How to Get Class Name in Java
- Importance of Getting Class Name in Java
-
Using the
getClass()
Method to Get Class Name in Java -
Using the
getSimpleName()
Method to Get Class Name in Java -
Using the
getName()
Method to Get Class Name in Java -
Using the
getCanonicalName()
Method to Get Class Name in Java - Conclusion
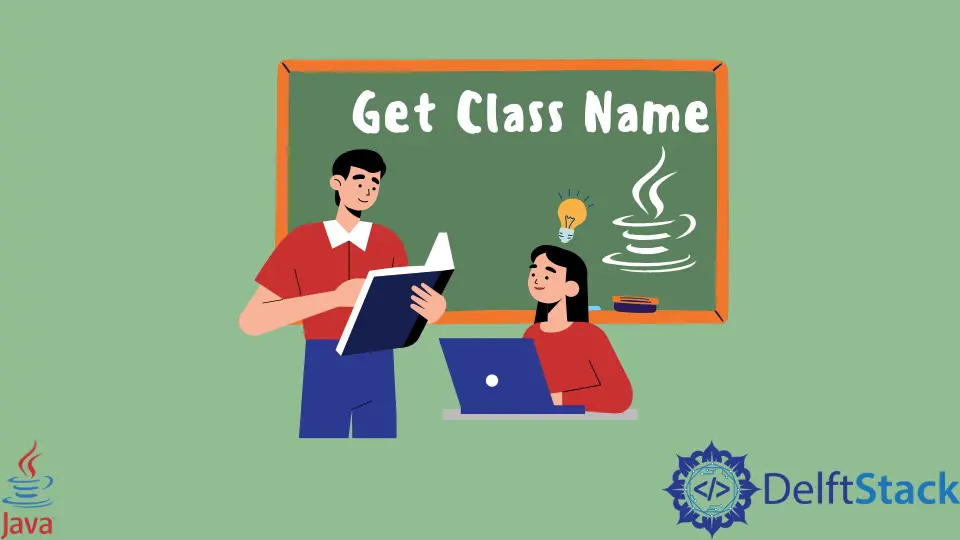
This article explores the significance of obtaining class names in Java and delves into various methods, such as getClass()
, getSimpleName()
, getName()
, and getCanonicalName()
, highlighting their respective importance and applications.
Importance of Getting Class Name in Java
Understanding the class name of an object in Java holds crucial significance in various programming scenarios. Primarily, it facilitates effective debugging and logging practices.
When unexpected issues arise during program execution, logging the class name along with relevant information can significantly streamline the identification and resolution of errors. Additionally, obtaining the class name is pivotal in scenarios involving reflection.
Reflection allows developers to inspect and manipulate the structure and behavior of classes dynamically at runtime. This capability is instrumental in creating generic utilities and frameworks.
Furthermore, class names are essential for creating flexible and reusable code, aiding in the design of systems that can adapt to varying input types or configurations.
Using the getClass()
Method to Get Class Name in Java
The getClass()
method in Java is valuable for obtaining the class name because it provides a dynamic and runtime approach to retrieve class information. getClass()
provides a more versatile means of introspection.
It allows developers to obtain the Class object dynamically, enabling them to explore and utilize additional information beyond just the name, such as annotations, methods, and fields. This flexibility makes getClass()
particularly advantageous in scenarios where a more comprehensive understanding of the class at runtime is needed, contributing to code that is adaptable and capable of handling diverse situations effectively.
Let’s start by examining a simple Java code snippet that demonstrates how to utilize the getClass()
method to obtain the class name of an object:
public class ClassNameExample {
public static void main(String[] args) {
// Creating an instance of MyClass
MyClass myObject = new MyClass();
// Obtaining the Class object using getClass()
Class<?> clazz = myObject.getClass();
// Getting the class name using getName()
String className = clazz.getName();
// Displaying the class name
System.out.println("Class Name: " + className);
}
}
class MyClass {
// Class definition for demonstration purposes
}
In the code example, a class named ClassNameExample
is defined to illustrate the getClass()
method. Inside the main
method, an instance of the class MyClass
is created, named myObject
, which becomes the target for obtaining the class name.
The getClass()
method is then invoked on the myObject
instance, yielding a Class
object denoted as clazz
. This object represents the runtime class of the original object and is of type Class<?>
.
Subsequently, the getName()
method is called on the clazz
object to retrieve the fully qualified class name as a string, and this result is stored in the className
variable. To conclude, the obtained class name is printed to the console using System.out.println()
.
Upon running the code, you will witness the effective use of the getClass()
method in obtaining the class name of the myObject
instance. The output in the console will display:
This output reveals the fully qualified class name, including the package information. Utilizing the getClass()
method provides a dynamic and versatile means of acquiring class names at runtime, offering developers valuable insights into their Java applications.
Using the getSimpleName()
Method to Get Class Name in Java
The getSimpleName()
method in Java is important for obtaining class names because it offers a concise way to retrieve only the class name without the package information. getSimpleName()
is particularly useful when you need a concise representation.
This method enhances code readability and is valuable in scenarios where the full package information is unnecessary. It simplifies the obtained class name, making it more suitable for display, logging, or scenarios where brevity is preferred.
