How to Initialize Multiple Variables in Java
- Importance of Initializing Multiple Variables with the Same Value
- Initialize Multiple String Variables With the Same Value in Java
- Conclusion
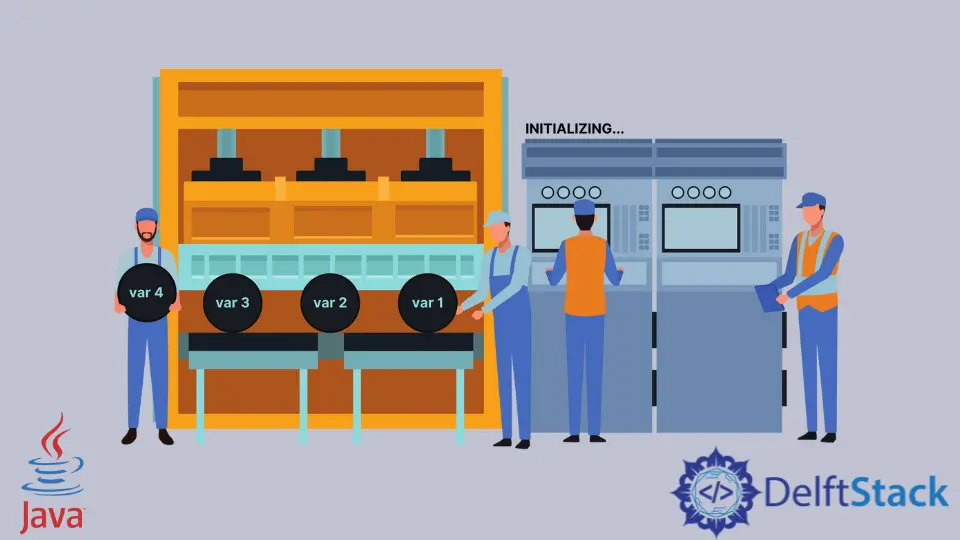
This article delves into the significance of initializing multiple variables with the same value and explores various methods to achieve this essential programming task.
Importance of Initializing Multiple Variables with the Same Value
Initializing multiple variables with the same value is essential for maintaining consistency and ensuring uniformity in a program. It simplifies code maintenance, reduces errors, and enhances readability.
When related variables share a common initial value, it fosters clarity in understanding code logic and promotes efficient use of resources. This practice is particularly crucial in scenarios where a group of variables should start with identical values, ensuring cohesive and predictable behavior in the program.
Initialize Multiple String Variables With the Same Value in Java
Inline Declaration and Initialization
The inline declaration and initialization method is crucial for concise code. Combining variable declaration and assignment in a single line minimizes redundancy and enhances readability.
This approach is efficient and effective, reducing the need for multiple lines and making it advantageous over alternative methods for initializing multiple variables with the same value.
Let’s consider a scenario where we need to initialize three integer variables (a
, b
, and c
) with the same value (let’s say 10
). Instead of repetitive individual assignments, we can leverage inline declaration and initialization to achieve this concisely.
public class InlineInitializationExample {
public static void main(String[] args) {
// Inline declaration and initialization
int a = 10, b = 10, c = 10;
// Displaying the initialized values
System.out.println("Value of a: " + a);
System.out.println("Value of b: " + b);
System.out.println("Value of c: " + c);
}
}
In this code breakdown, we examine the significance of the inline declaration and initialization method.
Initially, three integer variables (a
, b
, and c
) are declared and initialized in a single line, merging the variable type (int
) with the assignment. This streamlined approach reduces code verbosity.
Subsequently, we display the initialized values of a
, b
, and c
on the console, ensuring the successful implementation of the inline declaration and initialization method. This method proves crucial for maintaining code conciseness and verifying accurate variable initialization.
By employing inline declaration and initialization, we achieve a concise and expressive way of initializing multiple variables with the same value. The output of the provided code will be as follows:
This output confirms that all three variables (a
, b
, and c
) have been initialized with the desired value (10
). Utilizing inline declaration and initialization not only enhances code readability but also promotes a more efficient and elegant coding style, making your Java programs more maintainable and less prone to errors.
Chained Assignments Method
The chained assignments method is pivotal for concise and readable code. It reduces redundancy by assigning values in a sequential manner, enhancing the efficiency of variable initialization.
This approach streamlines the process, making the code more compact and maintaining a clear logical flow, providing a distinct advantage over alternative methods.
Consider a scenario where three integer variables (a
, b
, and c
) need to be initialized with the same value, let’s say 15
. The chained assignments method provides a concise and expressive way to achieve this:
public class ChainedAssignmentsExample {
public static void main(String[] args) {
// Chained assignments for initializing multiple variables
int a, b, c;
a = b = c = 15;
// Displaying the initialized values
System.out.println("Value of a: " + a);
System.out.println("Value of b: " + b);
System.out.println("Value of c: " + c);
}
}
Let’s analyze the code to comprehend the intricacies of the chained assignments method.
In the initial step, we declare and assign values to three integer variables (a
, b
, and c
) in a single line, employing chained assignments. The process flows from right to left, with the rightmost value (15
) assigned to c
, followed by the value of c
assigned to b
, and ultimately, the value of b
assigned to a
.
Subsequently, we display the initialized values of a
, b
, and c
on the console, ensuring the successful implementation of the chained assignments method for uniform initialization.
By employing the chained assignments method, we achieve a concise and expressive way of initializing multiple variables with the same value. The output of the provided code will be as follows:
This output confirms that all three variables (a
, b
, and c
) have been initialized with the desired value (15
). The chained assignments method offers an elegant solution to streamline code while maintaining clarity, making it a valuable technique for enhancing the readability and efficiency of your Java programs.
Common Value Method
Initializing multiple variables with the same value using the common value method reduces redundancy and promotes code clarity by providing a single point of reference for initialization. This approach simplifies maintenance and ensures consistency, making code more concise and readable compared to other methods.
Consider a scenario where three integer variables (a
, b
, and c
) need to be initialized with the same value, say 30
. The common value method provides a clean and concise solution:
public class CommonValueInitializationExample {
public static void main(String[] args) {
// Using a common value for initializing multiple variables
int commonValue = 30;
int a = commonValue;
int b = commonValue;
int c = commonValue;
// Displaying the initialized values
System.out.println("Value of a: " + a);
System.out.println("Value of b: " + b);
System.out.println("Value of c: " + c);
}
}
In the code example, we employ the common value method to initialize multiple variables with the same value.
Initially, a variable named commonValue
is declared and assigned the desired value (30
). This single point of reference is then utilized to initialize three integer variables (a
, b
, and c
).
By doing so, we minimize redundancy and enhance code clarity. The initialized values of these variables are subsequently displayed on the console.
This approach, known as the common value method, proves to be a simple and readable technique for achieving uniform initialization in Java.
By employing the common value method, we achieve a simple and effective way of initializing multiple variables with the same value. The output of the provided code will be as follows:
This output confirms that all three variables (a
, b
, and c
) have been successfully initialized with the desired value (30
). The common value method is particularly beneficial when dealing with a small number of variables, offering a clear and concise solution to the task of variable initialization in Java.
Array Method
The array method for initializing multiple variables with the same value is crucial for structured organization and uniformity. It minimizes redundancy, streamlines code, and provides a clear container for related variables.
This approach simplifies maintenance and promotes a concise, readable code structure, making it advantageous over other methods.
Imagine a scenario where you need to initialize three integer variables (a
, b
, and c
) with the same value, say 20
. The array method provides an organized and efficient solution:
public class ArrayInitializationExample {
public static void main(String[] args) {
// Using an array for initializing multiple variables
int[] variables = new int[3];
int commonValue = 20;
// Initializing variables using the common value
for (int i = 0; i < variables.length; i++) {
variables[i] = commonValue;
}
// Displaying the initialized values
System.out.println("Value of a: " + variables[0]);
System.out.println("Value of b: " + variables[1]);
System.out.println("Value of c: " + variables[2]);
}
}
In the code, the array method for initializing multiple variables involves several essential steps.
Initially, an array of integers named variables
is declared, providing a container for the related variables with a length of 3
. Following this, a variable named commonValue
is declared and set to the desired shared value.
The core of the method lies in a for
loop, where each element of the variables
array is iterated, and the commonValue
is assigned, achieving consistent initialization.
The code concludes by displaying the initialized values of the variables (a
, b
, and c
). This method offers a structured approach, enhancing code organization and maintaining uniformity.
By employing the array method, we achieve an organized and efficient way of initializing multiple variables with the same value. The output of the provided code will be as follows:
This output confirms that all three variables (a
, b
, and c
) have been successfully initialized with the desired value (20
). The array method provides a structured approach to handling related variables, contributing to cleaner and more maintainable Java code.
Conclusion
In conclusion, understanding the significance of initializing multiple variables with the same value is fundamental for code consistency and readability. This article has explored various effective methods, including inline declaration, chained assignments, common value, and array usage.
Choosing the right approach depends on the specific context of the program. By embracing these techniques, developers can streamline their code, reduce redundancy, and ensure a cohesive and predictable initialization of variables, contributing to the overall clarity and maintainability of Java programs.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn