How to Get Keys From HashMap in Java
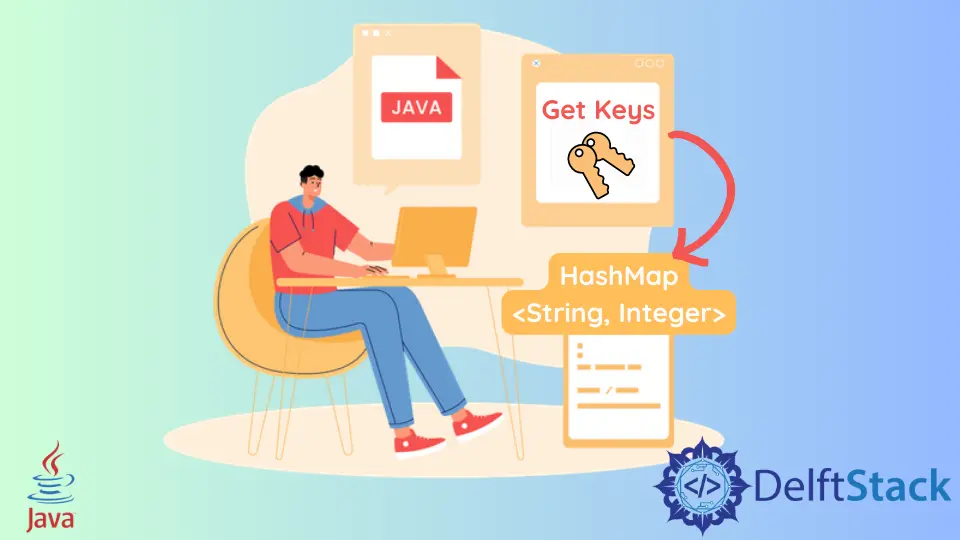
In the world of Java programming, understanding how to manipulate collections is crucial. One of the most widely used collections is the HashMap, which allows you to store key-value pairs. But what if you need to retrieve just the keys from a HashMap? Whether you’re building a complex application or just trying to understand Java better, knowing how to extract keys can be quite beneficial.
In this article, we will explore various methods to get the keys from a HashMap in Java, complete with code examples and detailed explanations. By the end, you’ll be equipped with the knowledge to efficiently access keys in your HashMap.
Using keySet() Method
One of the simplest ways to retrieve keys from a HashMap in Java is by using the keySet()
method. This method returns a Set view of the keys contained in the map. The Set returned is backed by the map, meaning any changes to the map will reflect in the Set and vice versa.
Here’s how you can use the keySet()
method:
import java.util.HashMap;
import java.util.Set;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
Set<String> keys = map.keySet();
for (String key : keys) {
System.out.println(key);
}
}
}
Output:
One
Two
Three
In this example, we create a HashMap and populate it with three key-value pairs. By calling the keySet()
method, we obtain a Set of keys and iterate through it using a for-each loop. This method is efficient and straightforward, making it a popular choice for retrieving keys from a HashMap.
Using Entry Set
Another effective way to retrieve keys from a HashMap is by using the entrySet()
method. This method returns a Set of Map.Entry objects, where each entry contains a key and its corresponding value. While this approach is slightly more complex, it allows you to access both keys and values simultaneously.
Here’s how to implement it:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
for (Map.Entry<String, Integer> entry : map.entrySet()) {
String key = entry.getKey();
System.out.println(key);
}
}
}
Output:
One
Two
Three
In this code, we again create a HashMap and fill it with key-value pairs. The entrySet()
method provides a Set of entries, which we can loop through. Each entry allows us to call getKey()
to retrieve the key. This method is particularly useful when you need to work with both keys and values simultaneously.
Using Streams API
If you’re using Java 8 or later, the Streams API offers a modern and functional approach to retrieve keys from a HashMap. This method is particularly elegant and concise, making it a great choice for developers familiar with functional programming paradigms.
Here’s how to utilize the Streams API:
import java.util.HashMap;
import java.util.List;
import java.util.stream.Collectors;
public class Main {
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
map.put("One", 1);
map.put("Two", 2);
map.put("Three", 3);
List<String> keys = map.keySet().stream().collect(Collectors.toList());
keys.forEach(System.out::println);
}
}
Output:
One
Two
Three
In this example, we first obtain the key set and convert it into a stream. We then collect the keys into a List using Collectors.toList()
. Finally, we print each key using a method reference. This approach is not only concise but also allows for additional stream operations, such as filtering or mapping, making it a powerful tool in modern Java programming.
Conclusion
Retrieving keys from a HashMap in Java is a fundamental skill that every Java developer should master. Whether you choose to use the keySet()
method, the entrySet()
method, or the Streams API, each approach has its own advantages and suits different scenarios. As you grow more comfortable with these methods, you’ll find them invaluable in your programming toolkit. Experiment with these techniques in your projects, and you’ll soon see how they can enhance your efficiency and code quality.
FAQ
-
What is a HashMap in Java?
A HashMap is a part of the Java Collections Framework that stores data in key-value pairs, allowing for fast retrieval based on keys. -
Can a HashMap have duplicate keys?
No, a HashMap cannot have duplicate keys. If you try to insert a duplicate key, the new value will overwrite the existing value.
-
Is HashMap thread-safe?
No, HashMap is not synchronized and is not thread-safe. For thread-safe operations, consider using ConcurrentHashMap. -
How do I check if a key exists in a HashMap?
You can use thecontainsKey(Object key)
method to check if a specific key exists in the HashMap. -
Can I store null values in a HashMap?
Yes, HashMap allows one null key and multiple null values.