How to Compare Strings in Java
-
Compare Strings in Java Using the
compareTo()
Method -
the Equality
==
Operator Method -
the
equals()
Method -
the
equals()
Method Case Sensitivity -
the
contentEquals()
Method
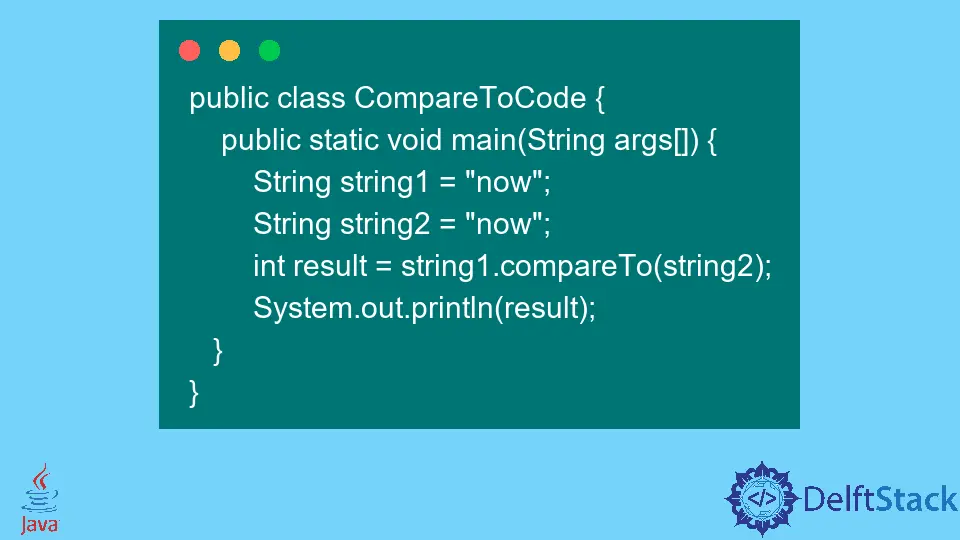
Almost all Java developers will at some point need to compare two strings to complete an application’s logic. A string variable is a collection of characters. To understand the comparison operations that are possible with a string we first need to understand the properties of a string.
- Strings are immutable. It means that they cannot grow and any change to a string leads to a new string being formed. This is because they are internally backed by char-arrays that are immutable.
- Whenever we say a string is created, two objects placed in different memory areas are created. One is created in the heap area, while the second is created in the String constant pool. The string reference points the machine towards the heap area object.
For instance,
String mystring = "World"
In this case, mystring
points to the heap area where the string World
is stored.
Below are the four methods used to compare strings in java and are explained in details in this article:
- The
compareTo()
method - The equality
==
operator - The
equals()
method - The
contentEquals()
method
Compare Strings in Java Using the compareTo()
Method
It compares two strings lexicographically. It works by first assigning each character in the string a Unicode value and then comparing the strings. This process returns the result as zero, a positive number, or a negative number.
- The result is zero when the two compared strings are equal lexicographically.
- The result is positive if the first string is larger than the second string.
- The result is negative when the second string is larger than the first string.
There are two ways of using the compareTo()
method.
Compare Two Strings Literals
Let’s compare string1 and string2. The result we get from comparing these two strings is an int
type. Example:
public class CompareToCode {
public static void main(String args[]) {
String string1 = "now";
String string2 = "now";
int result = string1.compareTo(string2);
System.out.println(result);
}
}
Output:
0
Compare a String and an Object
The compareTo()
method also allows for the comparison of unreferenced objects with a string. Example:
public class CompareTo {
public static void main(String args[]) {
String string1 = "now";
int result = string1.compareTo("now");
System.out.println(result);
}
}
Output:
0
compareTo()
Case Sensitivity
The compareTo()
method is case sensitive. This means that even if two strings are the same, it’ll show them as different if they are not uniform. Example:
public class CompareTo {
public static void main(String args[]) {
String string1 = "hello";
String string2 = "HELLO";
int result = string1.compareTo(string2);
System.out.println(result);
}
}
Output:
-32
The world hello
and HELLO
are handled differently from the above, and thus we get a result of -32.
However, another method in this string class ignores case sensitivity - compareToIgnoreCase()
. Example:
public class CompareToIgnoreCase {
public static void main(String args[]) {
String string1 = "hello";
String string2 = "HELLO";
int result = string1.compareToIgnoreCase(string2);
System.out.println(result);
}
}
Output:
0
the Equality ==
Operator Method
It is a java string comparison method that compares whether two objects are in the same memory location. Example:
public class EqualityOperator {
public static void main(String args[]) {
String oneS = new String("Wow");
String twoS = new String("Wow");
System.out.println(oneS == twoS);
}
}
Output:
false
The ==
operator compares oneS and twoS objects to check whether they are the same. Both Strings, oneS
and twoS
are different references and thus return false. oneS
and twoS
are two different objects. However, they both have the same value in them.
the equals()
Method
This is a method used to compare two strings in java based on their value. The output of this method is either true or false. If the strings being compared are not equal by the value, then the method returns false. If the strings being compared are equal, the method returns true. For instance,
public class Equals {
public static void main(String args[]) {
String oneS = new String("Wow");
String twoS = new String("Wow");
System.out.println(oneS.equals(twoS));
}
}
Output:
true
the equals()
Method Case Sensitivity
The equals()
method is case sensitive and thus sees the same words capped differently as different words. For instance:
public class Equals {
public static void main(String args[]) {
String oneS = new String("Wow");
String twoS = new String("WOW");
System.out.println(oneS.equals(twoS));
}
}
Output:
false
In the above case, wow
compared to WOW
using the equals()
method returns false.
However, if you want to use the equals()
method and not consider case sensitivity, you use the equalsToIgnoreCase()
method. For the above example, equalsIgnoreCase()
would return true.
public class EqualsIgnorecase {
public static void main(String args[]) {
String oneS = new String("Wow");
String twoS = new String("WOW");
System.out.println(oneS.equalsIgnoreCase(twoS));
}
}
Output:
true
the contentEquals()
Method
This is also a java string comparison method that compares a string buffer and string and returns either false
or true
. If the string matches the string buffer that it’s being compared to, the method returns true
. If the string doesn’t match the string buffer it is being compared to, the method returns false
.
Example:
public class ContentEquals {
public static void main(String args[]) {
String firstS = new String("Here we are");
StringBuffer secondS = new StringBuffer("Here we are");
System.out.println(firstS.contentEquals(secondS));
}
}
Output:
true