How to Ignore Exception in Java
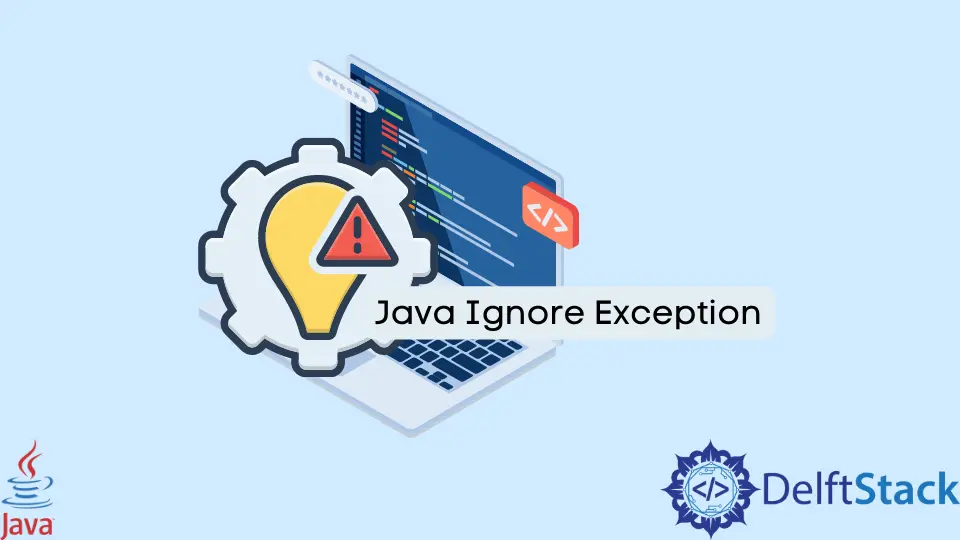
In computer programming, during software testing, we cannot cover every input; rather, we test some generic scenarios, units and conditional paths of the software. But still, there are some scenarios which you cannot prevent from occurring run-time errors and getting terminated until and unless you have used proper exception handling mechanisms.
But thanks to Java programming, which offers concepts like exception handling, you can prevent run-time errors and handle them before terminating or crashing your program.
Exception Handling in Java
Exception handling is a technique that helps the developers to handle errors before terminating your program. It is used to handle the abnormal conditions in your program that can cause errors to maintain the normal flow of the program.
But still, it requires the developer’s and tester’s skills of how professionally and efficiently they use the exception handling techniques to ignore exceptions in Java.
We can use special keywords to handle exceptions in Java: try
, catch
, finally
, throws
, and throw
.
Let’s say we have a small program that divides two numbers.
package codes;
public class Codes {
public static void main(String[] args) {
System.out.println(12 / 2);
System.out.println(12 / 1);
System.out.println(12 / 0);
System.out.println("This is the line after the exception");
}
}
Output:
6
12
Exception in thread "main" java.lang.ArithmeticException: / by zero
at codes.Codes.main(Codes.java:10)
In the above example, 12 is first divided by 2, 1, and then 3. The first two numbers, 2 and 1, have smoothly divided the number 12 without causing any error, but the last digit, 0, has caused an exception and terminated the program abnormally.
And the reason is that we cannot divide any number with 0, so the compiler has thrown a run-time error and terminated the program. As you can see, the last line of the program isn’t executed due to the abnormal termination.
Ignore Exceptions in Java
As we have seen in the above example, we got an ArithmeticException
by dividing a number by 0. But there are ways to ignore these exceptions or abnormal conditions in Java.
Let’s take the above example again and try to ignore the exception to maintain the normal flow of the program.
package codes;
public class Codes {
public static void main(String[] args) {
try {
System.out.println(12 / 2);
System.out.println(12 / 1);
System.out.println(12 / 0);
} catch (ArithmeticException e) {
System.out.println("Division by Zero() isn't a valid expression\n"
+ "The type of this exception is " + e);
}
System.out.println("This is the line after the exception");
}
}
Output:
6
12
Division by Zero() isn't a valid expression
The type of this exception is java.lang.ArithmeticException: / by zero
This is the line after the exception
In this program, we have used the try-catch
block to ignore exceptions in the Java program. Basically, in the try
block, we have the code that may cause run-time errors, and if there is any exception found in the try
block, then the catch
block is used to display any custom message to interpret the exception.
As in this case, the first two statements run smoothly, the third causes an exception handled by the try-catch
, and the last line of code is printed.
Common Examples of Java Exceptions
In Java, we have different exception types; each is responsible for scenarios or conditions. The following are some of the most common exceptions.
ClassNotFoundException
NoSuchMethodException
NullPointerException
ArrayIndexOutOfBoundsException
IllegalStateException
ArithmeticException
FileNotFoundException
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn