How to Close a Scanner in Java
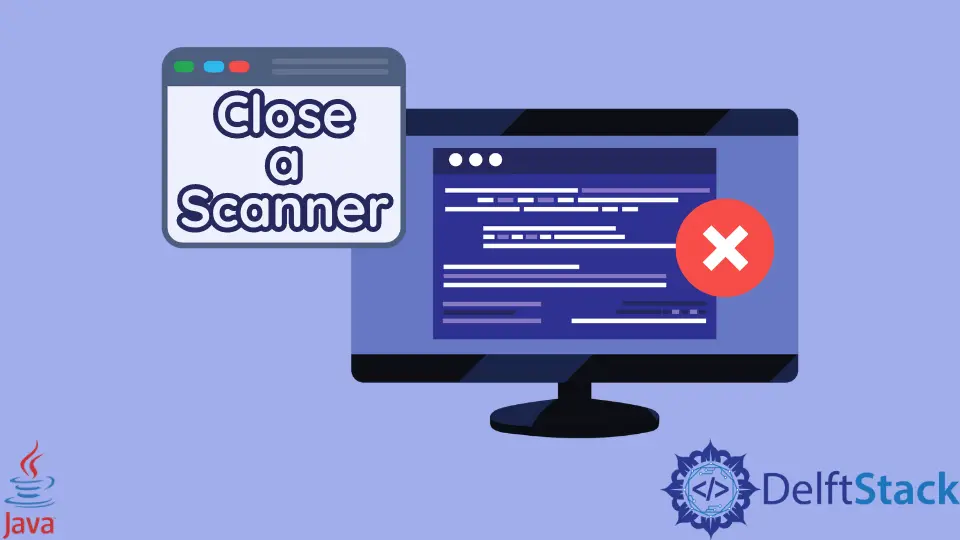
In this tutorial, we will learn how to close a scanner in Java and when we should use it.
The Scanner
class has a method close()
that is especially available to close the opened scanner. It is a good practice to close a scanner explicitly.
Below are the examples that show how and when we can use the Scanner.close()
method.
Close a Scanner
in Java Using close()
The Scanner.close()
method in Java is used to close the Scanner
object, releasing any system resources associated with it. Closing a Scanner
is essential for proper resource management, especially when the scanner is reading from external sources like files or streams.
This method should be called when the scanner is no longer needed to prevent potential resource leaks and ensure efficient memory usage. Additionally, closing the scanner prevents further operations on it and signals the end of its usage.
Case 1: Handling Standard Input From the User
In Java, when using the Scanner
class to handle standard input from the user, it’s essential to close the scanner using the close()
method to release system resources. This ensures proper cleanup and prevents resource leaks.
Always include scanner.close()
after reading user input to maintain code efficiency and avoid potential issues with unclosed resources.
Let’s delve into a complete working code example demonstrating how to use the close()
method with a Scanner
. Additionally, we’ll introduce a scenario where an IllegalStateException
may occur.
import java.util.Scanner;
public class ScannerClosingExample {
public static void main(String[] args) {
// Creating a Scanner for standard input
Scanner userInputScanner = new Scanner(System.in);
// Prompting the user for input
System.out.print("Enter Pet's Name: ");
try {
// Reading user input
String userInput = userInputScanner.nextLine();
// Printing the input
System.out.println("Pet's Name: " + userInput);
// Attempting to close the Scanner
userInputScanner.close();
// Simulating an illegal state by using the closed Scanner
userInputScanner.nextLine(); // This line will throw an IllegalStateException
} catch (IllegalStateException e) {
System.err.println("IllegalStateException: Scanner is closed.");
}
}
}
In the presented code example, the process begins with the creation of a Scanner
named userInputScanner
designed to interact with standard input, specifically associated with System.in
.
Subsequently, a user prompt is displayed, inviting input from the user. The program then employs the nextLine()
method to capture the text entered by the user.
Following this, the entered input is printed to the console, demonstrating the effective utilization of the Scanner
for handling standard input.
As a crucial aspect of resource management, the close()
method is invoked on the Scanner
to release associated resources after their intended use. To highlight the significance of this step, an intentional attempt to use nextLine()
after closing the Scanner
is made, resulting in the triggering of an IllegalStateException
.
The code includes a mechanism to catch and handle this exception, presenting an informative error message that underscores the importance of closing the scanner appropriately.
Output:
Enter Pet's Name: Pillow
Pet's Name: Pillow
IllegalStateException: Scanner is closed.
When the user runs this program, they will be prompted to enter something. After entering the text, the program will print the input. However, when it attempts to use nextLine()
on the closed Scanner
, an IllegalStateException
will be thrown. The catch block will handle this exception, indicating that the scanner is closed.
Case 2: After Reading the Contents of a File
In Java, when reading contents from a file using the Scanner
class, it’s crucial to close the scanner with the close()
method after finishing the file operations. This ensures proper resource management, releases system resources and prevents potential issues related to unclosed file connections.
Always include scanner.close()
to maintain code reliability and efficiency when handling file input.
Failing to close a Scanner
instance after reading a file could lead to resource leaks and unexpected behavior.
Here’s a simple example of using the close()
method with a Scanner
after reading contents from a file :
example.txt
:
Hello! My name is Pillow and I am a Chihuahua!
Example:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class FileScannerClosingExample {
public static void main(String[] args) {
try {
// Creating a Scanner for reading from a file
File file = new File("example.txt");
Scanner fileScanner = new Scanner(file);
// Reading and printing file contents
while (fileScanner.hasNextLine()) {
System.out.println(fileScanner.nextLine());
}
// Attempting to read more from the closed Scanner
fileScanner.nextLine(); // This line will throw an IllegalStateException
// Closing the Scanner to release file resources
fileScanner.close();
} catch (FileNotFoundException e) {
System.err.println("File not found: " + e.getMessage());
} catch (IllegalStateException e) {
System.err.println("IllegalStateException: Scanner is closed.");
}
}
}
In the code example, we start by creating a Scanner
named fileScanner
associated with a file named example.txt
.
Subsequently, we employ a while
loop to iterate through the lines of the file, printing each line to the console. This step illustrates the primary purpose of using a Scanner
for reading file contents.
To emphasize the importance of closing the scanner after its intended use, we intentionally attempt to read more from the closed Scanner
by using nextLine()
. As expected, this results in an IllegalStateException
, underscoring the necessity of proper resource management.
Finally, we invoke the close()
method on the Scanner
to release the file-related resources.
Exception handling is also incorporated, where a FileNotFoundException
is caught if the specified file is not found, and an IllegalStateException
is caught to manage the attempt to read from a closed scanner.
Output:
Hello! My name is Pillow and I am a Chihuahua!
Exception in thread "main" java.util.NoSuchElementException: No line found
at java.base/java.util.Scanner.nextLine(Scanner.java:1651)
at Scanz.main(Scanz.java:19)
When the user runs this program with an existing file, example.txt
, it will successfully read and print the file’s contents line by line. However, attempting to read more from the closed Scanner
will trigger an IllegalStateException
.
Both exceptions are caught and handled, providing informative error messages.
Conclusion
In conclusion, the close()
method is a fundamental practice for ensuring proper resource management when utilizing the Scanner
class in Java. This method plays a pivotal role in preventing potential issues, such as the IllegalStateException
, by releasing associated resources and signaling the end of the scanner’s usage.
By adhering to this best practice, developers enhance the overall stability and reliability of their Java applications.
Closing a Scanner
after reading the contents of a file is a critical practice in Java programming, contributing significantly to effective resource management. This practice ensures the proper release of file-related resources, mitigating potential issues like the IllegalStateException
that may arise when attempting to use a closed scanner.
By incorporating this approach, developers actively contribute to the reliability and efficiency of their Java applications, particularly when handling file input. The close()
method, when appropriately employed, safeguards against resource leaks and enhances the overall robustness of Java programs.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn