How to Exit a Method in Java
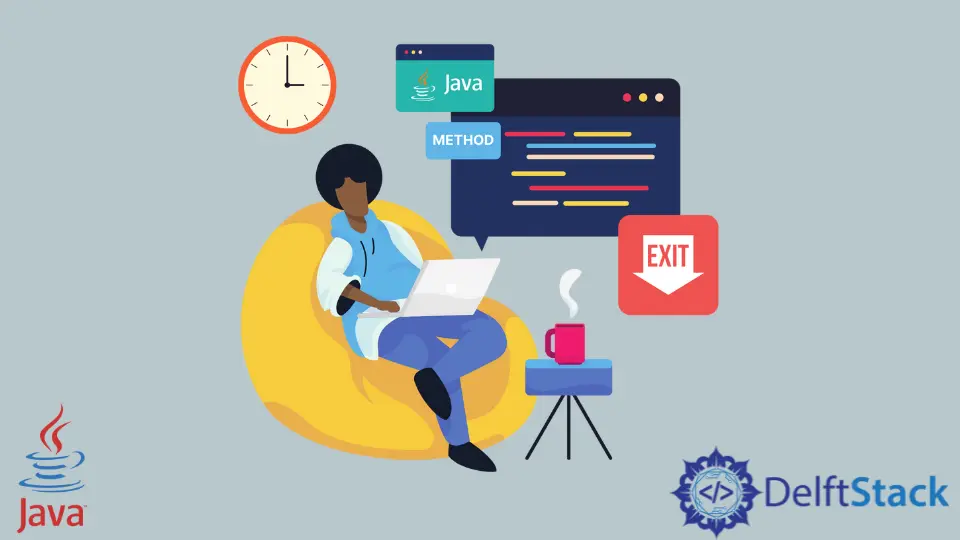
In this tutorial, we will uncover the techniques to exit or get out of a method in Java. Sometimes, there is a requirement to stop the current running function and get out of it. Commonly it happens when a condition is met. We will check out two ways to how to exit a method in Java.
Exit a Java Method Using System.exit(0)
System.exit(0)
is a method that, when executed, terminates the Java Virtual Machine (JVM), which results in termination of the currently running program too. It takes a single argument that is the status. If the status is 0, it indicates that the termination is successful, while a non-zero status tells an unsuccessful termination. We use this method to exit a method in the following example.
We have a method exampleMethod()
that takes an array of int
type. Our goal is to exit the exampleMethod()
when the index becomes more than 3. To do this, we loop through the array and insert a condition that, if met, will execute System.exit(0)
that will terminate the JVM, and thus the method will be exited.
public class ExitMethod {
public static void main(String[] args) {
int[] array = {0, 2, 4, 6, 8, 10};
exampleMethod(array);
}
public static void exampleMethod(int[] array) {
for (int i = 0; i < array.length; i++) {
if (i > 3) {
System.exit(0);
}
System.out.println("Array Index: " + i);
}
}
}
Output:
Array Index: 0
Array Index: 1
Array Index: 2
Array Index: 3
Exit a Java Method Using return
Another way to exit a method is by using the return
keyword. This keyword completes the execution of the method when used and can also be used to return a value from the function. In the below example, we have a method, exampleMethod
, that takes two parameters: num1
and num2
.
We perform subtraction in the method, but we also check if num2
is greater than num1
and if this condition becomes true, return
will exit the method, and the execution will be completed. As we are using void
as the return type, this method will not return any value.
public class ExitMethod {
public static void main(String[] args) {
exampleMethod(2, 5);
exampleMethod(3, 2);
exampleMethod(100, 20);
exampleMethod(102, 110);
}
public static void exampleMethod(int num1, int num2) {
if (num2 > num1)
return;
int subtractedValue = num1 - num2;
System.out.println(subtractedValue);
}
}
Output:
1
80
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn