}
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook