Java 中的 BiFunction 接口
-
Java 中的 BiFunction
apply()
方法示例 -
Java 中的 BiFunction
andThen()
方法 -
在 BiFunction 接口中避免
NullPointerException
- 在 Java 中使用 HashMap 的 BiFunction
- BiFunction 和方法参考
- 结论
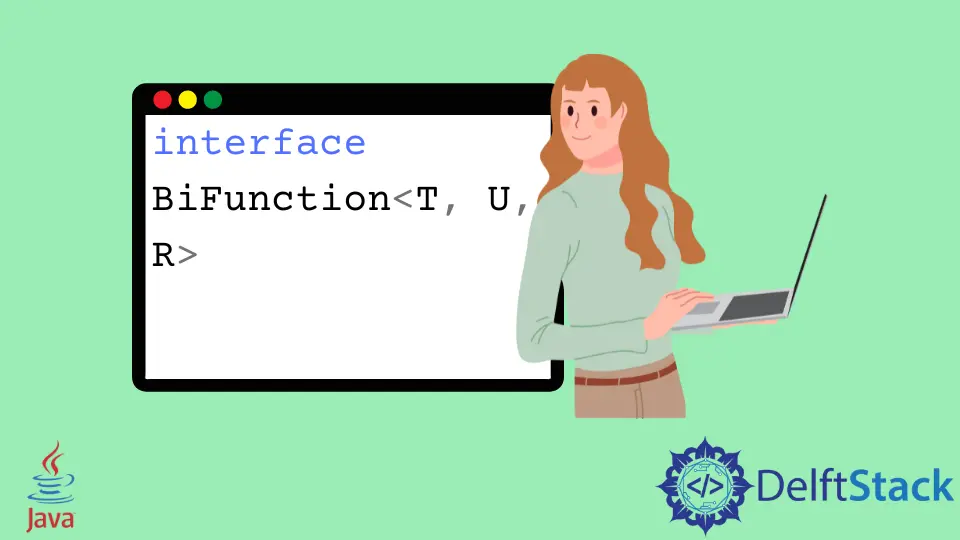
在本教程中,我们将讨论 Java 中的 BiFunction 接口。
BiFunction 接口是 Java 8 中引入的内置函数式接口,位于 java.util.function
包中。与接受两个泛型(一个参数类型和一个返回类型)的 Function 接口不同,BiFunction 接受两个参数并产生一个结果。
我们可以分配一个 lambda 表达式或一个方法引用,它接受两个参数并将结果返回给 BiFunction 类型的对象。
BiFunction 采用三个泛型 - T
、U
和 R
。T
和 U
分别是第一个和第二个参数的类型。R
是函数结果的类型。
interface BiFunction<T, U, R>
BiFunction 接口有两个方法:
apply()
:它对参数执行定义的操作并返回结果。这个方法的语法是:
R apply(T t, U u) // R is the return type. T and U are the types of the two arguments
andThen()
:它返回组合函数的结果。简单来说,它首先在两个参数上运行 BiFunction 并产生一个结果。接下来,它将结果传递给函数。这个方法的语法是:
default<V> BiFunction<T, U, V> andThen(Function<? super R, ? extends V> after)
Java 中的 BiFunction apply()
方法示例
让我们创建一个简单的 BiFunction,它接受 Integer 和 Double 类型的参数并返回一个 String。我们使用 apply()
方法来传递参数并获得结果。
请参见下面的示例。
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
// BiFunction with arguments of type Integer and Double
// and return type of String
BiFunction<Integer, Double, String> biFunction = (a, b) -> {
double result = a * b;
String s = "The result from the BiFunction is: " + result;
return s;
};
String output = biFunction.apply(10, 15.5);
System.out.print(output);
}
}
输出:
The result from the BiFunction is: 155.0
Java 中的 BiFunction andThen()
方法
让我们将上面定义的 BiFunction 的结果传递给一个函数。我们将使用 andThen()
方法。此方法首先评估 BiFunction 的结果,然后将该结果传递给 after
函数。
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
// BiFunction with arguments of type Integer and Double
// and return type of String
BiFunction<Integer, Double, String> biFunction = (a, b) -> {
double result = a * b;
String s = "The result from the BiFunction is: " + result;
return s;
};
// Function with argument of type String
// and return type of Integer
Function<String, Integer> after = s -> {
Integer length = s.length();
return length;
};
int output = biFunction.andThen(after).apply(
10, 15.5); // first evaluates the BiFunction and then the Function
System.out.print(output);
}
}
输出:
40
在 BiFunction 接口中避免 NullPointerException
如果我们将空引用传递给 andThen()
方法,代码将返回 NullPointerException
。请参见下面的示例。我们应该避免此类错误,并始终将代码放在 try...catch
块中。
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
BiFunction<Integer, Double, String> biFunction = (a, b) -> {
double result = a * b;
String s = "The result from the BiFunction is: " + result;
return s;
