在 Java 中初始化多个变量
Rupam Yadav
2023年10月12日
Java
Java Variable
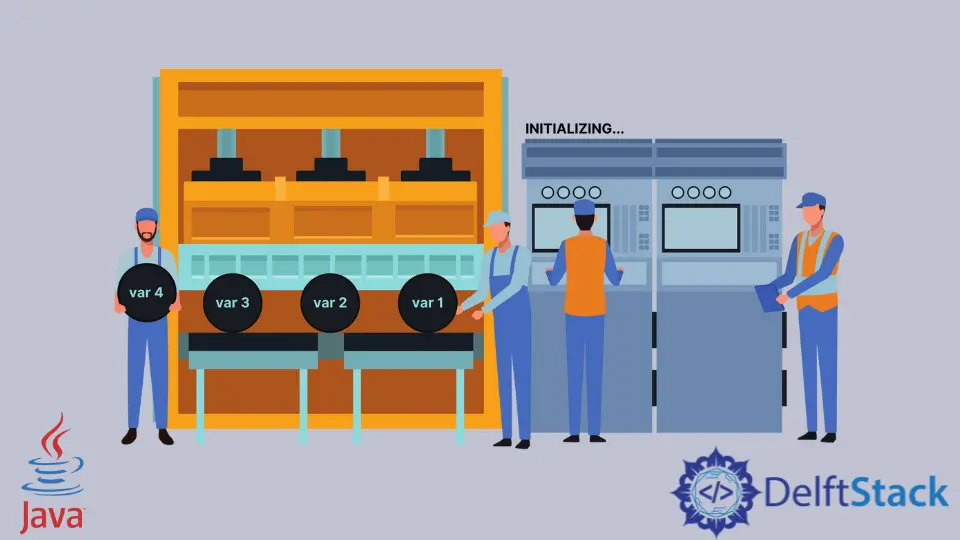
在本文中,我们将介绍在 Java 中,当我们想要初始化多个具有相同值的变量时需要遵循的步骤。我们将讨论为什么我们不能在声明时初始化所有具有相同值的变量。
在 Java 中初始化多个具有相同值的字符串变量
在下面的例子 1 中,我们声明了 String
类型的变量 one
、two
和 three
,然后我们用相同的值初始化这三个变量。我们是通过链式赋值来完成的,这意味着我们将最左边的变量的值赋给赋值操作符右边的所有变量。
- 例子 1
package com.company;
public class Main {
public static void main(String[] args) {
String one, two, three;
one = two = three = "All three variables will be initialized with this string";
System.out.println(one);
System.out.println(two);
System.out.println(three);
}
}
输出:
All three variables will be initialized with this string
All three variables will be initialized with this string
All three variables will be initialized with this string
如果我们的要求是在声明过程中初始化变量,那么我们可以像下面那样使用链式赋值。但是它降低了程序的可读性,因为可能有多个开发人员在同一个项目中工作。
- 例子 2
package com.company;
public class Main {
public static void main(String[] args) {
String one, two, three = two = one = "All three variables will be initialized with this string";
System.out.println(one);
System.out.println(two);
System.out.println(three);
}
}
输出:
All three variables will be initialized with this string
All three variables will be initialized with this string
All three variables will be initialized with this string
在 Java 中用同一个类初始化多个对象
我们看到,我们可以使用链式赋值技术在所有三个 String
变量中存储相同的值。但是,当我们想把同一个类对象的引用保存在多个变量中时,我们是否也可以这样做呢?让我们来看看。
当我们使用 new
关键字用类构造函数初始化一个变量时,这个变量被称为 object
,它指向类。我们可以使用链式赋值来创建同一个类的多个对象,但它将指向同一个引用,这意味着如果我们改变了 firstObj
的值,secondObj
也会反映出同样的变化。
我们可以参考下面的例子,在这个例子中,三个对象-firstObj
、secondObj
和 thirdObj
被分配在一起,但 fourthObj
被分开分配。输出显示了其中的差异。
package com.company;
public class Main {
public static void main(String[] args) {
SecondClass firstObj, secondObj, thirdObj;
firstObj = secondObj = thirdObj = new SecondClass();
firstObj.aVar = "First Object";
secondObj.aVar = "Second Object";
SecondClass fourthObj = new SecondClass();
fourthObj.aVar = "Fourth Object";
System.out.println(firstObj.aVar);
System.out.println(secondObj.aVar);
System.out.println(thirdObj.aVar);
System.out.println(fourthObj.aVar);
}
}
class SecondClass {
String aVar;
}
输出:
Second Object
Second Object
Second Object
Fourth Object
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Rupam Yadav
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn