How to Round a Double to Two Decimal Places in Java
-
Round
double
to Two Decimal Places in Java UsingMath.round
and Adjusting Scale -
Round
double
to Two Decimal Places in Java UsingBigDecimal
-
Round
double
to Two Decimal Places in Java UsingDecimalFormat
-
Round
double
to Two Decimal Places in Java Using Apache Commons Math -
Round
double
to Two Decimal Places in Java UsingString.format
- Conclusion
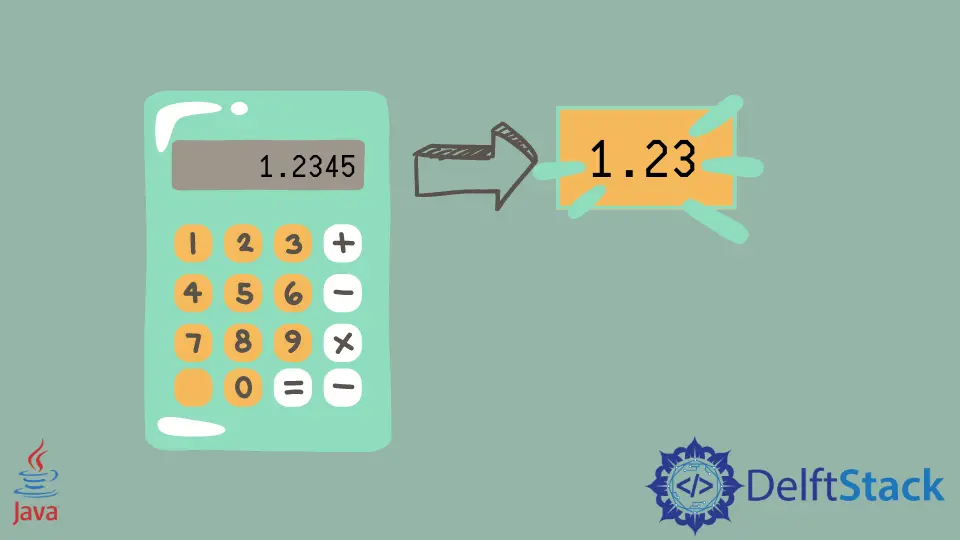
In Java programming, precision and accuracy are paramount when working with numerical data. One common requirement is rounding a double to a specific number of decimal places, a task crucial for financial applications, scientific calculations, or any scenario where exactness matters.
This article delves into various methods available in Java to round a double to two decimal places, exploring approaches such as Math.round
, BigDecimal
, String.format
, and Apache Commons Math. Each method comes with its syntax, advantages, and use cases, providing us with a range of options to meet our specific needs for rounding and maintaining numerical precision.
Round double
to Two Decimal Places in Java Using Math.round
and Adjusting Scale
One common approach to round a double
value to a specific number of decimal places is the Math.round
method in combination with adjusting the scale of the number.
The Math.round
method in Java is designed to round a floating-point number to the nearest integer. To round a double
to two decimal places, we can leverage this method by multiplying the original value by 100, rounding it to the nearest integer, and then dividing it back by 100.
This effectively shifts the decimal point two places to the right before rounding and then back after rounding.
Here’s the syntax:
double roundedNumber = Math.round(originalNumber * 100.0) / 100.0;
In this expression:
originalNumber
is the initialdouble
value you want to round.* 100.0
multiplies the number by 100, shifting the decimal point.Math.round
rounds the result to the nearest integer./ 100.0
then divides the rounded number by 100, restoring the original scale.
Let’s illustrate this approach with a practical example:
public class Main {
public static void main(String[] args) {
double originalNumber = 123.456789;
double roundedNumber = Math.round(originalNumber * 100.0) / 100.0;
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Number: " + roundedNumber);
}
}
In this Java code snippet, firstly, a double
variable named originalNumber
is declared and assigned the value 123.456789
. This represents the initial number that we want to round.
The rounding operation takes place in the subsequent line:
double roundedNumber = Math.round(originalNumber * 100.0) / 100.0;
Breaking this down, the originalNumber
is multiplied by 100.0
. This multiplication effectively shifts the decimal point two places to the right, preparing the number for rounding to two decimal places.
The Math.round
method then rounds this result to the nearest integer. Finally, we divide the rounded number by 100.0
to revert the decimal point to its original position, yielding the desired rounded number.
To illustrate the outcome, the program uses System.out.println
to print the original and rounded numbers to the console:
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Number: " + roundedNumber);
Code Output:
This showcases the result of rounding the original number to two decimal places using the described method. The precision is maintained, and the rounded number is printed for verification or further use in the program.
Round double
to Two Decimal Places in Java Using BigDecimal
Another method for rounding a double
to two decimal places is by using the BigDecimal
class. Unlike the Math.round
method, BigDecimal
provides a more flexible and precise way to handle decimal arithmetic.
The BigDecimal
class in Java allows us to set the scale of a decimal number using the setScale
method. To round a double
to two decimal places, we first create a BigDecimal
object with the original number and then call setScale(2, RoundingMode.HALF_UP)
.
The 2
parameter represents the desired scale (number of decimal places), and RoundingMode.HALF_UP
specifies the rounding method.
Here’s the syntax:
BigDecimal roundedBigDecimal = new BigDecimal(originalNumber).setScale(2, RoundingMode.HALF_UP);
double roundedNumber = roundedBigDecimal.doubleValue();
Where:
originalNumber
is the initialdouble
value you want to round.new BigDecimal(originalNumber)
creates aBigDecimal
object with the original number.setScale(2, RoundingMode.HALF_UP)
sets the scale to two decimal places with rounding.doubleValue()
converts theBigDecimal
back to adouble
.
Let’s illustrate this approach with a different example:
import java.math.BigDecimal;
import java.math.RoundingMode;
public class Main {
public static void main(String[] args) {
double originalNumber = 987.654321;
BigDecimal bd = new BigDecimal(originalNumber).setScale(2, RoundingMode.HALF_UP);
double roundedNumber = bd.doubleValue();
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Number: " + roundedNumber);
}
}
In this Java code snippet, we begin by importing the necessary classes from the java.math
package, namely BigDecimal
and RoundingMode
.
Inside the main
method, a double
variable named originalNumber
is declared and assigned the value 987.654321
. This value represents the initial number that we want to round to two decimal places.
The core rounding operation is performed with the following lines of code:
BigDecimal bd = new BigDecimal(originalNumber).setScale(2, RoundingMode.HALF_UP);
double roundedNumber = bd.doubleValue();
In this sequence:
new BigDecimal(originalNumber)
: This creates a newBigDecimal
object, initialized with theoriginalNumber
.setScale(2, RoundingMode.HALF_UP)
: ThesetScale
method is invoked on theBigDecimal
object to set the scale to two decimal places, andRoundingMode.HALF_UP
is specified as the rounding mode. TheHALF_UP
rounding mode rounds towards the nearest neighbor and breaks ties by rounding up.doubleValue()
: ThedoubleValue
method is called on theBigDecimal
object to convert it back to a primitivedouble
. This is necessary for compatibility with other parts of the code that might expect adouble
data type.
In order to validate the results, the program then prints both the original and the rounded numbers to the console using System.out.println
statements:
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Number: " + roundedNumber);
Code Output:
This demonstrates the successful rounding of the original number to two decimal places using BigDecimal
and provides an accurate representation of the decimal value. The choice of the HALF_UP
rounding mode ensures consistency and precision in the rounding process.
Round double
to Two Decimal Places in Java Using DecimalFormat
The DecimalFormat
class provides another approach for rounding a double
to a specific number of decimal places. This method is particularly useful when you need to format the output for display.
The DecimalFormat
class allows us to specify a custom pattern for formatting decimal numbers. To round a double
to two decimal places, we create a DecimalFormat
object with the desired pattern and then use the format
method to obtain the rounded representation as a string.
The rounded string can later be converted back to a double
if needed.
Here’s the syntax:
double originalNumber = 456.789012;
DecimalFormat df = new DecimalFormat("###.##");
String roundedNumberString = df.format(originalNumber);
double roundedNumber = Double.parseDouble(roundedNumberString);
In this expression:
originalNumber
is the initialdouble
value you want to round.new DecimalFormat("###.##")
creates aDecimalFormat
object with the pattern###.##
, indicating two decimal places.df.format(originalNumber)
formats the original number according to the specified pattern, returning a string.Double.parseDouble(roundedNumberString)
converts the rounded string back to adouble
.
Let’s illustrate this approach with a different example:
import java.text.DecimalFormat;
public class Main {
public static void main(String[] args) {
double originalNumber = 1234.56789;
DecimalFormat df = new DecimalFormat("###.##");
String roundedNumberString = df.format(originalNumber);
double roundedNumber = Double.parseDouble(roundedNumberString);
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Number: " + roundedNumber);
}
}
In this Java code, firstly, we import the DecimalFormat
class from the java.text
package. The main method begins by initializing a double variable named originalNumber
with the value 1234.56789
.
Next, we create an instance of the DecimalFormat
class named df
with a specified format pattern ###.##
. This pattern indicates that we want to round the number to two decimal places.
The #
symbol is a placeholder for a digit, and ##
after the decimal point ensures that we have at most two digits after the decimal.
We then use the format
method of the DecimalFormat
instance (df
) to convert the originalNumber
into a formatted string, roundedNumberString
. This string representation now contains the original number rounded to the specified format.
To obtain the rounded number as a double value, we use Double.parseDouble()
to convert the formatted string back to a double, assigning the result to the roundedNumber
variable.
Finally, we print both the original and rounded numbers to the console using System.out.println()
to observe the transformation and see how the original number is displayed with its full precision while the rounded number adheres to the specified format with only two decimal places.
