How to Join Two Lists in Java
-
Use
Stream
to Join Two Lists in Java -
Use
parameterize constructor
to Join Two Lists in Java -
Use
predeclared new array
to Join Two Lists in Java
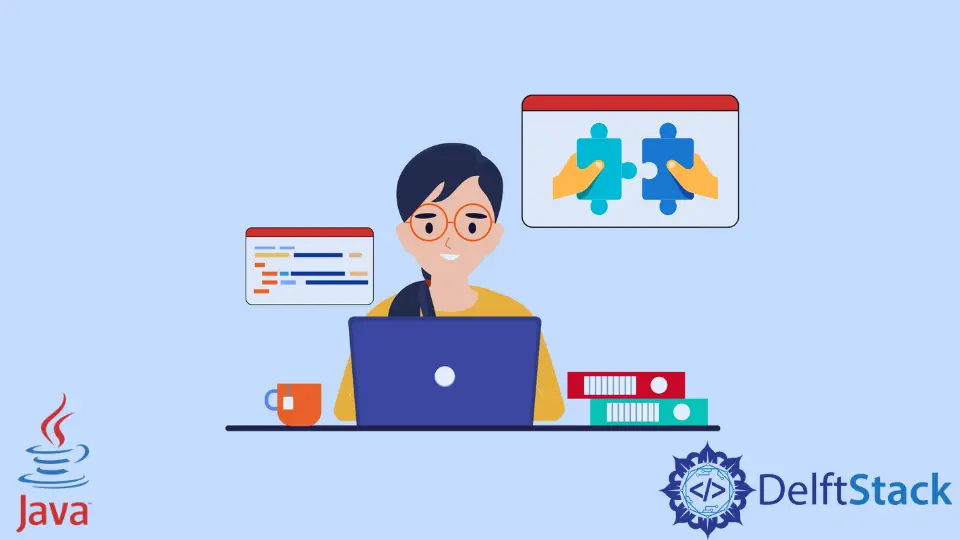
Often, before undertaking any procedure, we have to combine several lists into one. There are quite a few different ways to merge two lists or combine them into larger lists. We will look at two quick ways to merge two lists in Java
In this article, which you can further extend to merge or enforce any number of lists. Array-List or Java-based Linked-List.
One way to combine many collections is to use the java.util collection
class’s addAll()
function, which helps you to add material from one list to another. You can assign contents to as many lists as you want by using the addAll()
function, and it is the easiest way to merge many lists.
Also, some other methods are also explained for better understanding.
- Use of
Stream
- Use of
Parameterized Constructor
- Use of
Predeclared List
andaddAll()
Use Stream
to Join Two Lists in Java
Streams in Java version 8 allow us to have only one-liner solution for the greater part of the issues, and at the same time, the code looks clean. An arrangement of items that underpin different strategies which can be pipelined to create the ideal outcome stream
. In the solution mentioned below, lst.stream()
converts the list into Stream
data type then Stream.concat(Strem1,Stream2)
concatenates two different Stream
objects then Stream.collect(collectors.toList)
converts the Stream
object into the list object.
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Abc {
public static void main(String[] args) {
List<String> list1, list2;
list1 = new ArrayList<String>();
list2 = new ArrayList<String>();
list1.add("1");
list1.add("2");
list1.add("3");
list1.add("4");
list2.add("5");
list2.add("6");
list2.add("7");
list2.add("8");
for (int i = 0; i < list1.size(); i++) {
System.out.print(list1.get(i) + " ");
}
System.out.println();
for (int i = 0; i < list2.size(); i++) {
System.out.print(list2.get(i) + " ");
}
System.out.println();
// first Solution
List<String> resultList1 =
Stream.concat(list1.stream(), list2.stream()).collect(Collectors.toList());
// first Solution Prints
System.out.println("first Solution Prints");
for (int i = 0; i < resultList1.size(); i++) {
System.out.print(resultList1.get(i) + " ");
}
System.out.println();
}
}
Use parameterize constructor
to Join Two Lists in Java
List<String> newList = new ArrayList<String>(listOne);
will create a list by calling the parameterize constructor which copies the given list into the new list object then newList.addAll(listTwo);
appends a list (list2
) into the newly declared list.
import java.util.ArrayList;
import java.util.List;
public class Abc {
public static void main(String[] args) {
List<String> list1, list2;
list1 = new ArrayList<String>();
list2 = new ArrayList<String>();
list1.add("1");
list1.add("2");
list1.add("3");
list1.add("4");
list2.add("5");
list2.add("6");
list2.add("7");
list2.add("8");
for (int i = 0; i < list1.size(); i++) {
System.out.print(list1.get(i) + " ");
}
System.out.println();
for (int i = 0; i < list2.size(); i++) {
System.out.print(list2.get(i) + " ");
}
System.out.println();
// Second Solution
List<String> resultList2 = new ArrayList<String>(list1);
resultList2.addAll(list2);
// Second Solution Prints
System.out.println("Second Solution Prints");
for (int i = 0; i < resultList2.size(); i++) {
System.out.print(resultList2.get(i) + " ");
}
System.out.println();
}
}
Use predeclared new array
to Join Two Lists in Java
When the resultList3
is predefined or it can be done like this List<String> resultList3;
after declaring n result list then newList = new ArrayList<String>(list1)
will initialize new array by coping elements of list1
into it. (list).addAll(list2);
appends the elements of list2
into list1
.
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class Abc {
public static void main(String[] args) {
List<String> list1, list2;
list1 = new ArrayList<String>();
list2 = new ArrayList<String>();
list1.add("1");
list1.add("2");
list1.add("3");
list1.add("4");
list2.add("5");
list2.add("6");
list2.add("7");
list2.add("8");
for (int i = 0; i < list1.size(); i++) {
System.out.print(list1.get(i) + " ");
}
System.out.println();
for (int i = 0; i < list2.size(); i++) {
System.out.print(list2.get(i) + " ");
}
System.out.println();
// third Solution
List<String> resultList3;
(resultList3 = new ArrayList<String>(list1)).addAll(list2);
// Third Solution Prints
System.out.println("Third Solution Prints");
for (int i = 0; i < resultList3.size(); i++) {
System.out.print(resultList3.get(i) + " ");
}
System.out.println();
}
}