How to Import Custom Class in Java
- Understanding Java Packages
- Importing a Custom Class in the Same Package
- Importing a Custom Class from a Different Package
- Using Wildcards to Import Multiple Classes
- Static Imports for Class Members
- Conclusion
- FAQ
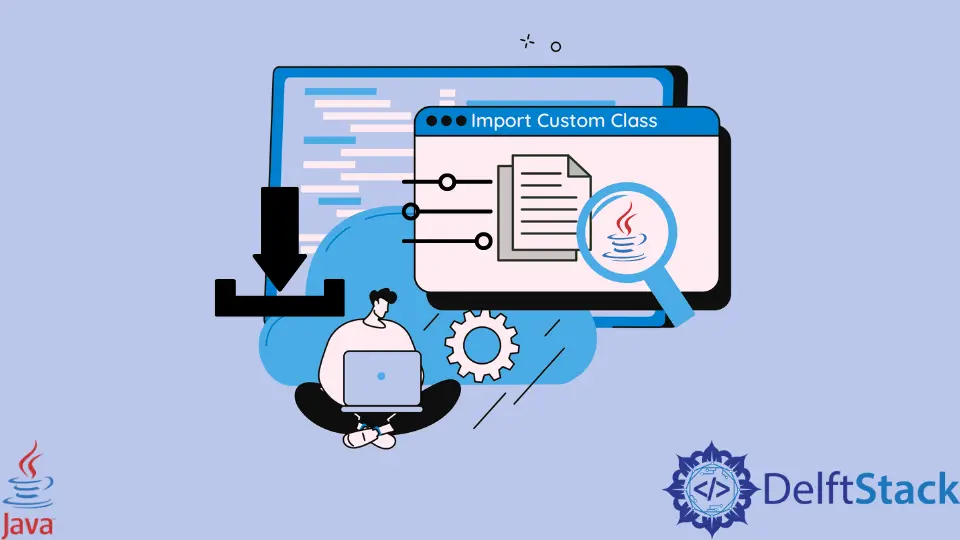
When working with Java, one of the fundamental skills you need to master is importing custom classes. This process allows you to organize your code better and reuse functionalities across different parts of your application. Whether you are developing a small project or a large enterprise application, understanding how to import custom classes can significantly enhance your coding efficiency.
In this article, we will explore various methods to import custom classes in Java, providing clear examples and explanations to help you grasp the concept effectively. Let’s dive in!
Understanding Java Packages
Before we get into the nitty-gritty of importing custom classes, it’s essential to understand Java packages. A package in Java is a namespace that organizes a set of related classes and interfaces. Think of it as a folder in your file system. When you create a custom class, it usually resides within a specific package, and to use that class in another file, you need to import it.
In Java, you can create your own packages using the package
keyword. For instance, if you create a class called MyClass
in a package named com.example
, the declaration at the top of your file would look like this:
package com.example;
public class MyClass {
public void display() {
System.out.println("Hello from MyClass!");
}
}
This simple declaration sets the stage for how we import this class into other files.
Importing a Custom Class in the Same Package
If you have multiple classes within the same package, importing becomes straightforward. You don’t need to use the import
statement at all. You can directly reference the class by its name. Here’s an example:
package com.example;
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.display();
}
}
Output:
Hello from MyClass!
In this example, the Main
class is in the same package as MyClass
. Therefore, you can create an instance of MyClass
without needing to import it explicitly. This is particularly useful for keeping related classes together, making your code cleaner and more manageable.
Importing a Custom Class from a Different Package
When your custom class resides in a different package, you must use the import
statement to access it. This is where things get a bit more interesting. Here’s how you can do it:
Assuming you have MyClass
in com.example
and you want to use it in a class located in com.test
, your setup would look like this:
package com.test;
import com.example.MyClass;
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.display();
}
}
Output:
Hello from MyClass!
In this code snippet, the import com.example.MyClass;
statement allows the Main
class in the com.test
package to access MyClass
. This is crucial for larger projects where classes are organized into different packages. It promotes modularity and reusability, enabling you to maintain your codebase effectively.
Using Wildcards to Import Multiple Classes
If you find yourself needing to import multiple classes from the same package, Java offers a convenient way to do this using wildcards. Instead of importing each class individually, you can import all classes from a package with a single statement. Here’s how:
package com.test;
import com.example.*;
public class Main {
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.display();
}
}
Output:
Hello from MyClass!
The import com.example.*;
statement imports all classes from the com.example
package. This can be a time-saver, especially when dealing with numerous classes. However, be cautious with this approach; it can lead to name conflicts if multiple classes share the same name across different packages.
Static Imports for Class Members
Another powerful feature in Java is static imports. This allows you to import static members (fields and methods) of a class. This can make your code cleaner and easier to read, especially when you use constants. Here’s how you can implement static imports:
Assuming you have a class with static members:
package com.example;
public class MathUtils {
public static final int PI = 3;
public static int square(int number) {
return number * number;
}
}
You can import the static members in another class like this:
package com.test;
import static com.example.MathUtils.PI;
import static com.example.MathUtils.square;
public class Main {
public static void main(String[] args) {
System.out.println("Value of PI: " + PI);
System.out.println("Square of 4: " + square(4));
}
}
Output:
Value of PI: 3
Square of 4: 16
By using import static
, you can access PI
and square
directly without needing to prefix them with the class name. This can make your code cleaner and more intuitive, especially when working with constants or utility methods.
Conclusion
Importing custom classes in Java is a foundational skill that every developer should master. Whether you are working within the same package or across different packages, understanding how to effectively use the import
statement and the various import options available can significantly enhance your coding efficiency. From using wildcards to static imports, these techniques not only help keep your code organized but also promote reusability and maintainability. As you continue your Java journey, remember that effective class management is key to building robust applications.
FAQ
-
What is a package in Java?
A package in Java is a namespace that organizes a set of related classes and interfaces, similar to a folder in a file system. -
Can I import classes from multiple packages in one file?
Yes, you can import classes from multiple packages in a single file using multiple import statements. -
What is the advantage of using wildcards in imports?
Using wildcards allows you to import all classes from a package with a single statement, saving time and reducing clutter in your code. -
What are static imports used for in Java?
Static imports allow you to access static members of a class directly without needing to prefix them with the class name, making your code cleaner. -
Is it possible to import a class without using the import statement?
Yes, if the class is in the same package, you can reference it directly without needing to import it.