Code Output:
This demonstrates the successful rounding of the original number to two decimal places using DecimalFormat
, providing a user-friendly and formatted output while maintaining numerical precision.
Round double
to Two Decimal Places in Java Using Apache Commons Math
In Java, the Apache Commons Math library provides a reliable and efficient way to round a double
to a specific number of decimal places. This library provides a more extensive set of mathematical functionalities, and we can leverage it to achieve precise rounding.
To use Apache Commons Math for rounding, you first need to include the library in your project. The Precision.round
method is then employed for rounding a double
to the desired number of decimal places.
The method takes two parameters: the number to be rounded and the scale (number of decimal places). By default, the method uses the rounding mode HALF_UP
.
Here’s the syntax:
import org.apache.commons.math3.util.Precision;
double roundedNumber = Precision.round(originalNumber, scale);
In this expression:
originalNumber
is the initialdouble
value you want to round.scale
is the number of decimal places to which you want to round the number.Precision.round(originalNumber, scale)
rounds theoriginalNumber
to the specified number of decimal places.
Before diving into the code, make sure to include the Apache Commons Math library in your project. You can do this by adding the following dependency to your project’s build file (Maven example):
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-math3</artifactId>
<version>3.6.1</version> <!-- Use the latest version available -->
</dependency>
Let’s illustrate this approach with an example:
import org.apache.commons.math3.util.Precision;
public class Main {
public static void main(String[] args) {
double originalNumber = 9876.54321;
double roundedNumber = Precision.round(originalNumber, 2);
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Number: " + roundedNumber);
}
}
In this Java code snippet, we first import the Precision
class from the org.apache.commons.math3.util
package.
The main method begins by initializing a double variable named originalNumber
with the value 9876.54321
. Instead of using DecimalFormat
as in the previous example, here we directly use the Precision.round()
method from the Apache Commons Math library to round the originalNumber
to two decimal places.
The second argument passed to the round
method specifies the number of decimal places to round to.
The result of the rounding operation is stored in the roundedNumber
variable. The precision of this rounded number is now limited to two decimal places.
Finally, we print both the original and rounded numbers to the console using System.out.println()
.
Code Output:
This showcases the successful use of the Apache Commons Math library’s Precision.round
method for rounding the original number to two decimal places, providing a reliable and precise solution for mathematical operations requiring accurate rounding.
Round double
to Two Decimal Places in Java Using String.format
The String.format
method provides a versatile way to format strings, including rounding a double
to a specified number of decimal places. This approach is particularly useful for creating formatted output or strings for display.
To round a double
using String.format
, you can specify a format pattern that includes the desired number of decimal places. The format specifier %.<decimal-places>f
is used, where <decimal-places>
is the number of decimal places you want in the output.
Here’s the syntax:
double roundedNumber = Double.parseDouble(String.format("%.2f", originalNumber));
In this expression:
originalNumber
is the initialdouble
value you want to round.String.format("%.2f", originalNumber)
creates a formatted string with two decimal places.Double.parseDouble(...)
then converts the formatted string back to adouble
.
Let’s illustrate this approach with an example:
public class Main {
public static void main(String[] args) {
double originalNumber = 123.456789;
double roundedNumber = Double.parseDouble(String.format("%.2f", originalNumber));
System.out.println("Original Number: " + originalNumber);
System.out.println("Rounded Number: " + roundedNumber);
}
}
Here, we begin by initializing a double variable named originalNumber
with the value 123.456789
.
To round the originalNumber
to two decimal places, we use the String.format()
method. The format specifier %.2f
is used within the String.format()
method, where %.2f
indicates that we want to format the floating-point number with two decimal places.
This operation effectively rounds the number to the specified precision. The formatted string is then passed to Double.parseDouble()
, converting it back to a double.
The result of this operation, the rounded number, is stored in the roundedNumber
variable. This approach allows us to achieve rounding without the use of additional rounding or formatting classes.
Finally, we print both the original and rounded numbers to the console using System.out.println()
.
Code Output:
This illustrates the successful use of String.format
for rounding the original number to two decimal places, providing a straightforward and concise method for formatting numeric values in Java.
Conclusion
Rounding a double to two decimal places in Java offers several versatile and precise approaches catering to different use cases. Whether you choose the straightforward Math.round
method, the precision-oriented BigDecimal
, the format-driven String.format
, or the specialized Precision.round
method from Apache Commons Math, each method provides a unique solution with its syntax and advantages.
The Math.round
method is simple and widely used, suitable for basic rounding requirements. On the other hand, BigDecimal
offers enhanced precision, making it ideal for scenarios demanding accurate decimal representation.
For formatting purposes, String.format
allows for concise and flexible string manipulation, while Apache Commons Math provides a specialized library with various mathematical functionalities, including precise rounding.
Choosing the right method depends on the specific needs of your application. If simplicity suffices, opt for the basic rounding methods. For more complex mathematical operations or stringent precision requirements, consider using advanced options like BigDecimal
or Apache Commons Math.