How to Set Classpath in Java
- How to Set the Classpath in Java?
- Setting Classpath in Java
- Setting Classpath Using Environment Variables
-
Setting
classpath
From Command Line -
Setting
Classpath
Using the-classpath
Option - Viewing the Classpath
- Summary
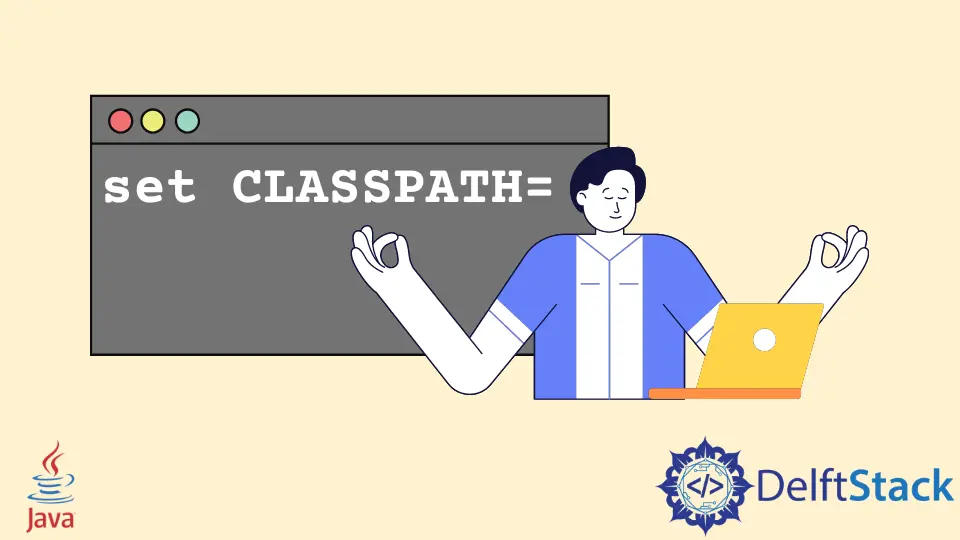
This tutorial introduces the procedure to set classpath in Java and lists some example codes to understand the topic.
How to Set the Classpath in Java?
We will often import external or user-defined classes to make them available for use in our current class. The import statement is used to do this in Java.
import org.projects.DemoClass;
DemoClass dc = new DemoClass();
It would be impossible for the JVM to go through every directory on our system and find the appropriate class. JVM uses the classpath to locate the required classes and jars. In this tutorial, we will learn how to set the classpath in Java.
Setting Classpath in Java
- We can set the classpath as an environment variable. Or we can use the command-line to do this.
- Before we begin, we need to know that the default value of the classpath is the current directory. It is set by using a dot(
.
). Changing the classpath will alter this default value. If you have classes or jars present in the current directory, then include the current directory in the classpath. - We can specify several class paths by using separators. If you are using Windows OS, then the separator is a semicolon(
;
). If you are on Linux/Unix-based systems, the colon(:
) is used as a separator.
The code below demonstrates this. We are trying to include all classes of the current directory(using dot), and two JAR files.
.;C:\javaProjects\someJAR.jar;C:\javaOldProjects\someOldJAR.jar //For Windows
.:/javaProjects/someJAR.jar:/javaOldProjects/someOldJAR.jar //For Linux/Unix
- Classpaths can contain the path to the jar files and the path to the top package of our classes. For example, if the path of the
DemoClass.class
file isC:\javaProjects\org\projects\DemoClass.class
, then we can set the classpath toC:\javaProjects
and use the following import statement.
import org.projects.DemoClass;
Setting Classpath Using Environment Variables
It is a good idea to add a classpath to the environment variable if we have a fixed location that will always contain the required jars and the class files. We need to find the system’s environment variables and add the CLASSPATH variable if absent. Next, we will add all the paths of the jars and the classes.
On a Windows system,
- Click on the Computer icon, and open the properties from the top left corner.
- Click on Advanced System Properties > Advanced > Environment Variables.
- Find the
CLASSPATH
variable and add the paths to it. If theCLASSPATH
variable is absent, then we first need to add the variable.
Setting classpath
From Command Line
We can use the set CLASSPATH
command in Windows to set the classpath. We need to separate different paths by using the semi-colon in Windows. In the example below, we are adding the current directory and a JAR file to the classpath.
$ set CLASSPATH=.;C:\javaProjects\someJAR.jar
In Linux/Unix, we can use the export CLASSPATH
command to set the classpath. Use the colon as a separator for multiple paths.
$ export CLASSPATH=.:/javaProjects/someJAR.jar
Setting Classpath
Using the -classpath
Option
We can use the -classpath
or the -cp
option to set the classpath when compiling and running the class files. The code below demonstrates this.
$ javac -classpath .;C:\javaProjects\someJAR.jar SomeClass.java
$ java -classpath .;C:\javaProjects\someJAR.jar SomeClass
Viewing the Classpath
We can verify whether our classpath was successfully set or not by using the following commands.
For Windows:
echo %CLASSPATH%
For Linux/Unix Based Systems:
echo $CLASSPATH
Summary
The classpath is the location where the JVM will look for classes, JAR files, and other resources. Setting the correct classpath makes sure that our Java application runs smoothly. If the classpath is not set correctly, then we may get ClassNotFoundException
or NoClassDefFoundError. We can either set the environment variables or use the command line to set the classpath. Setting global environment variables for classpath is not recommended. It is recommended to use the -cp
or -classpath
option from the command line to specify the classpath. It will make sure that no global configurations are altered.