Let’s dive into a practical demonstration of the getSimpleName()
method with a straightforward Java code snippet:
public class SimpleClassNameExample {
public static void main(String[] args) {
// Creating an instance of MyClass
MyClass myObject = new MyClass();
// Obtaining the simple class name using getSimpleName()
String simpleClassName = myObject.getClass().getSimpleName();
// Displaying the simple class name
System.out.println("Simple Class Name: " + simpleClassName);
}
}
class MyClass {
// Class definition for demonstration purposes
}
Our example introduces a class named SimpleClassNameExample
, serving as a context for demonstrating the getSimpleName()
method. Inside the main
method, an instance of MyClass
is created, represented by the variable myObject
, to showcase the magic of the getSimpleName()
method.
The simplicity unfolds when getSimpleClassName()
is invoked on myObject.getClass()
, retrieving the simple name of the runtime class without package details. The obtained simple class name is stored in the variable simpleClassName
.
Upon executing the code, you will observe the simplicity and effectiveness of the getSimpleName()
method in action. The output in the console will display:
This output demonstrates that getSimpleName()
successfully returns the simple name of the class, making it a valuable tool when you need concise class information without the clutter of package details. Embracing the getSimpleName()
method contributes to cleaner and more readable code in scenarios where a simpler representation of class names is desired.
Using the getName()
Method to Get Class Name in Java
The getName()
method in Java is crucial for obtaining class names as it provides a comprehensive and fully qualified representation, including package information. getName()
captures both the class name and its package.
This method is invaluable in scenarios where a detailed understanding of the class, including its package structure, is essential. It is particularly useful in reflection and debugging, offering a holistic view of the class at runtime.
Let’s explore the application of the getName()
method through a practical Java code snippet:
public class FullClassNameExample {
public static void main(String[] args) {
// Creating an instance of MyClass
MyClass myObject = new MyClass();
// Obtaining the full class name using getName()
String fullClassName = myObject.getClass().getName();
// Displaying the full class name
System.out.println("Full Class Name: " + fullClassName);
}
}
class MyClass {
// Class definition for demonstration purposes
}
Our exploration begins with a class named FullClassNameExample
, serving as the foundation for demonstrating the getName()
method. Inside the main
method, an instance of the class MyClass
is created, denoted by the variable myObject
, acting as the focal point for showcasing the getName()
method.
The crux of the process occurs when we invoke getName()
on myObject.getClass()
, retrieving the fully qualified class name that includes both the class name and package information. The obtained full class name is then stored in the variable fullClassName
.
Upon running the code, you will witness the power of the getName()
method in providing a comprehensive representation of the class name. The output in the console will display:
Full Class Name: MyClass
This output encapsulates the fully qualified class name, offering a wealth of information about the class’s package structure and name. The getName()
method proves invaluable in scenarios where a detailed and complete understanding of class names is essential.
Embracing this method enhances the capability of Java developers to create more versatile and insightful applications.
Using the getCanonicalName()
Method to Get Class Name in Java
The getCanonicalName()
method in Java is important for obtaining class names as it provides a standardized and fully qualified representation, including package details. getCanonicalName()
offers a standardized format, making it particularly useful when a uniform, fully qualified class name is required.
This method enhances code clarity and interoperability in scenarios where consistency is crucial, such as when working with external systems or tools.
Let’s delve into the world of getCanonicalName()
with a concise and illustrative Java code snippet:
public class CanonicalClassNameExample {
public static void main(String[] args) {
// Creating an instance of MyClass
MyClass myObject = new MyClass();
// Obtaining the canonical class name using getCanonicalName()
String canonicalClassName = myObject.getClass().getCanonicalName();
// Displaying the canonical class name
System.out.println("Canonical Class Name: " + canonicalClassName);
}
}
class MyClass {
// Class definition for demonstration purposes
}
Our journey begins with a class named CanonicalClassNameExample
, serving as the foundation for exploring the getCanonicalName()
method. Inside the main
method, an instance of the class MyClass
is created, represented by the variable myObject
, which becomes the focal point for showcasing the getCanonicalName()
method.
The essence of our exploration lies in invoking getCanonicalName()
on myObject.getClass()
, retrieving the canonical class name that encapsulates both the class name and the package information. The obtained canonical class name is then stored in the variable canonicalClassName
.
Upon executing the code, you will observe the precision and completeness offered by the getCanonicalName()
method. The output in the console will display:
Canonical Class Name: MyClass
This output provides a clear and canonical representation of the class name, incorporating the package information. The getCanonicalName()
method is particularly useful in scenarios where a standardized, fully qualified class name is required, such as when working with external tools or systems that expect a specific format.
Integrating this method into your Java toolkit enhances your ability to create more robust, interoperable, and well-defined applications.
Conclusion
Understanding and retrieving class names in Java are pivotal for various programming scenarios. Whether for debugging, logging, or dynamic code generation through reflection, the ability to obtain class names provides valuable insights into the runtime behavior of Java applications.
The methods discussed, including getClass()
, getSimpleName()
, getName()
, and getCanonicalName()
, offer distinct advantages based on specific needs. getClass()
provides dynamic runtime access, getSimpleName()
offers a concise name, getName()
delivers a comprehensive fully qualified name, and getCanonicalName()
provides a standardized format.
Choosing the appropriate method depends on the specific requirements of the task at hand, contributing to the flexibility, readability, and adaptability of Java code.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn