Java의 BiFunction 인터페이스
-
Java의 BiFunction
apply()
메소드 예제 -
Java의 BiFunction
andThen()
메서드 -
BiFunction 인터페이스에서
NullPointerException
방지 - Java에서 HashMap을 사용하는 BiFunction
- BiFunction 및 메서드 참조
- 결론
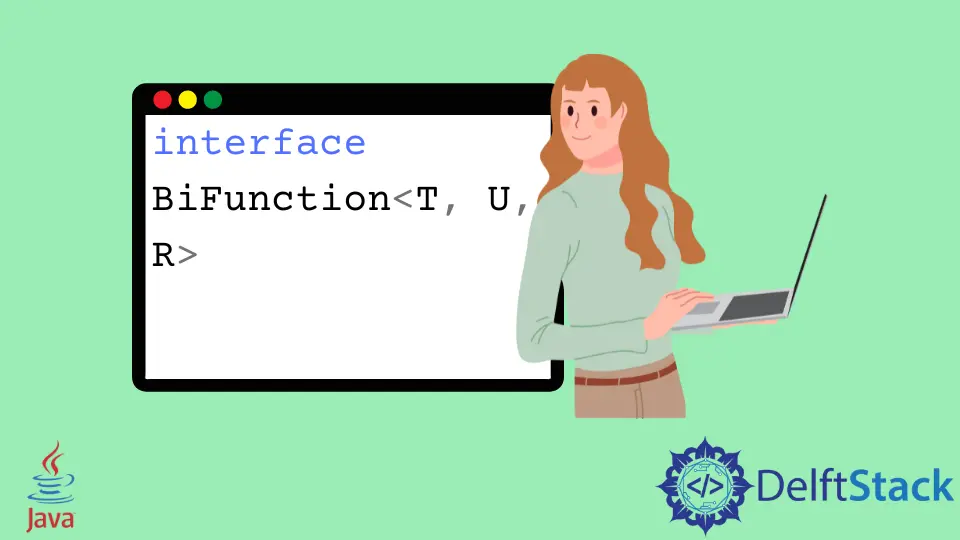
이 자습서에서는 Java의 BiFunction 인터페이스에 대해 설명합니다.
BiFunction 인터페이스는 Java 8에 도입된 내장 기능 인터페이스이며 java.util.function
패키지에 있습니다. 두 개의 제네릭(인수 유형 하나와 반환 유형 하나)을 사용하는 Function 인터페이스와 달리 BiFunction은 두 개의 인수를 사용하여 결과를 생성합니다.
두 개의 인수를 사용하고 결과를 BiFunction 유형의 개체에 반환하는 람다 식 또는 메서드 참조를 할당할 수 있습니다.
BiFunction은 T
, U
, R
의 세 가지 제네릭을 사용합니다. T
및 U
는 각각 첫 번째 및 두 번째 인수의 유형입니다. R
은 함수의 결과 유형입니다.
interface BiFunction<T, U, R>
BiFunction 인터페이스에는 두 가지 방법이 있습니다.
apply()
: 인수에 대해 정의된 연산을 수행하고 결과를 반환합니다. 이 메서드의 구문은 다음과 같습니다.
R apply(T t, U u) // R is the return type. T and U are the types of the two arguments
andThen()
: 구성된 함수의 결과를 반환합니다. 간단히 말해서, 먼저 두 인수에 대해 BiFunction을 실행하고 결과를 생성합니다. 다음으로 결과를 함수에 전달합니다. 이 메서드의 구문은 다음과 같습니다.
default<V> BiFunction<T, U, V> andThen(Function<? super R, ? extends V> after)
Java의 BiFunction apply()
메소드 예제
Integer 및 Double 형식 인수를 사용하고 String을 반환하는 간단한 BiFunction을 만들어 보겠습니다. 인수를 전달하고 결과를 얻기 위해 apply()
메소드를 사용했습니다.
아래 예를 참조하십시오.
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
// BiFunction with arguments of type Integer and Double
// and return type of String
BiFunction<Integer, Double, String> biFunction = (a, b) -> {
double result = a * b;
String s = "The result from the BiFunction is: " + result;
return s;
};
String output = biFunction.apply(10, 15.5);
System.out.print(output);
}
}
출력:
The result from the BiFunction is: 155.0
Java의 BiFunction andThen()
메서드
위에서 정의한 BiFunction의 결과를 Function에 전달해보자. 우리는 andThen()
메소드를 사용할 것입니다. 이 메서드는 먼저 BiFunction의 결과를 평가한 다음 그 결과를 after
함수에 전달합니다.
