How to Pass a Function as a Parameter in Java
-
Use an Instance of an
interface
to Pass a Function as a Parameter in Java -
Use
java.lang.reflect.Method
to Pass a Function as a Parameter in Java
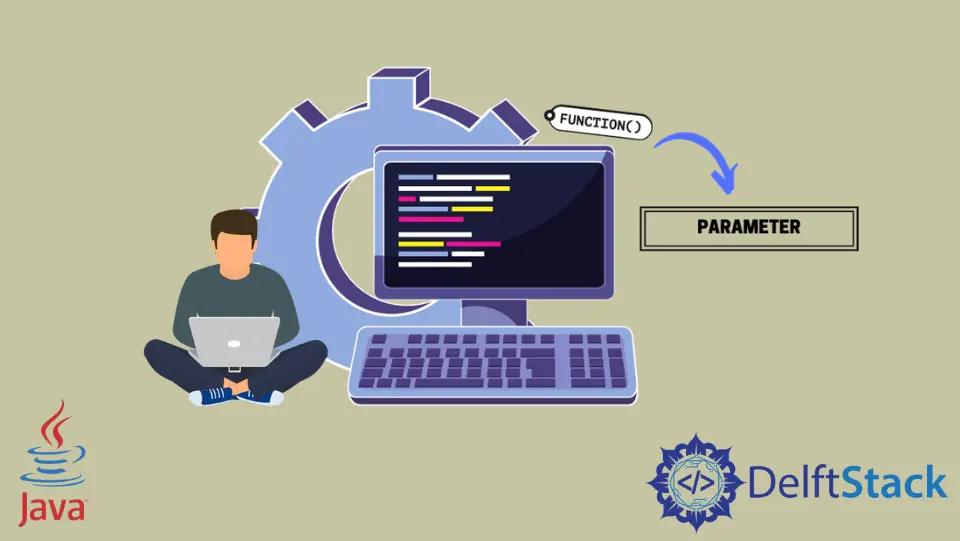
This tutorial will discuss how to pass a function as a parameter to another function in Java.
We will discuss two different methods to pass a function as a parameter in Java.
Use an Instance of an interface
to Pass a Function as a Parameter in Java
In this method, you need to write the function you need to pass as a parameter in a class implementing an interface containing that method’s skeleton only.
The below example illustrates this.
We define an interface Callable
which contains the function skeleton that we plan to pass as a parameter. Next, we define a class that implements Callable
and includes the full definition of the function. This function can be passed to another function like newFunction(Callable callable, int param)
where callable
represents an instance of the interface Callable
.
A full working example is shown in the code below.
interface Callable {
public void call(int param);
}
class Test implements Callable {
public void call(int param) {
System.out.println(param);
}
}
public class HelloWorld {
public static void invoke(Callable callable, int param) {
callable.call(param);
}
public static void main(String[] args) {
Callable cmd = new Test();
invoke(cmd, 10);
}
}
Output:
> 10
Use java.lang.reflect.Method
to Pass a Function as a Parameter in Java
We have a function functionToPass
which we need to pass as a parameter to the function outerFunction
.
There is no difference in how we define functionToPass
; however, we need to follow a specific syntax to define the outerFunction
: outerFunction(Object object, Method method, param1, param2, ...)
.
Have a look at the example below:
import java.lang.reflect.Method;
public class Main {
public void functionToPass(String message) {
String[] split = message.split(" ");
for (int i = 0; i < split.length; i++) System.out.println(split[i]);
}
public void outerFunction(Object object, Method method, String message) throws Exception {
Object[] parameters = new Object[1];
parameters[0] = message;
method.invoke(object, parameters);
}
public static void main(String[] args) throws Exception {
Class[] parameterTypes = new Class[1];
parameterTypes[0] = String.class;
Method functionToPass = Main.class.getMethod("functionToPass", parameterTypes[0]);
Main main = new Main();
main.outerFunction(main, functionToPass, "This is the input");
}
}
Here is another example of the same method. We are creating a function that also has a return value.
import java.lang.reflect.Method;
public class Main {
public int functionToPass(String message) {
return message.length();
}
public void outerFunction(Object object, Method method, String message) throws Exception {
Object[] parameters = new Object[1];
parameters[0] = message;
System.out.println(method.invoke(object, parameters));
}
public static void main(String[] args) throws Exception {
Class[] parameterTypes = new Class[1];
parameterTypes[0] = String.class;
Method functionToPass = Main.class.getMethod("functionToPass", parameterTypes[0]);
Main main = new Main();
main.outerFunction(main, functionToPass, "This is the input");
}
}