};
Function<String, Integer> after = null;
int output = biFunction.andThen(after).apply(10, 15.5);
System.out.print(output);
}
}
输出:
Exception in thread "main" java.lang.NullPointerException
at java.base/java.util.Objects.requireNonNull(Objects.java:222)
at java.base/java.util.function.BiFunction.andThen(BiFunction.java:69)
at SimpleTesting.main(SimpleTesting.java:15)
请注意,我们不能使用 andThen()
方法集成两个 BiFunction。
在 Java 中使用 HashMap 的 BiFunction
BiFunctions 在一些 HashMap 方法中用作参数,例如 compute()
、computeIfPresent()
、merge()
和 replaceAll()
。
让我们创建一个在 HashMap 中使用 BiFunction 的示例。考虑一个存储学生卷号及其分数的 HashMap。我们需要在每个学生的分数上加上 5
,除了卷号 101
。
import java.util.HashMap;
import java.util.function.*;
public class SimpleTesting {
public static void main(String args[]) {
HashMap<Integer, Double> scoreMap = new HashMap<>();
scoreMap.put(101, 95.2);
scoreMap.put(102, 86.0);
scoreMap.put(103, 91.9);
scoreMap.put(104, 72.8);
scoreMap.put(105, 89.5);
System.out.println("Intial HashMap: " + scoreMap);
// BiFunction with arguments of type Integer and Double
// and return type of Double
BiFunction<Integer, Double, Double> biFunction = (key, value) -> {
if (key == 101)
return value;
else
return value + 5;
};
scoreMap.replaceAll(biFunction);
System.out.print("HashMap After The Update: " + scoreMap);
}
}
输出:
Intial HashMap: {101=95.2, 102=86.0, 103=91.9, 104=72.8, 105=89.5}
HashMap After The Update: {101=95.2, 102=91.0, 103=96.9, 104=77.8, 105=94.5}
BiFunction 和方法参考
到目前为止,我们已经使用 BiFunction 接口作为 lambda 表达式的参考。也可以使用 BiFunction 引用方法。该方法必须只接受两个参数并返回一个结果。
让我们编写一个简单的静态方法来检查两个给定整数的和是否为偶数。我们可以将此方法引用分配给 BiFunction。请参见下面的示例。
import java.util.function.*;
public class SimpleTesting {
static boolean isSumEven(int a, int b) {
int sum = a + b;
if (sum % 2 == 0)
return true;
else
return false;
}
public static void main(String args[]) {
BiFunction<Integer, Integer, Boolean> biFunction = SimpleTesting::isSumEven;
System.out.println("Is sum of 10 and 21 even? " + biFunction.apply(10, 21));
System.out.print("Is sum of 5 and 7 even? " + biFunction.apply(5, 7));
}
}
输出:
Is sum of 10 and 21 even? false
Is sum of 5 and 7 even? true
结论
Java BiFunction 接口是一个函数式接口。它用作 lambda 表达式或方法引用的赋值目标。当我们需要传递两个参数时,BiFunction 接口优于 Function 接口。
BiFunction 接口包含两个方法:apply()
和 andThen()
。apply()
方法将函数应用于两个给定的参数。andThen()
方法用于将 BiFunction 的结果传递给 Function 接口。
相关文章 - Java Stream
- Java 中的 Stream 的 reduce 操作
- Java 中的流过滤器
- Java 中的 findFirst 流方法
- 在 Java 中将 Stream 元素转换为映射
- Java 中的 flatMap
- 在 Java 中把数组转换为流