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
// BiFunction with arguments of type Integer and Double
// and return type of String
BiFunction<Integer, Double, String> biFunction = (a, b) -> {
double result = a * b;
String s = "The result from the BiFunction is: " + result;
return s;
};
// Function with argument of type String
// and return type of Integer
Function<String, Integer> after = s -> {
Integer length = s.length();
return length;
};
int output = biFunction.andThen(after).apply(
10, 15.5); // first evaluates the BiFunction and then the Function
System.out.print(output);
}
}
출력:
40
BiFunction 인터페이스에서 NullPointerException
방지
andThen()
메서드에 대한 null 참조를 전달하면 코드는 NullPointerException
을 반환합니다. 아래 예를 참조하십시오. 우리는 그러한 실수를 피하고 항상 try...catch
블록에 코드를 넣어야 합니다.
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
BiFunction<Integer, Double, String> biFunction = (a, b) -> {
double result = a * b;
String s = "The result from the BiFunction is: " + result;
return s;
};
Function<String, Integer> after = null;
int output = biFunction.andThen(after).apply(10, 15.5);
System.out.print(output);
}
}
출력:
Exception in thread "main" java.lang.NullPointerException
at java.base/java.util.Objects.requireNonNull(Objects.java:222)
at java.base/java.util.function.BiFunction.andThen(BiFunction.java:69)
at SimpleTesting.main(SimpleTesting.java:15)
andThen()
메서드를 사용하여 두 개의 BiFunction을 통합할 수 없습니다.
Java에서 HashMap을 사용하는 BiFunction
BiFunctions는 compute()
, computeIfPresent()
, merge()
및 replaceAll()
과 같은 몇 가지 HashMap 메서드에서 인수로 사용됩니다.
HashMap에서 BiFunction을 사용하는 예제를 만들어 보겠습니다. 학생 명부 번호와 점수를 저장하는 HashMap을 고려하십시오. 롤 번호 101
을 제외한 모든 학생의 점수에 5
를 추가해야 합니다.
import java.util.HashMap;
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
HashMap<Integer, Double> scoreMap = new HashMap<>();
scoreMap.put(101, 95.2);
scoreMap.put(102, 86.0);
scoreMap.put(103, 91.9);
scoreMap.put(104, 72.8);
scoreMap.put(105, 89.5);
System.out.println("Intial HashMap: " + scoreMap);
// BiFunction with arguments of type Integer and Double
// and return type of Double
BiFunction<Integer, Double, Double> biFunction = (key, value) -> {
if (key == 101)
return value;
else
return value + 5;
};
scoreMap.replaceAll(biFunction);
System.out.print("HashMap After The Update: " + scoreMap);
}
}
출력:
Intial HashMap: {101=95.2, 102=86.0, 103=91.9, 104=72.8, 105=89.5}
HashMap After The Update: {101=95.2, 102=91.0, 103=96.9, 104=77.8, 105=94.5}
BiFunction 및 메서드 참조
지금까지 BiFunction 인터페이스를 람다 식에 대한 참조로 사용했습니다. BiFunction을 사용하여 메서드를 참조할 수도 있습니다. 이 메서드는 두 개의 인수만 사용하고 결과를 반환해야 합니다.
주어진 두 정수의 합이 짝수인지 여부를 확인하는 간단한 정적 메서드를 작성해 보겠습니다. 이 메서드 참조를 BiFunction에 할당할 수 있습니다. 아래 예를 참조하십시오.
import java.util.function.*;
public class SimpleTesting {
static boolean isSumEven(int a, int b) {
int sum = a + b;
if (sum % 2 == 0)
return true;
else
return false;
}
public static void main(String args[]) {
BiFunction<Integer, Integer, Boolean> biFunction = SimpleTesting::isSumEven;
System.out.println("Is sum of 10 and 21 even? " + biFunction.apply(10, 21));
System.out.print("Is sum of 5 and 7 even? " + biFunction.apply(5, 7));
}
}
출력:
Is sum of 10 and 21 even? false
Is sum of 5 and 7 even? true
결론
Java BiFunction 인터페이스는 기능적 인터페이스입니다. 람다 식 또는 메서드 참조에 대한 할당 대상으로 사용됩니다. BiFunction 인터페이스는 두 개의 인수를 전달해야 할 때 Function 인터페이스보다 선호됩니다.
BiFunction 인터페이스에는 apply()
및 andThen()
의 두 가지 메소드가 있습니다. apply()
메소드는 주어진 두 인수에 함수를 적용합니다. andThen()
메서드는 BiFunction의 결과를 Function 인터페이스에 전달하는 데 사용됩니다.