Class.forName() and Class.forName().newInstance() in Java
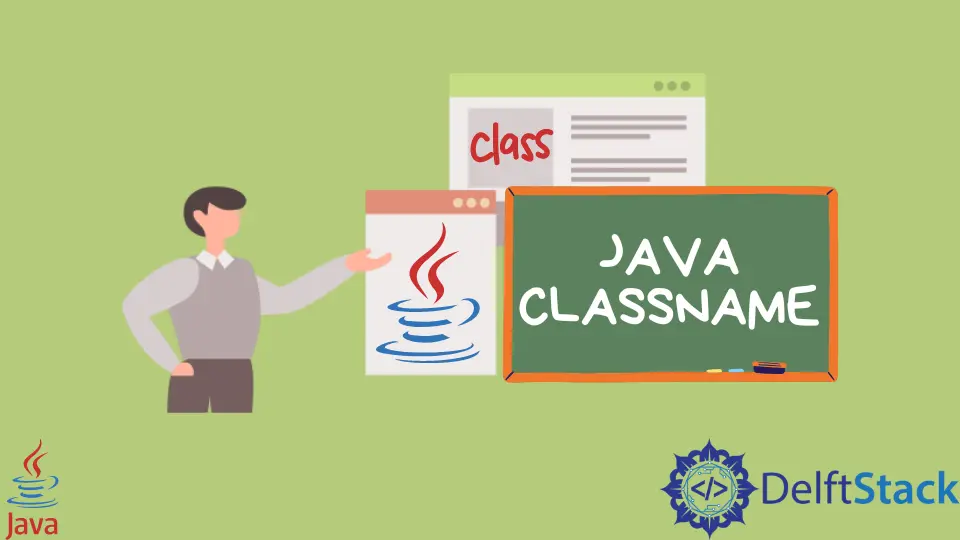
This article will explain the difference between Class.forName()
and Class.forName().newInstance()
in Java.
the Class.forName()
in Java
The Class-Type for the supplied name is returned by Class.forName()
. It will return a reference to a class and load all static blocks, not instance methods, that are accessible.
The java.lang.Class
class’s forName()
function is used to retrieve the instance of such a class with the supplied class name. The class name is supplied as the string
argument.
If the class cannot be found, a ClassNotFoundException
is thrown.
Syntax:
public static Class<S> forName(String suppliedclass) throws ClassNotFoundException
Example:
The forName()
technique is demonstrated in the applications below.
- Create a
Class
type variable namedexampleclass
in theMain
class and set the parameter ofClass.ForName
to ("java.lang.String"
). - Using the following line of code, you may see the name of the
Class
.
System.out.print(" The Example Class represents a class named : " + exampleclass.toString());
Source Code:
public class ForNameExample {
public static void main(String[] args) throws ClassNotFoundException {
Class exampleclass = Class.forName("java.lang.String");
System.out.print("The Example Class represents a class named : " + exampleclass.toString());
}
}
Output:
The Example Class represents a class named : class java.lang.String
the Class.forName().newInstance()
in Java
Class’s instance is returned by newInstance()
. Then that will return an object of that class, which we can use to invoke the instance methods.
The forName()
method of the Class
class, found in the java.lang
package, returns the Class
object for the argument, then loaded by the class loader. The class’s newInstance()
function then returns a new instance.
Whenever you wish to load the class, execute its static blocks, and access its non-static parts, you’ll need an instance, which will require the following.
Syntax:
Class.forName("class").newInstance();
Example:
Create a class called MultiplyExample
and initialize two integer
type variables named z
and x
. This class returns an instance by multiplying two provided numbers.
class MultiplyExample {
int Multiply(int z, int x) {
return (z * x);
}
}
Now create an object of MultiplyExample
named m
, after that apply Class.forName().newInstance()
.
MultiplyExample m = (MultiplyExample) Class.forName("MultiplyExample").newInstance();
At last, display the result by using the passed object to the Multiply
function.
System.out.println("After Multiply result is " + m.Multiply(7, 5));
In this case, Class.forName("MultiplyExample")
generates MultiplyExample.class
, and newInstance()
produces a Multiply
class instance. In short, it’s the same as executing new MultiplyExample()
.
Source Code:
class MultiplyExample {
int Multiply(int z, int x) {
return (z * x);
}
}
class Main {
public static void main(String args[]) throws Exception {
MultiplyExample m = (MultiplyExample) Class.forName("MultiplyExample").newInstance();
System.out.println("After Multiply result is " + m.Multiply(7, 5));
}
}
Output:
After Multiply result is 35
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn